By Zevan | March 15, 2010
Actionscript:
-
[SWF(width = 500, height=500, backgroundColor=0x000000)]
-
-
var clockNum:int = 100;
-
var clocks:Vector.<Function> = new Vector.<Function>(clockNum, true);
-
-
var clockContainer:Sprite = Sprite(addChild(new Sprite()));
-
clockContainer.x = stage.stageWidth / 2;
-
clockContainer.y = stage.stageHeight / 2;
-
buildClocks();
-
runClocks();
-
-
function buildClocks():void{
-
for (var i:int = 0; i<clockNum; i++){
-
var theta:Number = Math.random() * Math.PI * 2;
-
var radius:Number = Math.random() * 200;
-
var xp:Number = radius * Math.cos(theta);
-
var yp:Number = radius * Math.sin(theta);
-
clocks[i] = makeClock(xp,yp,Math.random() * Math.PI * 2);
-
}
-
}
-
function runClocks():void{
-
addEventListener(Event.ENTER_FRAME, onRunClocks);
-
}
-
function onRunClocks(evt:Event):void{
-
for (var i:int = 0; i<clockNum; i++){
-
clocks[i]();
-
}
-
clockContainer.rotationX = clockContainer.mouseY / 30;
-
clockContainer.rotationY = -clockContainer.mouseX / 30;
-
}
-
function makeClock(x:Number, y:Number, time:Number=0):Function{
-
var radius:Number = Math.random() * 20 + 5;
-
var border:Number = radius * 0.2;
-
var smallRadius:Number = radius - radius * 0.3;
-
-
var clock:Sprite = Sprite(clockContainer.addChild(new Sprite()));
-
clock.x = x;
-
clock.y = y;
-
clock.z = 100 - Math.random() * 200;
-
clock.rotationX = Math.random() * 40 - 20;
-
clock.rotationY = Math.random() * 40 - 20;
-
clock.rotationZ = Math.random() * 360;
-
return function():void{
-
with (clock.graphics){
-
clear();
-
lineStyle(1,0xFFFFFF);
-
drawCircle(0,0,radius + border);
-
var xp:Number = smallRadius * Math.cos(time/2);
-
var yp:Number = smallRadius * Math.sin(time/2);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
xp = radius * Math.cos(time);
-
yp = radius * Math.sin(time);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
}
-
time+=0.1;
-
}
-
}
You can go check the swf out at wonderfl.net...
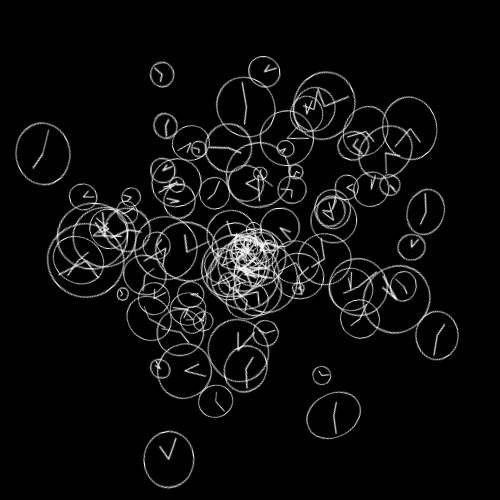
Also posted in 3D, misc, motion | Tagged actionscript, as3, flash |
If you're at all interested in watching me free from code. I recorded a video of me coding this snippet (which is about 11 minutes long or so).
In the video I create a few functions that allow you to draw shapes like these:
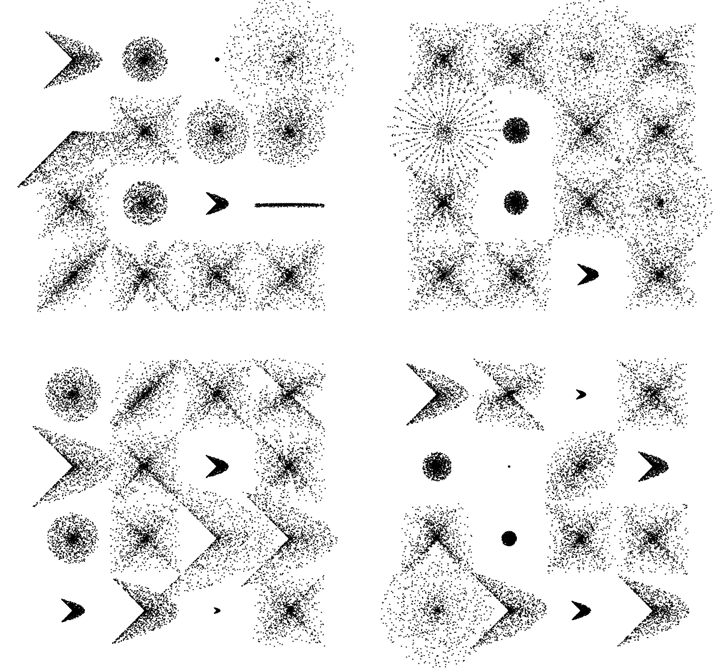
Mathematically this stuff is really simple ... the free form nature of the video takes a less technical perspective as you'll see (I even made a few funny mistakes).
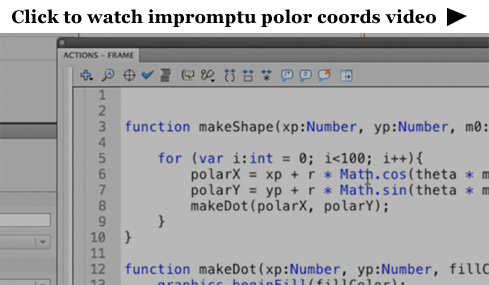
Actionscript:
-
[SWF(width = 600, height = 600)]
-
var dotNum:int = 1000;
-
var dotRad:Number = 0.5;
-
-
x = 120
-
y = 100;
-
-
// extra stuff to display what the functions can do
-
stage.addEventListener(MouseEvent.CLICK, onDrawAll);
-
-
function onDrawAll(evt:Event):void{
-
graphics.clear();
-
for (var i:int = 0; i<16; i++){
-
var m:Number;
-
-
var rad:Number = 120;
-
var xp:Number = i % 4 * rad
-
var yp:Number = int(i / 4) * rad
-
-
var type:int = int(Math.random() * 4);
-
if (type == 0){
-
makeShape(xp, yp, rad-60, Math.random() , 1);
-
}else if (type == 1){
-
makeShape(xp, yp, rad-60, 1, Math.random());
-
}
-
else if (type == 2){
-
m = Math.random() * 2;
-
makeShape(xp, yp, rad-Math.random()*120, m, m);
-
}
-
else if (type == 3){
-
m = Math.random() * 2;
-
makeShape(xp, yp, rad-Math.random()*120, m, m/2);
-
}
-
}
-
}
-
-
// main part from the video
-
function makeShape(xp:Number, yp:Number,
-
maxRad:Number = 100,m0:Number=1,
-
m1:Number=1):void{
-
var polarX:Number;
-
var polarY:Number;
-
var radius:Number;
-
graphics.lineStyle(0, 0);
-
var theta:Number = Math.random() * Math.PI * 2;
-
for (var i:int = 0; i<dotNum; i++){
-
radius = Math.random() * maxRad
-
polarX = xp + radius * Math.cos(theta * m0);
-
polarY = yp + radius * Math.sin(theta * m1);
-
theta += 0.1;
-
-
makeDot(polarX, polarY);
-
-
}
-
}
-
-
function makeDot(xp:Number, yp:Number, fillColor:uint = 0x000000):void{
-
graphics.beginFill(fillColor);
-
graphics.drawCircle(xp, yp, dotRad);
-
graphics.endFill();
-
}
Here it is over at wonderf:
By Zevan | January 20, 2010
Today's quiz is not multiple choice. Instead, your task is to write a function that draws stairs that look like this:
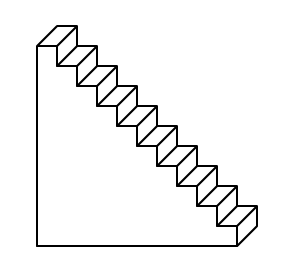
Your function should have the following arguments:
drawStairs(graphics, stairNum);
// you may include additional arguments for size, depth etc..
Feel free to post your solution in the comments.
I'll post my solution for this in the comments tomorrow. There is also another multiple choice quiz in the pipeline for tomorrow...
BONUS: Try to use as few Graphics class method calls as possible.
You can see my solutions here.
Also posted in Quiz | Tagged actionscript, as3, flash, Quiz |
By Zevan | December 11, 2009
Actionscript:
-
[SWF(width = 700, height=700, frameRate=12)]
-
var cmds:Array = [];
-
-
var loader:URLLoader = new URLLoader();
-
var req:URLRequest = new URLRequest("http://search.twitter.com/search.atom");
-
var vars:URLVariables = new URLVariables();
-
vars.q = "#asgraph";
-
// results per page
-
vars.rpp = "100";
-
vars.page = 1;
-
-
req.data = vars;
-
req.method = URLRequestMethod.GET;
-
-
loader.addEventListener(Event.COMPLETE, onLoaded);
-
loader.load(req);
-
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.defaultTextFormat = new TextFormat("_sans", 12);
-
with (txt){ x=10, y=10, autoSize="left"; }
-
txt.htmlText = "loading...";
-
-
function onLoaded(evt:Event):void{
-
removeChild(txt);
-
var searchData:XML = new XML(loader.data);
-
var atom:Namespace = searchData.namespace("");
-
-
var leng:int = searchData.atom::entry.length() -1;
-
for (var i:int = leng; i>=0; i--){
-
var cmd:String =
-
searchData.atom::entry[i].atom::title.toString().replace(/\#asgraph/g,
-
"");
-
// added this to ignore words starting with @
-
cmd = cmd.replace(/@(\w+)/g, "");
-
cmds.push(cmd);
-
}
-
-
var time:Timer = new Timer(100, cmds.length);
-
time.addEventListener(TimerEvent.TIMER, onTick);
-
time.start();
-
}
-
function onTick(evt:TimerEvent):void{
-
render(parseFunctions(cmds[evt.target.currentCount - 1]));
-
graphics.endFill();
-
graphics.lineStyle();
-
}
-
-
// parse and run Graphics class commands
-
function parseFunctions(dat:String):Array{
-
var a:Array = dat.split(";") ;
-
for (var i:int = 0; i<a.length-1; i++){
-
a[i] = a[i].split(/\(\)|\(|\)/g);
-
var f:String = a[i][0] = a[i][0].replace(/\s/g,"");
-
a[i] = a[i].splice(0, a[i].length - 1);
-
if (a[i].length> 1){
-
a[i] = a[i][1].split(",");
-
a[i].unshift(f);
-
}
-
}
-
return a.splice(0,a.length - 1);
-
}
-
function render(p:Array):void {
-
for (var i:int = 0; i<p.length; i++) {
-
try{
-
graphics[p[i][0]].apply(graphics,p[i].splice(1));
-
}catch(e:Error){};
-
}
-
}
This is a simple idea I had awhile back... This snippet searches twitter for the #asgraph hashtag and if it finds standard Graphics class method calls it renders them.
So if you tweet something likethis :
#asgraph beginFill(0xFF); drawCircle(200,200,10);
it will get rendered into the below swf (you'll need to refresh to see your tweet get rendered):
This movie requires Flash Player 9
Here is a direct link to the swf