Actionscript:
-
var rand:Number = Math.random() * Math.random() * Math.random();
This is a trick I use when I need more contrast in my random numbers. In this case, the variable rand will get closer to the number 1 significantly less frequently than if you just used Math.random() once.
To illustrate this I created this snippet:
Actionscript:
-
[SWF(width=800, height=600)]
-
-
var r:Number = Math.random() * Math.random() * Math.random();
-
var inc:int = 0;
-
var xp:Number = 10;
-
var yp:Number = 10;
-
var count:int = 1;
-
-
var compare:Shape = Shape(addChild(new Shape()));
-
compare.graphics.lineStyle(0,0x2222FF);
-
-
graphics.lineStyle(0,0x00000);
-
scaleX = scaleY = 2;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
r = Math.random() * Math.random() * Math.random();
-
-
if (inc == 0){
-
graphics.moveTo(xp + inc, yp + 30 - r * 30);
-
}else{
-
graphics.lineTo(xp + inc, yp + 30 - r * 30);
-
}
-
-
r = Math.random();
-
if (inc == 0){
-
compare.graphics.moveTo(xp + inc, yp + 70 - r * 30);
-
}else{
-
compare.graphics.lineTo(xp + inc, yp + 70 - r * 30);
-
}
-
inc++;
-
if (inc == 50){
-
inc = 0;
-
xp = 10 + count % 6 * 60;
-
yp = 10 + int(count / 6) * 80;
-
r = Math.random()*Math.random();
-
count++;
-
}
-
}
The blue lines plots normal Math.random() and the black lines plots Math.random()*Math.random()*Math.random()
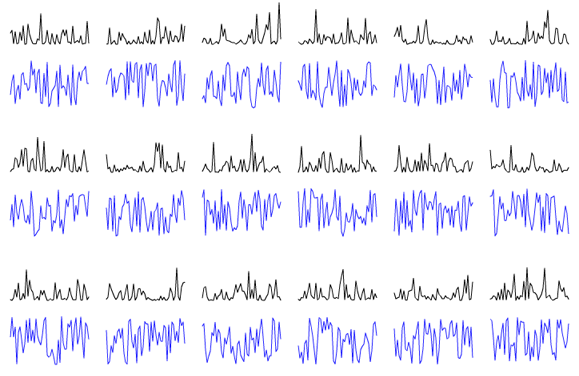
Also posted in random | Tagged actionscript, as3, flash |
Actionscript:
-
for (var i:int = 0; i<10; i++){
-
// randomly set a variable to -1 or positive 1
-
trace(int(Math.random()*2) - 1 | 1);
-
}
-
-
/* outputs something like this
-
1
-
1
-
-1
-
1
-
-1
-
-1
-
1
-
1
-
-1
-
-1
-
*/
The past few days I found myself using this one-liner a few times. Just a quick way to randomly get -1 or 1.
I was brainstorming today and wrote a weird/bad inline version of an old post:
var rand:Number = [-3, 2, 1, 1, 1, 0.5][int(Math.random() * 6)];
I need to install a related posts plugin for WordPress - would be nice if this post were related to my old post about Tausworthe random seeds.
Been posting medium/large snippets and snippets the relate to QuickBox2D - so today I figured I'd get back to basics...
Also posted in random | Tagged actionscript, as3, flash |
Actionscript:
-
var leng:int = 10;
-
for (var i:int = 0, j:int = leng; i <leng; i++, j = leng - i){
-
trace(i, j);
-
}
-
-
/*outputs
-
0 10
-
1 9
-
2 8
-
3 7
-
4 6
-
5 5
-
6 4
-
7 3
-
8 2
-
9 1
-
*/
Looping backwards and forwards.
Also posted in Math, misc | Tagged actionsnippet, flash |
Actionscript:
-
function create(obj:Class, props:Object):*{
-
var o:* = new obj();
-
for (var p:String in props){
-
o[p] = props[p];
-
}
-
return o;
-
}
-
-
// test out the function
-
-
var txt:TextField = create(TextField, {x:200, y:100, selectable:false, text:"hello there", textColor:0xFF0000, defaultTextFormat:new TextFormat("_sans", 20)});
-
addChild(txt);
-
-
var s:Sprite = Sprite(addChild(create(Sprite, {x:100, y:100, rotation:45, alpha:.5})));
-
-
with (s.graphics) beginFill(0xFF0000), drawRect(-20,-20,40,40);
-
-
var blur:BlurFilter = create(BlurFilter, {blurX:2, blurY:8, quality:1});
-
-
s.filters = [blur];
This snippet shows a function called create() that takes two arguments. The first argument is the name of a class to instantiate. The second is an Object with a list of properties to set on a newly created instance of the class (referenced in the first argument).
This could be particularly useful for TextFields which for some reason have no arguments in their constructor.
This will currently only work for classes that have either all optional constructor arguments or no constructor arguments.