By Zevan | September 17, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
[SWF(width = 800, height=600, backgroundColor=0x000000, frameRate = 60)]
-
-
var main:MovieClip = MovieClip(addChild(new MovieClip()));
-
main.z = 500;
-
main.rotationX = -40;
-
-
var sim:QuickBox2D = new QuickBox2D(main);
-
-
sim.createStageWalls({fillColor:0x1133CC});
-
sim.setDefault({lineColor:0xFFFFFF, fillColor:0x113355});
-
-
for (var i:int = 0; i<30; i++){
-
var b:QuickObject = sim.addBox({x:Math.random()*10 + 3, y:Math.random()*10 + 3,
-
width:0.25 + Math.random()*2, height:0.25 + Math.random()*2});
-
}
-
-
sim.start();
-
sim.mouseDrag();
This demo shows how you can render your QuickBox2D simulation to somewhere other than the main timeline. In this case, I render to a MovieClip that has altered z and and rotationX properties.
Have a look at the swf...
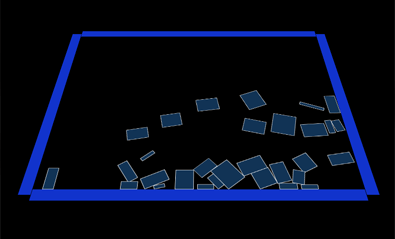
By Zevan | September 16, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Common.Math.*;
-
-
[SWF(width = 800, height = 600, backgroundColor = 0x222222, frameRate=60)]
-
-
var sim:QuickBox2D = new QuickBox2D(this, {debug:false});
-
-
sim.createStageWalls();
-
-
var cup:QuickObject = sim.addPoly({x:3, y:13, wireframe:false, density:0,
-
points:[0,0, .5, 0, .5,3, 2,3, 2,0, 2.5,0, 2.5, 3.5, 0, 3.5, 0,0]});
-
var deleter:QuickObject = sim.addBox({x:4.251, y: 15.9, width:1.5, height:0.25, fillColor:0xFF0000});
-
-
// use a distance joint instead of a group since isCurrentContact
-
// uses the QuickObject.body property
-
sim.addJoint({a:cup.body, b:deleter.body, x1:deleter.x, y1:deleter.y+0.5});
-
-
var circles:Array = [];
-
for (var i:int = 0; i<10; i++){
-
circles[i] = sim.addCircle({x:8 + i, y:5, radius:0.5});
-
}
-
var boxes:Array = [];
-
for (i = 0; i<10; i++){
-
boxes[i] = sim.addBox({x:8.5 + i, y:10, width:0.5, height:0.5});
-
}
-
-
sim.start();
-
sim.mouseDrag();
-
-
var shootVec:b2Vec2 = new b2Vec2(4, -30);
-
-
// when circles are dropped in the cup they are destroyed
-
// when boxes are droppped in the cup their linear velocity is altered
-
var contacts:QuickContacts = sim.addContactListener();
-
contacts.addEventListener(QuickContacts.ADD, onAdd);
-
function onAdd(evt:Event):void{
-
for (var i:int = 0; i<circles.length; i++){
-
var circ:QuickObject = circles[i];
-
if (contacts.isCurrentContact(circ, deleter)){
-
// it's ok to destroy QuickObject here because they are only actually
-
// destroyed after the timeStep has finished at the end of QuickBox2D's
-
// internal loop
-
circ.destroy();
-
}
-
}
-
for (i = 0; i<boxes.length; i++){
-
var box:QuickObject = boxes[i];
-
if (contacts.isCurrentContact(box, deleter)){
-
box.body.SetLinearVelocity(shootVec);
-
}
-
}
-
}
Note: This snippet requires QuickBox2D 1.0 or greater
Here is another example of using QuickBox2D style contacts. In this example there is a cup with a small red box inside of it. When circles are placed inside the cup they are destroyed. When boxes are placed inside the cup they are shot upward.
Have a look at the swf...
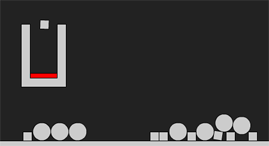
A Note About Creation and Destruction
It's worth noting that in Box2D your not supposed to destroy or create rigid bodies within the listener function for any contact events. QuickBox2D gets around this restriction by flagging the rigid body for destruction and destroying it at the end of QuickBox2D's internal loop. This is not the case with creation of rigid bodies - if you attempt to create a rigid body within one of these listener functions you will get an error. See QuickBox2D Contacts Part 1 for an example of creating rigid bodies when there is a collision.
By Zevan | September 15, 2009
Here is a quick list of the new features in QuickBox2D along with links to the docs.
1) The QuickBox2D.STEP event:
A few releases ago, frim (frame rate independent motion) was added to QuickBox2D. However, there was no way to execute calls to b2Body.ApplyImpulse() b2Body.SetLinearVelocity() etc... along with the b2World.Step() method. This meant that you couldn't call these methods in a way that would truly maintain frim. As you'll see in upcoming demos, rather than doing key controls or additional simulation logic in an enterframe event, I'll be listening for the QuickBox2D.STEP event. This is executed every time a timeStep occurs. If frim is on, this the STEP event will be executed independently of frameRate.
2) Some features were added to help trigger events at specific times in the simulation. See:
QuickBox2D.totalTimeSteps
QuickBox2D.addTimeStepSequence
...for an example of addTimeStepSequence() check out this recent post.
3) Some additional control was added to mouse dragging for QuickBox2D. There is a new property in the QuickBox2D params object called customMouse. When set to true, this enables you to pass in mouse coordinates rather than having QuickBox2D automatically use the coordinates of the DisplayObject that all the rigid bodies are being drawn to. This feature is useful if you are rendering using a 3D engine. See:
QuickBox2D customMouse
QuickBox2D.setMouse()
4) A few features were added to make contact points easy to work with. See:
QuickBox2D.addContactListener()
QuickContacts
...I'll be posting a few demos showing how to use QuickContacts - here are the first two (more to come in the next few days):
part 1
part 2
By Zevan | September 15, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
-
[SWF(width = 800, height = 600, backgroundColor = 0x000000, frameRate=60)]
-
-
var sim:QuickBox2D = new QuickBox2D(this);
-
-
sim.createStageWalls();
-
-
var boxA:QuickObject = sim.addBox({x:3, y:3, width:2, height:2});
-
var boxB:QuickObject = sim.addBox({x:3, y:6, width:2, height:2});
-
-
// add a few extra circles to show that there are other contacts
-
// occuring
-
for (var i:int = 0; i<10; i++){
-
sim.addCircle({x:5 + i, y:4, radius:0.1 + Math.random()});
-
}
-
-
sim.start();
-
sim.mouseDrag();
-
-
// when boxA touches boxB change their alpha values to 50%
-
var contacts:QuickContacts = sim.addContactListener();
-
contacts.addEventListener(QuickContacts.ADD, onAdd);
-
contacts.addEventListener(QuickContacts.PERSIST, onPersist);
-
contacts.addEventListener(QuickContacts.REMOVE, onRemove);
-
function onAdd(evt:Event):void{
-
if(contacts.isCurrentContact(boxA, boxB)){
-
boxA.userData.alpha = 0.5;
-
boxB.userData.alpha = 0.5;
-
}
-
}
-
function onPersist(evt:Event):void{
-
if(contacts.isCurrentContact(boxA, boxB)){
-
boxA.userData.alpha = 0.5;
-
boxB.userData.alpha = 0.5;
-
}
-
}
-
function onRemove(evt:Event):void{
-
if(contacts.isCurrentContact(boxA, boxB)){
-
boxA.userData.alpha = 1;
-
boxB.userData.alpha = 1;
-
}
-
}
Note: This snippet requires QuickBox2D 1.0 or greater
This is another example showing how to make use of QuickBox2D contacts. In this example three contact events are used ADD, PERSIST and REMOVE. When the two boxes are touching their alpha values are set to 50% - otherwise their alpha values are at 100%.
Have a look at the swf...
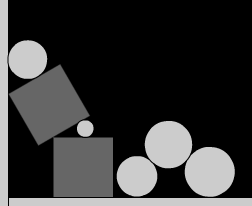
A Little Detail About the Events
The ADD event is dispatched when a new b2ContactPoint is generated. The PERSIST event is dispatched as long as the b2ContactPoint exists for more than one timeStep - and from what I can tell, is dispatched until the rigid bodies it is associated with go to sleep. The REMOVE event is called when the b2ContactPoint is removed, meaning the rigid bodies that caused the creating of the b2ContactPoint are no longer touching.
You need to be careful with the code that you place inside the listener functions for these events. The reason being that these events are called many many times for every contact point in the simulation.