By Zevan | January 25, 2009
Actionscript:
-
[SWF(width=600, height=400, backgroundColor=0x000000, frameRate=30)]
-
// make some ships
-
for (var i:int = 0; i<10; i++) makeShip();
-
//
-
function makeShip():void {
-
// setup vars
-
var char:Object ={posX:Math.random()*stage.stageWidth, posY:Math.random()*stage.stageHeight, velX:0, velY:0, rad:4, theta:Math.random()*6.28, thetaDest:Math.random()*6.28, mc: MovieClip(addChild(new MovieClip()))}
-
// draw and position the char
-
with(char.mc) graphics.lineStyle(0,0xFFFFFF), graphics.moveTo(-15, -5), graphics.lineTo(0, 0), graphics.lineTo(-15, 5), x=char.posX, y=char.posY;
-
// loop
-
addEventListener(Event.ENTER_FRAME, function(){
-
// change position based on velocity, .9 friction
-
with (char) posX += velX *= .9, posY += velY *= .9;
-
// randomize radius and theta
-
if (int(Math.random()*10) == 1) char.thetaDest += Math.random()*2-1;
-
if (int(Math.random()*90) == 1) char.rad = Math.random()*4 + 4
-
// apply changes to velocity
-
char.theta += (char.thetaDest - char.theta) / 12;
-
char.velX = char.rad * Math.cos(char.theta);
-
char.velY = char.rad * Math.sin(char.theta);
-
// screen bounds
-
if (char.posX> 620) char.posX = -20;
-
if (char.posX <-20) char.posX = 620;
-
if (char.posY> 420) char.posY = -20;
-
if (char.posY <-20) char.posY = 420;
-
// rotation
-
char.mc.rotation = (Math.abs(char.mc.y - char.posY)>.7 || Math.abs(char.mc.x - char.posX)> .7) ? Math.atan2(char.posY - char.mc.y,char.posX - char.mc.x) / Math.PI * 180 : char.mc.rotation;
-
// shoot
-
if(int(Math.random()*100) <20) shootBullet(char, 5 * Math.cos(char.mc.rotation * Math.PI / 180)+ char.velX, 5 * Math.sin(char.mc.rotation * Math.PI / 180) + char.velY);
-
// apply position
-
with(char) mc.x = posX, mc.y = posY;
-
});
-
}
-
//
-
function shootBullet(char:Object, vx:Number, vy:Number):void{
-
var b:MovieClip = MovieClip(addChild(new MovieClip()));
-
b.life = 0;
-
with(b) graphics.beginFill(0xFFFFFF), graphics.drawCircle(0,0,2), x = char.mc.x, y = char.mc.y;
-
b.addEventListener(Event.ENTER_FRAME, function(){
-
with (b) x += vx, y += vy, life++;
-
if (b.life> 30) b.removeEventListener(Event.ENTER_FRAME, arguments.callee), removeChild(b);
-
});
-
}
An asteroids inspired code snippet. See the swf here.
Also posted in motion | Tagged actionscript, flash |
By Zevan | January 21, 2009
SNIPPET ONE
SNIPPET TWO
I was very impressed when I saw Mario Klingemann's game of line in 3 lines of code blog post late last year. I've been meaning to post about it for awhile - finally got around to it.
Mario Klingemann is the developer of Peacock... an excellent node based image generator written in ActionScript.
BitmapData.paletteMap() is very powerful. I haven't done much with it, but I have some ideas floating around.
For those of you that are game of live savvy... try other rule sets from the lexicon... my personal favorite has always been coral (45678/3). Here's a link to some more.
Another short game of life recently appeared on on the 25lines.com forums.... here... the forum post features a nice explanation for using a ConvolutionFilter and Threshold to create The Game of Life. The technique is so small and impressive looking that I figured I'd post it as is right here:
Actionscript:
-
addChild(new Bitmap(new BitmapData(stage.stageWidth, stage.stageHeight, false, 0)))["bitmapData"].noise(int(Math.random()*int.MAX_VALUE),0,255,7,true);
-
addEventListener(Event.ENTER_FRAME, function (e) {
-
getChildAt(0)["bitmapData"].applyFilter(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), new ConvolutionFilter(3, 3, [3,3,3,3,2,3,3,3,3],255,0,true,false,0,1));
-
getChildAt(0)["bitmapData"].threshold(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), "==", 8, 0xFFFFFFFF, 0xFC);
-
getChildAt(0)["bitmapData"].threshold(getChildAt(0)["bitmapData"], getChildAt(0)["bitmapData"].rect, new Point(), "!=", 0xFFFFFFFF, 0x00000000)})
The above code is by Daniil Tutubalin 25lines.com forums.
UPDATE:
Since writing this post the 25lines.com forum thread has grown to include a 4 line version and some additional discussion about this topic... be sure to check it out.
By Zevan | January 20, 2009
Actionscript:
-
var blends:Array = [BlendMode.ADD, BlendMode.DARKEN, BlendMode.DIFFERENCE, BlendMode.HARDLIGHT, BlendMode.INVERT, BlendMode.LIGHTEN, BlendMode.MULTIPLY, BlendMode.OVERLAY, BlendMode.SCREEN, BlendMode.SUBTRACT];
and a little later...
Actionscript:
-
displace.perlinNoise(150,150, 3, 30, true, false,0,true);
-
var currentBlend:String = blends[ blendCount % blends.length];
-
displace.draw(radial, null ,null, currentBlend);
-
blendCount++;
The above are excepts from a recommendation I made in the comments of yesterdays post...
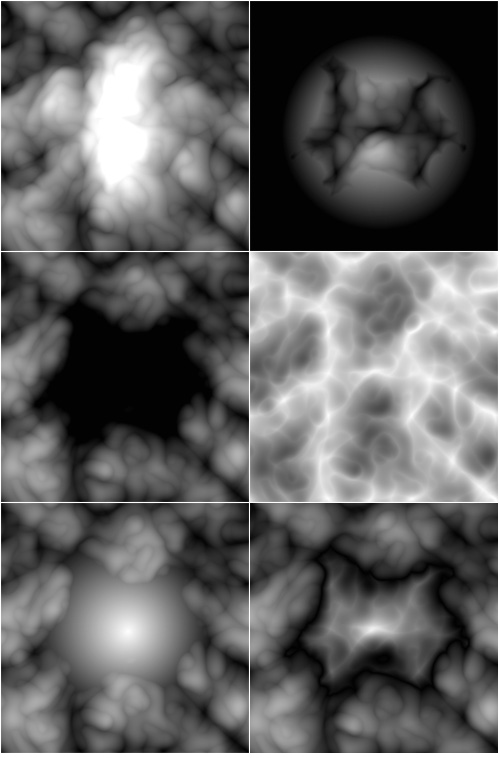
Try some different blend modes.... take a look at the swf here.
Also posted in 3D, BitmapData | Tagged actionscript, flash |
By Zevan | January 19, 2009
Actionscript:
-
[SWF(width=500, height=500, backgroundColor=0xCCCCCC, frameRate=30)]
-
-
var canvas:BitmapData = new BitmapData(500, 500, true, 0xFF000000);
-
addChild(new Bitmap(canvas));
-
-
// create a radial gradient
-
var radial:Shape = new Shape();
-
var m:Matrix = new Matrix()
-
m.createGradientBox(500,500,0,0,0);
-
radial.graphics.beginGradientFill(GradientType.RADIAL, [0xFFFFFF, 0x000000], [1, 1], [0, 200], m, SpreadMethod.PAD);
-
-
radial.graphics.drawRect(0,0,500,500);
-
radial.x = radial.y = 0;
-
-
var displace:BitmapData = new BitmapData(500, 500, false,0xFF000000);
-
displace.perlinNoise(150,150, 3, 30, true, false,0,true);
-
-
// try different blendmodes here
-
displace.draw(radial, null ,null, BlendMode.LIGHTEN);
-
-
var displacementMap:DisplacementMapFilter = new DisplacementMapFilter(displace, new Point(0,0), 1, 1, 0, 0, DisplacementMapFilterMode.WRAP);
-
-
var scale:Number = 50 / stage.stageWidth;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.copyPixels(displace, canvas.rect, new Point(0,0));
-
-
displacementMap.scaleX = 25 - mouseX * scale ;
-
displacementMap.scaleY = 25 - mouseY * scale ;
-
-
canvas.applyFilter(canvas, canvas.rect, new Point(0,0), displacementMap);
-
}
This one is hard to describe, so take a look at the swf first.
I think displacement maps are underused - they're very powerful. In this snippet I use a DisplacementMapFilter to create a parallax effect on some perlin noise and a radial gradient. The result is an abstract texture that looks 3D.
I got the idea from a demo for the alternative game engine. The first time I saw this demo I had no idea how they were doing it... but after looking at it a few times, I noticed some distortion around the neck area of the model... at that point I recognized it as a displacement map and whipped up a demo (using a drawings as the source image). The alternativa demo also has some color stuff happening to simulate lighting... I'm assuming that's done with a ColorMatrixFilter or two, but I'm not sure.
As in the alternativa demo, this technique could be used with 2 images... one depth map rendered out from your favorite 3D app and one textured render....
Also posted in 3D, BitmapData | Tagged actionscript, flash |