Actionscript:
-
var pointNum:int = 3000;
-
x = stage.stageWidth / 2;
-
y = stage.stageHeight / 2;
-
-
var verts:Vector.<Number> = new Vector.<Number>();
-
var tVerts:Vector.<Number> = new Vector.<Number>();
-
var pVerts:Vector.<Number> = new Vector.<Number>();
-
var uv:Vector.<Number> = new Vector.<Number>();
-
var cmds:Vector.<int> = new Vector.<int>();
-
-
var index:int = 0;
-
var half:Number = pointNum / 20000;
-
for (var i:int = 0; i<pointNum; i+=3){
-
verts[i] = 0.08 * Math.cos(i * Math.PI / 180);
-
verts[i+1] = 0.08 * Math.sin(i * Math.PI / 180);
-
verts[i+2] = i / 10000 - half;
-
cmds[index++] = 2;
-
}
-
cmds[0] = 1;
-
-
var proj:PerspectiveProjection = new PerspectiveProjection();
-
proj.fieldOfView = 45;
-
var projMat:Matrix3D = proj.toMatrix3D();
-
var m:Matrix3D = new Matrix3D();
-
var dx:Number = 0, dy:Number = 0;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
m.identity();
-
dx += (mouseX - dx) / 4;
-
dy += (mouseY - dy) / 4;
-
m.appendRotation(dy,Vector3D.X_AXIS);
-
m.appendRotation(dx,Vector3D.Y_AXIS);
-
m.appendTranslation(0,0,0.5);
-
m.transformVectors(verts, tVerts);
-
Utils3D.projectVectors(projMat, tVerts, pVerts, uv);
-
graphics.clear();
-
graphics.lineStyle(3,0x000000);
-
graphics.drawPath(cmds, pVerts);
-
}
This snippet draws a spring shape in 3D with perspective. This is the first snippet where I've made use of the PerspectiveProjection class. So if your wondering how to add perspective to your Utils3D.projectVectors code... this is one way to do it...
Have a look at the swf...
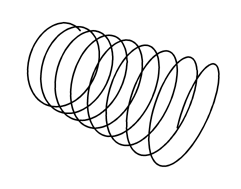
Actionscript:
-
var pointNum:int = 20000;
-
var radius:int = 150;
-
-
var canvas:BitmapData = new BitmapData(400,400,false, 0x000000);
-
addChild(new Bitmap(canvas));
-
var verts:Vector.<Number> = new Vector.<Number>();
-
var pVerts:Vector.<Number> = new Vector.<Number>();
-
var uv:Vector.<Number> = new Vector.<Number>();
-
-
for (var i:int = 0; i<pointNum; i+=3){
-
var xp:Number = Math.random() * 400 - 200;
-
var yp:Number = Math.random() * 400 - 200;
-
var zp:Number = Math.random() * 400 - 200;
-
var dist:Number = Math.sqrt(xp * xp + yp * yp + zp * zp);
-
// normalize and scale x,y,z
-
verts[i] = xp / dist * radius;
-
verts[i+1] = yp / dist * radius;
-
verts[i+2] = zp / dist * radius;
-
}
-
-
var m:Matrix3D = new Matrix3D();
-
var dx:Number = 0, dy:Number = 0;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
m.identity();
-
dx += (mouseX - dx) / 4;
-
dy += (mouseY - dy) / 4;
-
m.appendRotation(dx, Vector3D.X_AXIS);
-
m.appendRotation(dy, Vector3D.Y_AXIS);
-
m.appendTranslation(200,200,0);
-
Utils3D.projectVectors(m, verts, pVerts, uv);
-
canvas.fillRect(canvas.rect, 0x000000);
-
for (var i:int = 0; i<pVerts.length; i+=2){
-
canvas.setPixel(pVerts[i], pVerts[i + 1], 0xFFFFFF);
-
}
-
}
This snippet shows a quick way to randomly place a bunch of xyz coordinates on the surface of a sphere. I saw this trick in an OpenGL book a few years back - dug around my books but couldn't find it... If I find it I'll update this post.
The trick is achieved by normalizing the vector defined by each 3D coordinate...
Have a look at the swf...
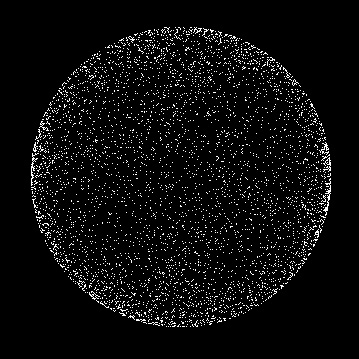
Actionscript:
-
[SWF(width=800, height=600)]
-
var dupes:int = 5
-
var pntNum:int = 180;
-
-
var hh:Number = stage.stageHeight / 2;
-
var points:Vector.<Number> = new Vector.<Number>();
-
var vel:Vector.<Number> = new Vector.<Number>();
-
var cmds:Vector.<int> = new Vector.<int>();
-
var vectors:Vector.<Shape> = new Vector.<Shape>();
-
-
for (var i:int = 0; i<dupes; i++){
-
vectors[i] = Shape(addChildAt(new Shape(),0));
-
vectors[i].x = 10 * i;
-
vectors[i].y = -10 * i;
-
}
-
var index:int = 0;
-
for (i = 0; i<pntNum; i++){
-
points[index++] = 10 + i * 4;
-
points[index++] = hh;
-
cmds[i] = 2;
-
vel[i] = 0;
-
}
-
cmds[0] = 1;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
index = 1;
-
points[index] += (mouseY - points[index]) / 12;
-
for (var i:int = 3; i<points.length; i+=2){
-
points[i] += vel[index];
-
vel[index] += ((points[i] - points[i - 2]) * -0.16 - vel[index]) / 4;
-
index++;
-
}
-
for (i = 0; i<dupes; i++){
-
var c:uint = 255 - 255 / i;
-
with (vectors[i].graphics){
-
clear();
-
lineStyle(0,c <<16 | c <<8 | c);
-
drawPath(cmds, points);
-
}
-
}
-
}
This snippet uses elasticity to create an interesting wave effect. I first stumbled upon this technique back in my director days...
Have a look at the swf here...
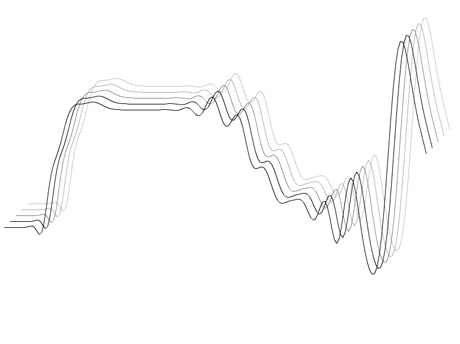
Actionscript:
-
var a:Array = [true, true, true, false, false, true, true, true, false];
-
-
var counter:int = 0;
-
var prev:Boolean;
-
var summary:Array = [];
-
for (var i:int = 1; i<a.length; i++){
-
prev = a[i - 1]
-
counter++;
-
if (prev != a[i]){
-
if (prev){
-
summary.push("true: "+ counter);
-
}else{
-
summary.push("false: "+ counter);
-
}
-
counter = 0;
-
}
-
}
-
summary.push(a[i-1].toString()+": "+ (counter+1));
-
-
trace(summary);
-
-
/** outputs:
-
true: 3,false: 2,true: 3,false: 1
-
*/
This is a handy way to summarize the contents of an array of boolean values.