By Zevan | April 30, 2009
Actionscript:
-
[SWF(backgroundColor = 0x333333)]
-
-
import com.actionsnippet.qbox.*;
-
-
// setting debug = true, will use the Box2D debug rendering
-
var sim:QuickBox2D=new QuickBox2D(this, {debug:false, gravityY:10});
-
-
// creates static boxes on all sides of the screen
-
sim.createStageWalls({lineAlpha:0, fillColor:0xFF9900});
-
-
// add 25 boxes
-
for (var i:int = 0; i<25; i++){
-
var xp:Number = 3 + (i % 5);
-
var yp:Number = 1 + int( i / 5);
-
sim.addBox({x:xp, y:yp, width:1, height:1, fillColor: i * 10 <<16, lineAlpha:0, angularDamping:5});
-
}
-
-
sim.addBox({x:7, y:10, width:3, height:.2, angle:-.3, density:0,lineAlpha:0, fillColor:0xFF9900});
-
-
sim.addCircle({x:3, y:14, radius:2, fillColor:0xCC0000, lineColor:0x333333});
-
-
sim.addPoly({x:13, y:5, verts:[[1,0,2,2,1,1.33],[1,0,1,1.33,0,2]], angle: .4, density:1});
-
-
// begins the simulation
-
sim.start();
-
-
// all non-static objects can be dragged
-
sim.mouseDrag();
I've been messing with Box2D for the past few weeks - I decided to create a library (QuickBox2D) for quick prototyping. This snippet makes use of this library, so in order to run it you'll need to download the zip included at the end of this post...
If you don't already know, Box2D is a great physics library created by Erin Catto. It was ported to AS3 by Matthew Bush and John Nesky. You can download the AS3 version of the library here.
Today's snippet creates this swf (click any image to view demo):
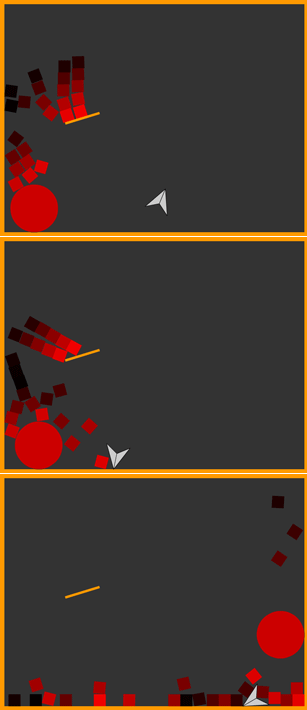
QuickBox2D
QuickBox2D simplifies Box2D instantiation and makes it easy to skin rigid bodies with Library assets... normally you need to do something like this to create a box in Box2D:
Actionscript:
-
var bodyDef:b2BodyDef = new b2BodyDef();
-
bodyDef.position.Set(3, 3);
-
var boxDef:b2PolygonDef = new b2PolygonDef();
-
boxDef.SetAsBox(1, 1);
-
boxDef.density = 1;
-
var body:b2Body = w.CreateBody(bodyDef);
-
body.CreateShape(boxDef);
-
body.SetMassFromShapes();
and this doesn't include setting up the physics world and the main loop to run the simulation... after writing a good deal of repetitive code I decided to make QuickBox2D. After writing lots of QuickBox2D style instantiation I decided to create an editor so that I could just draw circles, boxes, polygons and joints and then test and save them in real time (will be posting this editor in the near future). The next few posts will be about this mini-library and I'll be writing up some real documentation for it... as of now, it doesn't have any...
You can download Box2D here
You can download QuickBox2D here
and you can view some AS3 Box2D demos here
By Zevan | April 29, 2009
Actionscript:
-
[SWF(width = 800, height = 800)]
-
var a:Number = 0.19;
-
var b:Number = .9;
-
var c:Number = 1.3
-
var xn1:Number = 5;
-
var yn1:Number = 0;
-
var xn:Number, yn:Number;
-
-
var scale:Number =40;
-
var iterations:Number = 20000;
-
-
function f(x:Number):Number{
-
// too lazy to simplify this at the moment
-
return((x + .1 + x * (a - c) * x) / (1.1 + a * (c*c + a*a) * x * x )) * 1.3;
-
}
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(800,800,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
-
c += ((stage.stageWidth/2 - mouseX) / 8000 - c) / 2;
-
-
xn1 = 0;
-
yn1 = 0;
-
for (var i:int = 0; i<iterations; i++){
-
xn = xn1;
-
yn = yn1;
-
-
xn1 = -xn - a + c + f(yn);
-
yn1 = -xn + c * f(xn * yn);
-
canvas.setPixel( 380 + xn1 * scale, 450 + yn1 * scale, 0x000000);
-
}
-
}
I was randomly messing around with strange attractors a few weeks back and this is one of the better results.... I arbitrarily altered equations until I got a nice result. You can move your mouse left and right to alter some of the params. Here is what it looks like when your mouse is in the middle of the screen:
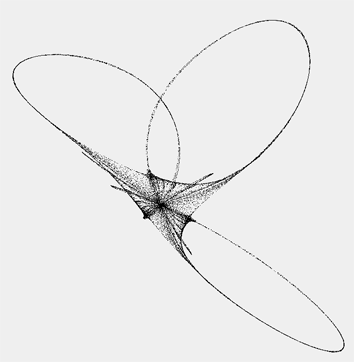
By Zevan | April 28, 2009
Actionscript:
-
var canvas:BitmapData=new BitmapData(400,400,false,0x000000);
-
addChild(new Bitmap(canvas));
-
-
var a:Number=-1.21;
-
var r:Rectangle=new Rectangle(0,0,3,5);
-
var halfWidth:Number=canvas.width/2;
-
var halfHeight:Number=canvas.height/2;
-
-
render();
-
-
function render():void{
-
for (var x:Number = -2; x<=2; x+=.01) {
-
for (var y:Number = -2; y<=2; y+=.02) {
-
-
// equation from : http://en.wikipedia.org/wiki/Bicuspid_curve
-
//(x^2 - a^2) * (x - a)^2 + (y^2 - a^2) * (y^2 - a^2) = 0
-
-
// unpoptimized:
-
// var e:Number = (x*x - a*a) * (x-a)*(x-a) + (y*y-a*a) * (y*y-a*a);
-
// optimized:
-
var x_a:Number=x-a;
-
// factoring: x^2 - a^2 = (x + a) * (x - a)
-
var y2_a2:Number = (y + a) * (y - a);
-
var e:Number = (x + a) * x_a * x_a * x_a + y2_a2 * y2_a2;
-
-
r.x=halfWidth+y*50;
-
r.y=halfHeight-x*100;
-
var col:Number = e * 50;
-
if (col <10){
-
col = Math.abs(col) + 70;
-
canvas.fillRect(r, col <<16 | col <<8 | col );
-
}
-
}
-
}
-
}
This is a variation on a post from a little while back.... it plots a modified Bicuspid that resembles a tooth:
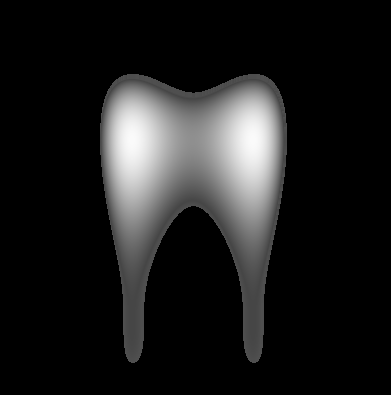
By Zevan | April 27, 2009
Actionscript:
-
package {
-
-
[SWF(width=1000,height=1000)]
-
import flash.display.*;
-
import flash.events.*;
-
public class RandomWalkTexture extends Sprite {
-
-
private var _canvas:BitmapData;
-
private var _populationNum:int=100;
-
private var _movers:Vector.<Mover>;
-
public function RandomWalkTexture() {
-
var sw:Number=stage.stageWidth;
-
var sh:Number=stage.stageHeight;
-
scaleX=scaleY=.25;
-
_canvas=new BitmapData(sw*4,sh*4,false,0x000000);
-
addChild(new Bitmap(_canvas));
-
-
_movers = new Vector.<Mover>();
-
for (var i:int = 0; i<_populationNum; i++) {
-
_movers[i]=new Mover(_canvas,sw*1.5+Math.random()*sw,sh*1.5+Math.random()*sh);
-
}
-
addEventListener(Event.ENTER_FRAME, onRun);
-
}
-
private function onRun(evt:Event):void {
-
for (var i:int = 0; i<200; i++) {
-
for (var j:int = 0; j<_populationNum; j++) {
-
_movers[j].run();
-
}
-
}
-
}
-
}
-
}
-
-
import flash.display.BitmapData;
-
class Mover {
-
public var x:Number;
-
public var y:Number;
-
public var velX:Number;
-
public var velY:Number;
-
public var speed:Number;
-
private var _canvas:BitmapData;
-
-
public function Mover(canvas:BitmapData, xp:Number, yp:Number) {
-
_canvas=canvas;
-
x=xp;
-
y=yp;
-
velX=0;
-
velY=0;
-
speed=Math.random()*5-2.5;
-
}
-
public function run():void {
-
x+=velX;
-
y+=velY;
-
_canvas.setPixel(x, y, 0xFFFFFF);
-
var dir:Number=int(Math.random()*4);
-
if (dir==0) {
-
velX=0;
-
velY=- speed;
-
} else if (dir == 1) {
-
velX=0;
-
velY=speed;
-
} else if (dir == 2) {
-
velX=- speed;
-
velY=0;
-
} else if (dir == 3) {
-
velX=speed;
-
velY=0;
-
}
-
}
-
}
This snippet is meant to be run as a document class. Nothing special here... this is just something I found laying around - the actual bitmap being drawn is rather big, so I recommending right clicking (control clicking on mac) on the swf and to zoom in and out.
Here are a few images:
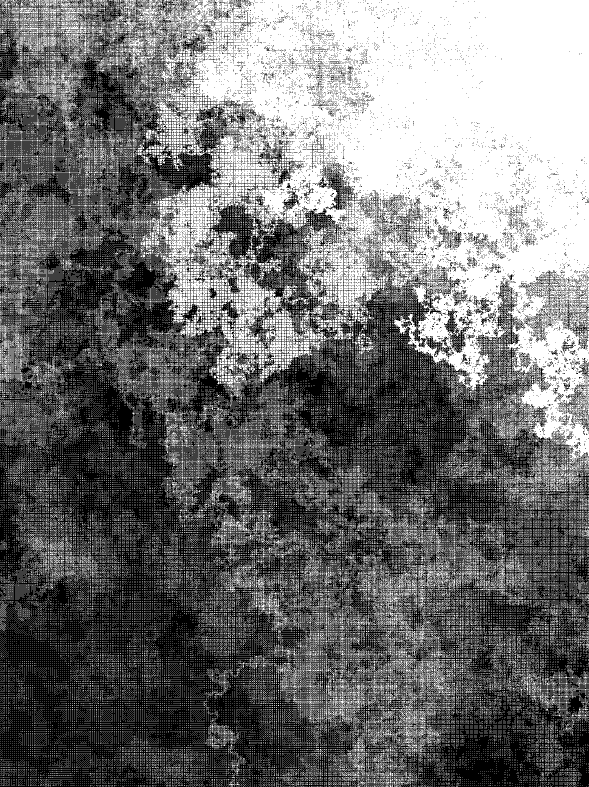
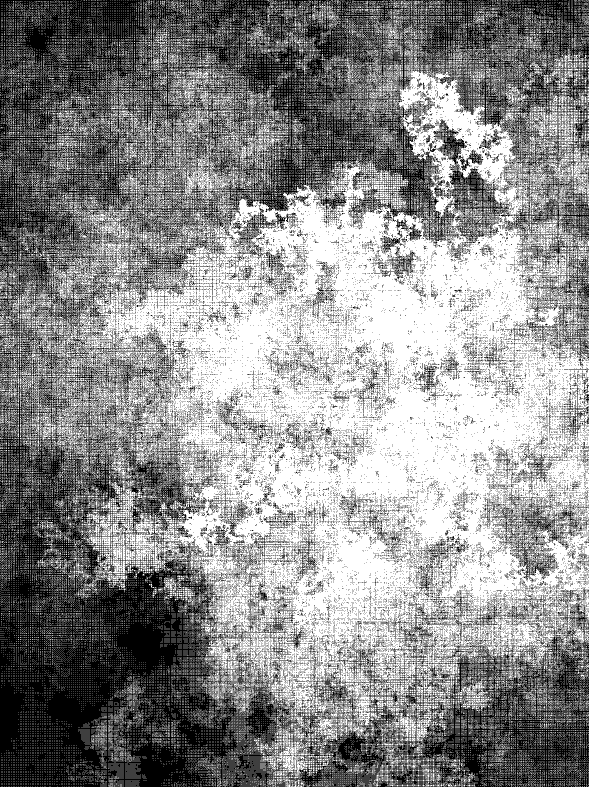
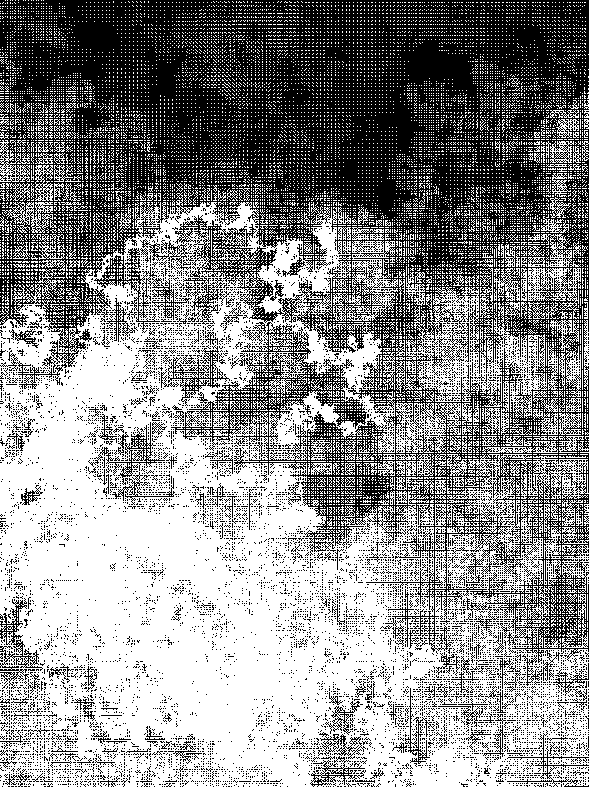
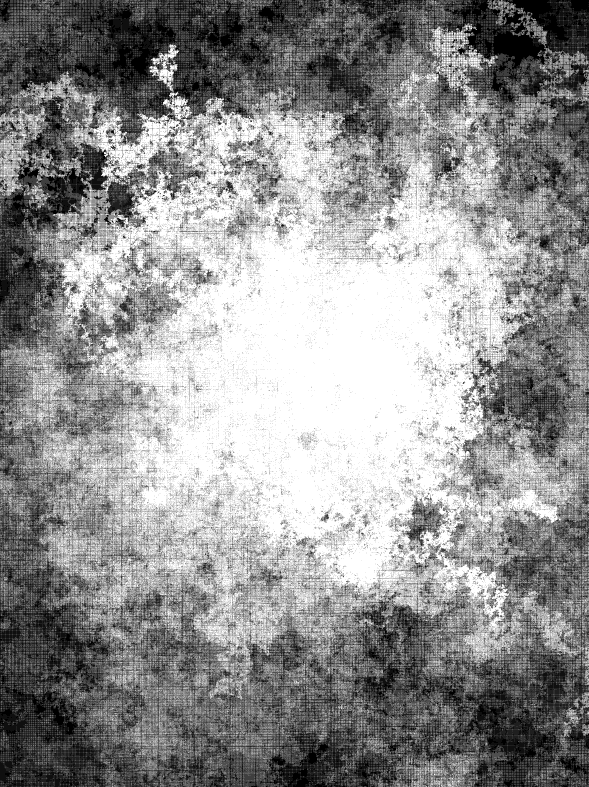
Posted in misc | Tagged actionscript, flash |