By Zevan | January 23, 2009
Actionscript:
-
[SWF(width=700, height=400, backgroundColor=0x000000, frameRate=30)]
-
-
var points:Array = new Array();
-
var index:int = -1;
-
function polar(thetaInc:Number, radius:Number):Point{
-
index++;
-
if (!points[index]) points[index] = 0;
-
return Point.polar(radius, points[index] += thetaInc)
-
}
-
///////////////////////////////////////////////////
-
// test it out:
-
-
var canvas:BitmapData = new BitmapData(700, 400, false, 0xFFFFFF);
-
-
addChild(new Bitmap(canvas, "auto", true));
-
-
var p0:Point = new Point(200, 200);
-
var p1:Point = new Point(500, 200);
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
var rad:Point = polar(.05, 4);
-
-
for (var i:int= 0; i<100; i++){
-
-
// reset index;
-
index = -1;
-
-
p0 = p0.add(polar(.025, 2).add(polar(-.05,rad.x)));
-
canvas.setPixel(p0.x, p0.y, 0x000000);
-
-
p1 = p1.add(polar(.025, 2).add(polar(-.05,rad.x).add(polar(.1, rad.y))));
-
canvas.setPixel(p1.x, p1.y, 0x000000);
-
}
-
}
This is pretty much the same as yesterdays... I just changed the way I use the polar() function to draw two more shapes:
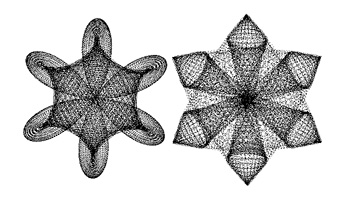
By Zevan | January 22, 2009
Actionscript:
-
[SWF(width=600, height=500, backgroundColor=0x000000, frameRate=30)]
-
var points:Array = new Array();
-
var index:int = -1;
-
function polar(thetaInc:Number, radius:Number):Point{
-
index++;
-
if (!points[index]) points[index] = 0;
-
return Point.polar(radius, points[index] += thetaInc);
-
}
-
///////////////////////////////////////////////////
-
// test it out:
-
-
var canvas:BitmapData = new BitmapData(600, 500, false, 0xFFFFFF);
-
-
addChild(new Bitmap(canvas, "auto", true));
-
-
var p0:Point = new Point(80, 100);
-
var p1:Point = new Point(270, 100);
-
var p2:Point = new Point(480, 40);
-
var p3:Point = new Point(170, 180);
-
var p4:Point = new Point(430, 300);
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
for (var i:int= 0; i<100; i++){
-
-
// reset index;
-
index = -1;
-
-
p0 = p0.add(polar(.2, 4).add(polar(-.4,2).add(polar(.05, 1))));
-
canvas.setPixel(p0.x, p0.y, 0x000000);
-
-
p1 = p1.add(polar(.1, 2).add(polar(-.2, 2).add(polar(.03, 1).add(polar(-.01,.5)))));
-
canvas.setPixel(p1.x, p1.y, 0x000000);
-
-
p2 = p2.add(polar(.08, 3 ).add(polar(-.2, -12).add(polar(2, 10))));
-
canvas.setPixel(p2.x, p2.y, 0x000000);
-
-
p3 = p3.add(polar(.08, 7).add(polar(-.2, -12).add(polar(2, 11))));
-
canvas.setPixel(p3.x, p3.y, 0x000000);
-
-
p4 = p4.add(polar(.025, 2).add(polar(-.05,1)));
-
canvas.setPixel(p4.x, p4.y, 0x000000);
-
}
-
}
The polar() function is the real trick here... the rest of the code just uses it to draw this:
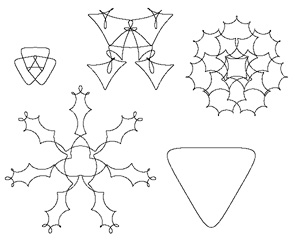
The Point.polar() function is just a conversion from polar to cartesian coords:
Actionscript:
-
x = radius * Math.cos(theta);
-
y = radius * Math.sin(theta);
By Zevan | January 21, 2009
SNIPPET ONE
SNIPPET TWO
I was very impressed when I saw Mario Klingemann's game of line in 3 lines of code blog post late last year. I've been meaning to post about it for awhile - finally got around to it.
Mario Klingemann is the developer of Peacock... an excellent node based image generator written in ActionScript.
BitmapData.paletteMap() is very powerful. I haven't done much with it, but I have some ideas floating around.
For those of you that are game of live savvy... try other rule sets from the lexicon... my personal favorite has always been coral (45678/3). Here's a link to some more.
Another short game of life recently appeared on on the 25lines.com forums.... here... the forum post features a nice explanation for using a ConvolutionFilter and Threshold to create The Game of Life. The technique is so small and impressive looking that I figured I'd post it as is right here:
Actionscript:
-
addChild(new Bitmap(new BitmapData(stage.stageWidth, stage.stageHeight, false, 0)))["bitmapData"].noise(int(Math.random()*int.MAX_VALUE),0,255,7,true);
-
addEventListener(Event.ENTER_FRAME, function (e) {
-
getChildAt(0)["bitmapData"].applyFilter(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), new ConvolutionFilter(3, 3, [3,3,3,3,2,3,3,3,3],255,0,true,false,0,1));
-
getChildAt(0)["bitmapData"].threshold(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), "==", 8, 0xFFFFFFFF, 0xFC);
-
getChildAt(0)["bitmapData"].threshold(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), "!=", 0xFFFFFFFF, 0x00000000)})
The above code is by Daniil Tutubalin 25lines.com forums.
UPDATE:
Since writing this post the 25lines.com forum thread has grown to include a 4 line version and some additional discussion about this topic... be sure to check it out.
By Zevan | January 20, 2009
Actionscript:
-
var blends:Array = [BlendMode.ADD, BlendMode.DARKEN, BlendMode.DIFFERENCE, BlendMode.HARDLIGHT, BlendMode.INVERT, BlendMode.LIGHTEN, BlendMode.MULTIPLY, BlendMode.OVERLAY, BlendMode.SCREEN, BlendMode.SUBTRACT];
and a little later...
Actionscript:
-
displace.perlinNoise(150,150, 3, 30, true, false,0,true);
-
var currentBlend:String = blends[ blendCount % blends.length];
-
displace.draw(radial, null ,null, currentBlend);
-
blendCount++;
The above are excepts from a recommendation I made in the comments of yesterdays post...
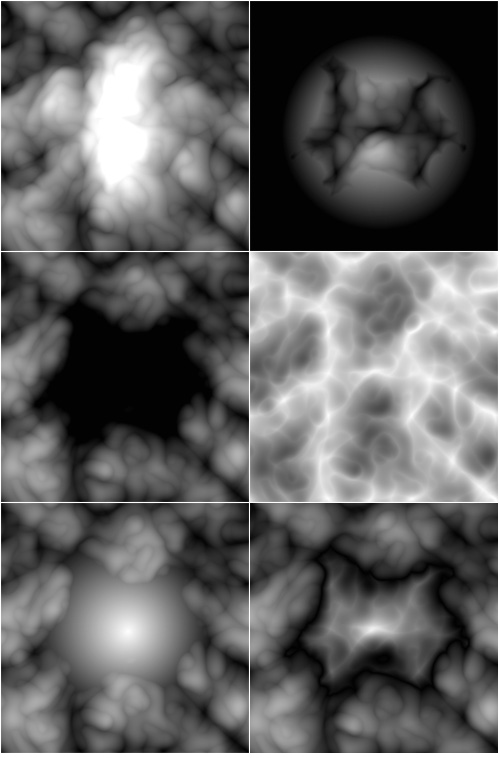
Try some different blend modes.... take a look at the swf here.