By Zevan | February 23, 2009
Actionscript:
-
g=graphics;
-
mt=g.moveTo;
-
lt=g.lineTo;
-
ls=g.lineStyle;
-
m=Math;
-
r=m.random;
-
s=m.sin;
-
i=0;
-
o={};
-
function f(e){
-
s=150,x=y=s,z=-s,c=(!i)?addChild(new Bitmap(new BitmapData(s,s))).bitmapData:c;while(i<22500)i++,c.setPixel(i%s,i/s,(i%s|i/s)*mouseX);i=1;
-
}
-
addEventListener("enterFrame",f);
Have to link to this very fun new contest:
http://tweetcoding.machine501.com/
http://gskinner.com/playpen/tweetcoding.html
I may just have to get a twitter account...
Posted in misc | Tagged actionscript, flash |
By Zevan | February 23, 2009
Actionscript:
-
var clock:Sprite = Sprite(addChild(new Sprite()));
-
clock.x = clock.y = 150;
-
-
var bg:Shape = Shape(clock.addChild(new Shape()));
-
with (bg.graphics) lineStyle(2, 0x666666), beginFill(0xEFEFEF), drawCircle(0,0,110);
-
-
var hHand:Shape = clockHand(6, 50);
-
var mHand:Shape = clockHand(2, 80);
-
var sHand:Shape = clockHand(1, 90);
-
-
var center:Shape = Shape(clock.addChild(new Shape()));
-
with (center.graphics) beginFill(0x000000), drawCircle(0,0,5);
-
-
var hInc:Number = 360/24;
-
var msInc:Number = 360/60 ;
-
var nOff:Number = 6;
-
var verdana:TextFormat = new TextFormat("Verdana", 8);
-
// add numbers to clock
-
for (var i:int = 0; i<24; i++){
-
var ang:Number = (i * hInc - 90) * Math.PI/180;
-
createNumber(70,ang, i.toString());
-
var ms:Number = i * 2.5;
-
if (ms % 5 == 0){
-
createNumber(95, ang, ms.toString());
-
}
-
}
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
var date:Date = new Date();
-
hHand.rotation = hInc * date.getHours();
-
mHand.rotation = msInc * date.getMinutes();
-
sHand.rotation = msInc * date.getSeconds();
-
}
-
-
function clockHand(thickness:Number, leng:Number):Shape{
-
var hand:Shape = Shape(clock.addChild(new Shape()));
-
with (hand.graphics) {
-
lineStyle(thickness, 0x000000, 1, true, LineScaleMode.NORMAL, CapsStyle.SQUARE);
-
lineTo(0,-leng);
-
}
-
return hand;
-
}
-
-
function createNumber(radius:Number, theta:Number, str:String):void{
-
var t:TextField = TextField(clock.addChild(new TextField()));
-
with (t) defaultTextFormat = verdana, t.autoSize = "left";
-
t.text = str;
-
t.x = radius * Math.cos(theta) - nOff;
-
t.y = radius* Math.sin(theta) - nOff;
-
}
I was watching a movie the other day and I saw a 24 hour clock in the background of one of the shots. After the movie I coded this snippet. It draws a very basic clock with an hour hand that takes 24 hours to go full circle...
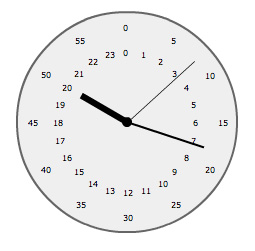
Posted in misc | Tagged actionscript, flash |
By Zevan | February 22, 2009
Actionscript:
-
addChild(new TextField());
-
getChildAt(0)["text"] = "Hello World";
Notice that square bracket syntax makes it so we don't need to typecast. Take a look at the other way:
Actionscript:
-
addChild(new TextField());
-
TextField(getChildAt(0)).text = "Hello World";
Typecasting is the way to go.... the square bracket technique is just an interesting trick.
I wrote this after somehow stumbling on this very entertaining page - it shows hello world written in approximately 200 different languages...
Also posted in dynamic | Tagged actionscript, flash |
By Zevan | February 19, 2009
Actionscript:
-
// best thing about this are the random seeds
-
var s1:Number= 0xFFFFFF;
-
var s2:Number = 0xCCCCCC;
-
var s3:Number= 0xFF00FF;
-
-
// saw this algorithm on this somewhat annoying thread:
-
// http://www.reddit.com/r/programming/comments/7yjlc/why_you_should_never_use_rand_plus_alternative/
-
// additional googling brought me to this: http://wakaba.c3.cx/soc/kareha.pl/1100499906/
-
// and finally to this ( didn't understand most of this one) www.ams.org/mcom/1996-65-213/S0025-5718-96-00696-5/S0025-5718-96-00696-5.pdf
-
-
function rand():Number {
-
s1=((s1&4294967294)<<12)^(((s1<<13)^s1)>>19);
-
s2=((s2&4294967288)<<4)^(((s2<<2)^s2)>>25);
-
s3=((s3&4294967280)<<17)^(((s3<<3)^s3)>>11);
-
var r:Number = (s1^s2^s3) * 2.3283064365e-10;
-
r = (r<0) ? r+=1 : r;
-
return r;
-
}
-
-
// see a visual comparison between this and actionscript's Math.random()
-
-
var canvas:BitmapData = new BitmapData(400,400,false, 0xCCCCCC);
-
addChild(new Bitmap(canvas));
-
-
var posX:Number;
-
var posY:Number;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function onLoop(evt:Event):void {
-
-
for (var i:int = 0; i<100; i++){
-
posX = rand() * 180
-
posY = rand() * 180
-
canvas.setPixel( 100 + posX, 10 + posY, 0x000000);
-
}
-
-
for (i = 0; i<100; i++){
-
posX = Math.random() * 180
-
posY = Math.random() * 180
-
canvas.setPixel( 100 + posX, 210 + posY, 0x000000);
-
}
-
}
The above snippet demo's an alternative random number generator that uses the Tausworthe method.
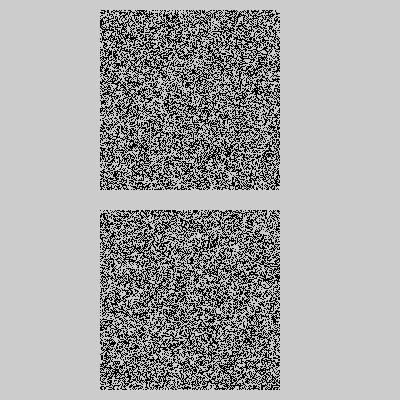
Top square is Tausworthe and bottom is Math.random()
I don't actually know much about this, or fully understand how it works. I just saw it on a random reddit thread, googled about it for a few minutes and then ported it to actionscript.
There's lots of talk about what the BEST random number generator is.... which one is the fastest, which one is the most random. etc... I don't really know much about that, especially since I'm just playing around with code and I don't need a RNG for some scientific experiment. For me, what's nice about this approach is the three random seeds. For some reason, Math.random() doesn't have a place where you can enter a seed for random numbers. Random seeds are very useful when you want to do something random but want to be able to replicate the random results at a later time. For instance:
Actionscript:
-
var s1:Number= 0xFFFFFF;
-
var s2:Number = 0xCCCCCC;
-
var s3:Number= 0xFF00FF;
-
-
trace("first three values:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
s1 = 0xFF;
-
s2 = 0xEFEFEF;
-
s3 = 19008;
-
-
trace("\nfirst three values with different seeds:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
s1 = 0xFFFFFF;
-
s2 = 0xCCCCCC;
-
s3 = 0xFF00FF;
-
-
trace("\noriginal three values:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
function rand():Number {
-
s1=((s1&4294967294)<<12)^(((s1<<13)^s1)>>19);
-
s2=((s2&4294967288)<<4)^(((s2<<2)^s2)>>25);
-
s3=((s3&4294967280)<<17)^(((s3<<3)^s3)>>11);
-
var r:Number = (s1^s2^s3) * 2.3283064365e-10;
-
r = (r<0) ? r+=1 : r;
-
return r;
-
}
-
-
/*
-
outputs:
-
first three values:
-
0.051455706589931385
-
0.050584114155822715
-
0.417276361484596
-
-
first three values with different seeds:
-
0.6032885762463134
-
0.9319786790304015
-
0.8631882804934321
-
-
original three values:
-
0.051455706589931385
-
0.050584114155822715
-
0.417276361484596
-
*/
I stumbled upon a bunch of other random number algorithms, maybe I'll throw them in a class in the next couple days.
Also posted in Number | Tagged actionscript, flash |