By Zevan | January 22, 2009
Actionscript:
-
[SWF(width=600, height=500, backgroundColor=0x000000, frameRate=30)]
-
var points:Array = new Array();
-
var index:int = -1;
-
function polar(thetaInc:Number, radius:Number):Point{
-
index++;
-
if (!points[index]) points[index] = 0;
-
return Point.polar(radius, points[index] += thetaInc);
-
}
-
///////////////////////////////////////////////////
-
// test it out:
-
-
var canvas:BitmapData = new BitmapData(600, 500, false, 0xFFFFFF);
-
-
addChild(new Bitmap(canvas, "auto", true));
-
-
var p0:Point = new Point(80, 100);
-
var p1:Point = new Point(270, 100);
-
var p2:Point = new Point(480, 40);
-
var p3:Point = new Point(170, 180);
-
var p4:Point = new Point(430, 300);
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
for (var i:int= 0; i<100; i++){
-
-
// reset index;
-
index = -1;
-
-
p0 = p0.add(polar(.2, 4).add(polar(-.4,2).add(polar(.05, 1))));
-
canvas.setPixel(p0.x, p0.y, 0x000000);
-
-
p1 = p1.add(polar(.1, 2).add(polar(-.2, 2).add(polar(.03, 1).add(polar(-.01,.5)))));
-
canvas.setPixel(p1.x, p1.y, 0x000000);
-
-
p2 = p2.add(polar(.08, 3 ).add(polar(-.2, -12).add(polar(2, 10))));
-
canvas.setPixel(p2.x, p2.y, 0x000000);
-
-
p3 = p3.add(polar(.08, 7).add(polar(-.2, -12).add(polar(2, 11))));
-
canvas.setPixel(p3.x, p3.y, 0x000000);
-
-
p4 = p4.add(polar(.025, 2).add(polar(-.05,1)));
-
canvas.setPixel(p4.x, p4.y, 0x000000);
-
}
-
}
The polar() function is the real trick here... the rest of the code just uses it to draw this:
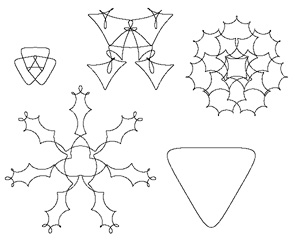
The Point.polar() function is just a conversion from polar to cartesian coords:
Actionscript:
-
x = radius * Math.cos(theta);
-
y = radius * Math.sin(theta);
Also posted in motion | Tagged actionscript, flash |
By Zevan | January 16, 2009
Actionscript:
-
[SWF(width=500, height=500, backgroundColor=0x000000, frameRate=30)]
-
-
// draw an ugly gradient
-
var size:int = 500;
-
var pixNum:int = size * size;
-
var gradient:BitmapData = new BitmapData(size, size, true, 0xFF000000);
-
-
gradient.lock();
-
var xp:int, yp:int;
-
for (var i:int = 0; i<pixNum; i++){
-
xp = i % size;
-
yp = i / size;
-
gradient.setPixel(xp, yp, (yp /= 2) <<16 | (255 - yp) <<8 | (xp / 2) );
-
}
-
gradient.unlock();
-
-
// draw an alphaChannel (radial gradient)
-
var radius:Number = 50;
-
var diameter:Number = radius * 2;
-
-
pixNum = diameter * diameter;
-
-
var brushAlpha = new BitmapData(diameter, diameter, true, 0x00000000);
-
var dx:int, dy:int;
-
var ratio:Number = 255 / radius;
-
var a:int;
-
-
brushAlpha.lock();
-
for (i = 0; i<pixNum; i++){
-
xp = i % diameter;
-
yp = i / diameter;
-
dx = xp - radius;
-
dy = yp - radius;
-
a = int(255 - Math.min(255,Math.sqrt(dx * dx + dy * dy) * ratio));
-
brushAlpha.setPixel32(xp, yp, a <<24);
-
}
-
brushAlpha.unlock();
-
-
// create a black canvas
-
var canvas:BitmapData = new BitmapData(size, size, true, 0xFF000000);
-
addChild(new Bitmap(canvas));
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
// draw the gradient onto the canvas using the alphaChannel (brushAlpha);
-
xp = mouseX - radius;
-
yp = mouseY - radius;
-
canvas.copyPixels(gradient,
-
new Rectangle(xp, yp, diameter, diameter),
-
new Point(xp, yp), brushAlpha, new Point(0,0), true);
-
}
This demo creates an airbrush that paints one BitmapData onto another. This is achieved by using the alpha related arguments of the BitmapData.copyPixels() function. If your not familiar with these you should take some time to play around with them... they're very powerful.
copyPixels() is the fastest way to draw in flash, so if you need speed, copyPixels() is the way to go. It's much faster than using draw().
Also posted in BitmapData | Tagged actionscript, flash |
By Zevan | January 11, 2009
Actionscript:
-
var canvas:BitmapData=new BitmapData(280,280,false,0x000000);
-
addChild(new Bitmap(canvas, PixelSnapping.AUTO, true));
-
var color:uint;
-
// anchor x1, anchor y1,
-
// control-handle x2, control-handle y2,
-
// anchor x3, anchor y3, [resolution incremental value between 0-1]
-
function quadBezier(x1:Number, y1:Number, x2:Number, y2:Number, x3:Number, y3:Number, resolution:Number=.03):void {
-
var b:Number,pre1:Number,pre2:Number,pre3:Number,pre4:Number;
-
for (var a:Number = 0; a <1;a+=resolution) {
-
-
b=1-a;
-
pre1=(a*a);
-
pre2=2*a*b;
-
pre3=(b*b);
-
-
canvas.setPixel(pre1*x1 + pre2*x2 + pre3*x3 ,
-
pre1*y1 + pre2*y2 + pre3*y3, color);
-
}
-
}
-
-
// draw a few
-
color = 0xFFFFFF;
-
-
for (var i:int = 0; i<20; i++){
-
quadBezier(40,100, 150 , 20 + i * 10 , 200, 100,.01);
-
}
-
-
color = 0xFF0000;
-
-
for (i= 0; i<20; i++){
-
quadBezier(150,200, 100 + i * 10, 100 , 120, 30,.01);
-
}
The above demos a function that draws quadratic bezier curves using setPixel().
One of the first posts on this site was a snippet that used setPixel() to draw a cubic bezier curve. I recently needed to do the exact same thing but I wanted to use a quadratic bezier... I knew I had the code laying around somewhere, but I couldn't seem to find it so I just looked on wikipedia and changed the previous cubicBezier() function accordingly.
By Zevan | January 10, 2009
Actionscript:
-
var canvas:BitmapData = new BitmapData(400, 400, false, 0xCCCCCC);
-
addChild(new Bitmap(canvas));
-
-
fillCircle(100,100,50,0xFF0000);
-
-
function fillCircle(xp:Number,yp:Number, radius:Number, col:uint = 0x000000):void {
-
var xoff:int =0;
-
var yoff:int = radius;
-
var balance:int = -radius;
-
-
while (xoff <= yoff) {
-
var p0:int = xp - xoff;
-
var p1:int = xp - yoff;
-
-
var w0:int = xoff + xoff;
-
var w1:int = yoff + yoff;
-
-
hLine(p0, yp + yoff, w0, col);
-
hLine(p0, yp - yoff, w0, col);
-
-
hLine(p1, yp + xoff, w1, col);
-
hLine(p1, yp - xoff, w1, col);
-
-
if ((balance += xoff++ + xoff)>= 0) {
-
balance-=--yoff+yoff;
-
}
-
}
-
}
-
-
function hLine(xp:Number, yp:Number, w:Number, col:uint):void {
-
for (var i:int = 0; i <w; i++){
-
canvas.setPixel(xp + i, yp, col);
-
}
-
}
An implementation of yesterdays post that draws a filled circle instead of an outlined circle.