Actionscript:
-
[SWF(width = 600, height=600, backgroundColor=0xCCCCCC, frameRate=24)]
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight, false, 0x000000);
-
var blur:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight, false, 0x000000);
-
addChild(new Bitmap(canvas, "auto", true));
-
-
var w:int = canvas.width
-
var hw:int = w / 2;
-
var size:int = w * w;
-
var seed:Number = Math.random()*100;
-
var pnt:Point = new Point();
-
var dy:Number = 0, dx:Number = 0;
-
var blr:BlurFilter = new BlurFilter(10,10,1);
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
dx += (mouseX - dx) / 4;
-
dy += (mouseY - dy) / 4;
-
canvas.lock();
-
canvas.perlinNoise(hw,hw,2,seed,false, false, 1, true, [new Point(dx, dy), new Point(-dx, -dy)]);
-
var pix:Vector.<uint> = canvas.getVector(canvas.rect);
-
for (var i:int = 0; i<size; i++){
-
var col:uint = 255 - pix[i] <<4 & 0x00FF00;
-
pix[i] = col <<8 | col | col>> 8;
-
}
-
canvas.setVector(canvas.rect, pix);
-
blur.copyPixels(canvas, canvas.rect, pnt);
-
blur.applyFilter(blur, blur.rect, pnt, blr);
-
canvas.draw(blur, null, null, BlendMode.DIFFERENCE);
-
canvas.draw(canvas, null, null, BlendMode.INVERT);
-
canvas.unlock();
-
}
This is actually an optimized variation on some recent posts that made use of perlin noise. You can get a wide range of effects by changing just the BlendMode values alone.... I particularly like this combination of BlendModes because it reminds me a bit of a terrain map...
Have a look at the swf...
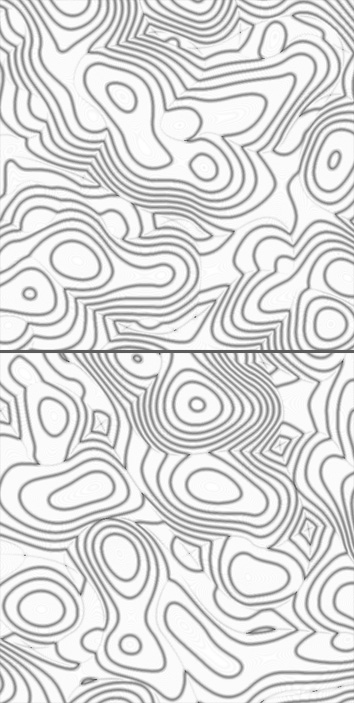
Actionscript:
-
[SWF(backgroundColor=0x000000, width = 800, height = 600)]
-
-
// this is a trick to keep the 3D texture quality up...
-
// try setting it right off the bat and you'll notice that the
-
// Shapes look pixilated
-
setTimeout(function():void{ stage.quality="low"}, 500);
-
-
var matrix:Matrix = new Matrix();
-
matrix.createGradientBox(600, 600, 0, -450, -450);
-
-
var boxNum:int = 30;
-
var boxes:Array = [];
-
for (var i:int = 0; i<boxNum; i++) boxes[i] = makeBox();
-
-
var dx:Number = 0, dy:Number = 0;
-
onLoop();
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function onLoop(evt:Event=null):void {
-
dx += (mouseX - dx) / 4;
-
dy += (mouseY - dy) / 4;
-
for (var i:int = 0; i<boxNum; i++){
-
var box:Shape = boxes[i];
-
box.z = 400 - i * 20;
-
box.x = dx;
-
box.y = dy;
-
box.rotation = i + getTimer() / 10;
-
}
-
}
-
-
function makeBox():Shape{
-
var box:Shape = Shape(addChild(new Shape()));
-
box.x = stage.stageWidth/2;
-
box.y = stage.stageHeight/2;
-
box.z = 1;
-
with (box.graphics){
-
beginGradientFill(GradientType.RADIAL, [0xFFFFFF, 0x333333], [1,1], [0, 255], matrix, SpreadMethod.PAD);
-
drawRect(-100, -100, 200, 200);
-
drawRect(-70, -70, 140, 140);
-
}
-
return box;
-
}
This snippet draws 30 gradient box shapes, gives them different z values and then moves them based on the mouse. This technique is good if you just want a few layers of parallax motion - I got carried away and you'll notice that if you add more boxes it begins to slow down pretty quick.
Have a look at the swf....
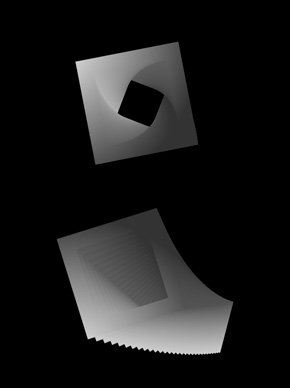
I first used this technique for this small interactive drawing...
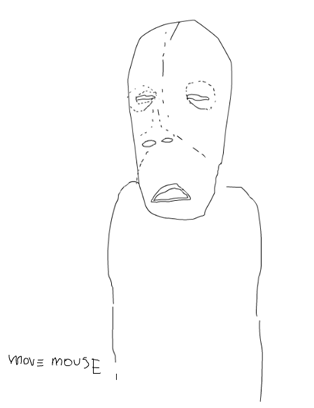
Something interesting I noticed about fp10 3D DisplayObjects is that if you set the stage.quality to low right off the bat, the display objects look pixelated... but if you wait a few milliseconds, you end up with less pixelation and you still get a speed boost from the low quality - I think it must have something to do with the way the 3D textures are handled by the player...
Tomorrow I think I'll post a version of this that uses IGraphicsData and Utils.projectVectors()... should be a huge speed boost...
Posted in 3D, motion | Tagged actionscript, as3, flash |
Actionscript:
-
var canvas:BitmapData = new BitmapData(1200,1200,false, 0x000000);
-
addChild(new Bitmap(canvas));
-
-
scaleX = scaleY = 0.5;
-
var w:int = canvas.width
-
var hw:int = w / 2;
-
var hhw:int = hw / 2;
-
var size:int = canvas.width * canvas.width;
-
-
canvas.perlinNoise(hhw,hhw,1,Math.random()*100,false, false, 1, true);
-
-
for (var i:int = 0; i<size; i++){
-
var xp:int = i % w;
-
var yp:int = int(i / w);
-
var col:uint = canvas.getPixel(xp, yp) / (-20|i+xp)>> 8 & 0xFF
-
canvas.setPixel(xp, yp, col <<16 | col <<8 | col);
-
}
-
-
canvas.applyFilter(canvas, canvas.rect, new Point(0,0), new BlurFilter(4,4,1));
-
var blur:BitmapData = canvas.clone();
-
blur.applyFilter(blur, blur.rect, new Point(0,0), new BlurFilter(10,10,1));
-
-
canvas.draw(blur, null, null, BlendMode.DARKEN);
This is a variation on yesterdays post. I think it's time to optimize this and see how it does in real time...
Here is what this snippet will draw:
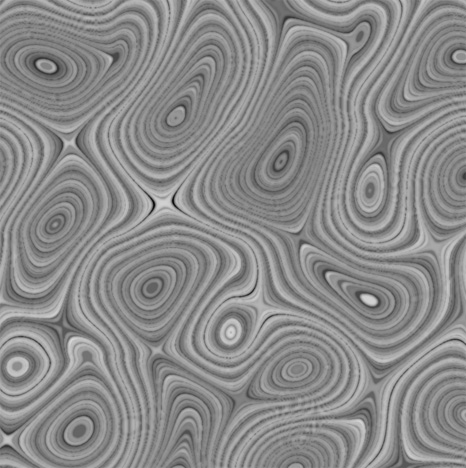
Actionscript:
-
var canvas:BitmapData = new BitmapData(1200,1200,false, 0x000000);
-
addChild(new Bitmap(canvas));
-
-
scaleX = scaleY = 0.5;
-
var w:int = canvas.width
-
var hw:int = w / 2;
-
var hhw:int = hw / 2;
-
var size:int = canvas.width * canvas.width;
-
-
canvas.perlinNoise(hhw,hhw,2,Math.random()*100,false, false, 1, true);
-
-
for (var i:int = 0; i<size; i++){
-
var xp:int = i % w;
-
var yp:int = int(i / w);
-
var col:uint = canvas.getPixel(xp, yp) / (xp+yp-w)>> 8 & 0xFF
-
canvas.setPixel(xp, yp, col <<16 | col <<8 | col)
-
}
-
-
var blur:BitmapData = canvas.clone();
-
blur.applyFilter(blur, blur.rect, new Point(0,0), new BlurFilter(10,10,1));
-
-
canvas.draw(blur, null, null, BlendMode.ADD);
I was playing around awhile back and created this snippet, it will draw something that looks like this:
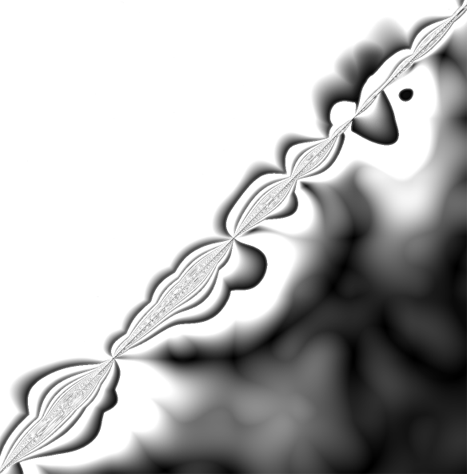
this is one of those snippets that can produce vastly different looking images with minor changes to the code... for instance, try changing the blendMode to darken and line 15 to this:
var col:uint = canvas.getPixel(xp, yp) / (xp|yp-w) >> 5 & 0xFF;
and you'll end up with this:
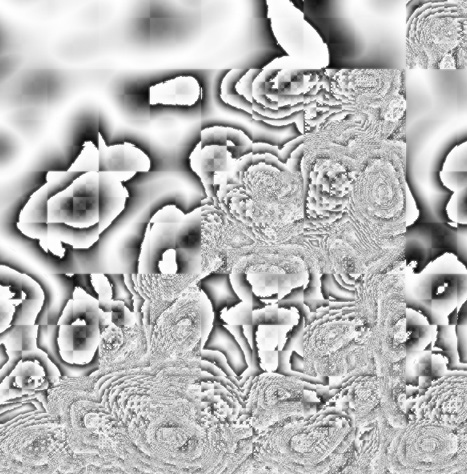
...take the original snippet and change the blendMode to subtract:
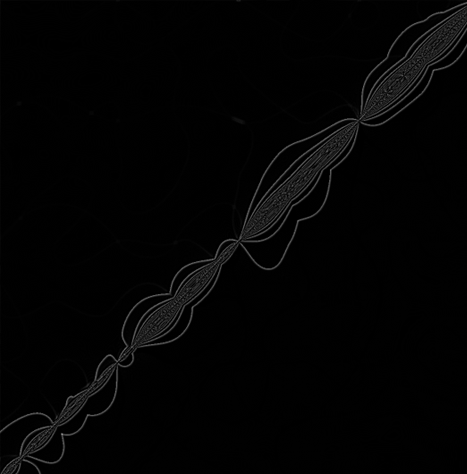
etc...