Actionscript:
-
[SWF(width = 600, height = 600)]
-
var a:Number = 0.02;
-
-
var xn:Object = new Object();
-
-
var scale:Number = 20;
-
var iterations:Number = 10000;
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(600,600,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
a = mouseX / 100;
-
-
// equations from here: http://www.discretedynamics.net/Attractors/Flames.htm
-
// i used object syntax so that I could really reflect the math notation, changing these to
-
// 3 variables will significantly improve performance:
-
xn["n-1"] = mouseX / 200;
-
xn["n"] = mouseY / 200;
-
xn["n+1"] = 0;
-
-
for (var i:int = 0; i<iterations; i++){
-
-
xn["n+1"] = ((a * xn["n"]) / (1 + (xn["n-1"] * xn["n-1"]))) - ((xn["n-1"]) / (1 + (xn["n"] * xn["n"])));
-
-
canvas.setPixel( 280 + xn["n-1"] * scale, 300 + xn["n"] * scale, 0x000000);
-
-
xn["n-1"] = xn["n"];
-
xn["n"] = xn["n+1"];
-
}
-
}
This is an attractor that vaguely resembles flames, I wrote this code so that it would be very similar to the math notation to help anyone who wonders how to go from notation to code. You could replace the Object syntax with 3 separate variables for increased performance.
See the equation here:
http://www.discretedynamics.net/Attractors/Flames.htm
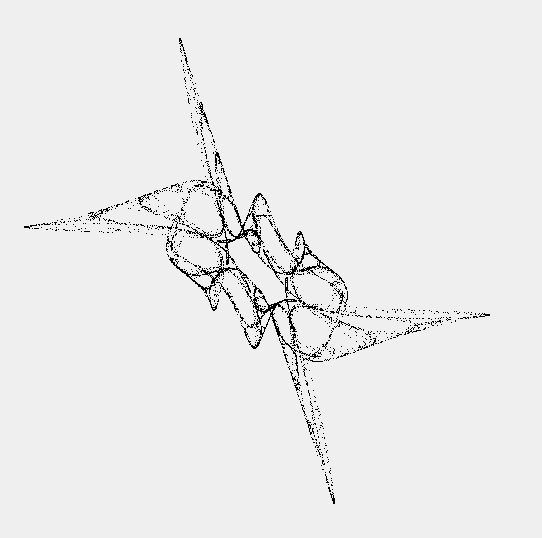
This snippet will create something that looks like this and is reactive to the mouse location
By Zevan | February 16, 2009
Actionscript:
-
[SWF(width=720,height=360,backgroundColor=0x000000,frameRate=30)]
-
-
// ported from here:
-
//http://www.cs.rit.edu/~ncs/color/t_convert.html
-
-
function hsv(h:Number, s:Number, v:Number):Array{
-
var r:Number, g:Number, b:Number;
-
var i:int;
-
var f:Number, p:Number, q:Number, t:Number;
-
-
if (s == 0){
-
r = g = b = v;
-
return [Math.round(r * 255), Math.round(g * 255), Math.round(b * 255)];
-
}
-
-
h /= 60;
-
i = Math.floor(h);
-
f = h - i;
-
p = v * (1 - s);
-
q = v * (1 - s * f);
-
t = v * (1 - s * (1 - f));
-
-
switch( i ) {
-
case 0:
-
r = v;
-
g = t;
-
b = p;
-
break;
-
case 1:
-
r = q;
-
g = v;
-
b = p;
-
break;
-
case 2:
-
r = p;
-
g = v;
-
b = t;
-
break;
-
case 3:
-
r = p;
-
g = q;
-
b = v;
-
break;
-
case 4:
-
r = t;
-
g = p;
-
b = v;
-
break;
-
default: // case 5:
-
r = v;
-
g = p;
-
b = q;
-
break;
-
}
-
return [Math.round(r * 255), Math.round(g * 255), Math.round(b * 255)];
-
}
-
-
-
//
-
// -- test it out by drawing a few things
-
//
-
-
var canvas:BitmapData = new BitmapData(720, 360, false, 0x000000);
-
-
addChild(new Bitmap(canvas));
-
-
canvas.lock();
-
var size:int = canvas.width * canvas.height;
-
var xp:int, yp:int, c:Array, i:int;
-
-
for (i = 0; i<size; i++){
-
xp = i % 360;
-
yp = i / 360;
-
c = hsv(xp, 1, yp / 360);
-
canvas.setPixel(xp, yp, c[0] <<16 | c[1] <<8 | c[2]);
-
}
-
-
var dx:Number, dy:Number, dist:Number, ang:Number;
-
-
for (i = 0; i<size; i++){
-
xp = i % 360;
-
yp = i / 360;
-
dx = xp - 180;
-
dy = yp - 180;
-
dist = 1 - Math.sqrt((dx * dx) + (dy * dy)) / 360;
-
ang = Math.atan2(dy, dx) / Math.PI * 180;
-
if (ang <0){
-
ang += 360;
-
}
-
c = hsv(ang, 1, dist);
-
canvas.setPixel(360 + xp, yp, c[0] <<16 | c[1] <<8 | c[2]);
-
}
-
canvas.unlock();
This is one of those things I've been meaning to play with for awhile. The above demos a function called hsv() which takes 3 arguments: angle (0-360), saturation(0-1) and value(0-1). The function returns an array of rgb values each with a range of (0-255).
There's some room for optimization here, but for clarity I left as is. Even just playing with HSV (also know as HSB) for a few minutes, I see some interesting potential for dynamically generating color palettes for generative style experiments.
I looked around for the most elegant looking code snippet to port in order to write this... I eventually stumbled upon this great resource.
If you test the above on your timeline it will generate this image:
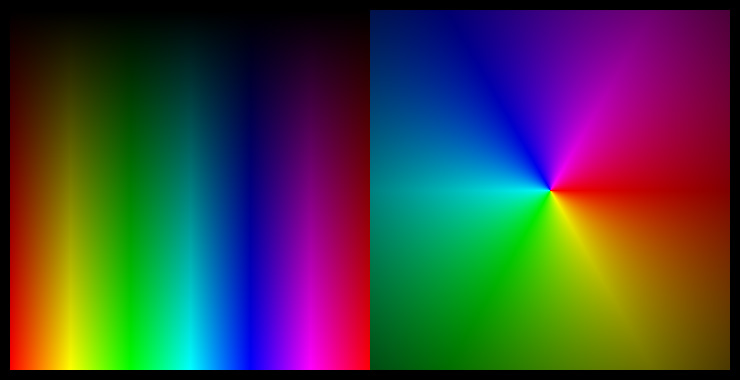
I usually only post one snippet a day... not sure why I decided to post two today.
By Zevan | February 4, 2009
Actionscript:
-
[SWF(width=900,height=390,backgroundColor=0x000000,frameRate=30)]
-
-
var canvas:BitmapData=new BitmapData(4,8,false,0xFFFFFFFF);
-
var pixNum:int = canvas.width * canvas.height;
-
-
var frame:Bitmap = Bitmap(addChild(new Bitmap(canvas)));
-
frame.scaleX = frame.scaleY = canvas.width * 10;
-
frame.x = stage.stageWidth / 2 - frame.width / 2;
-
frame.y =20;
-
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.autoSize = TextFieldAutoSize.LEFT;
-
txt.defaultTextFormat = new TextFormat("Verdana", 8, 0xFFFFFF);
-
txt.x = frame.x - 3;
-
txt.y = frame.y + frame.height + 10;
-
-
var s:String, a:Number = 0, d:Number = 0;
-
var r:Number = 0xFFFFFFFF / (stage.stageWidth-20) ;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
a += (d - a) / 8;
-
-
d = (mouseX-10) * r;
-
-
s = Math.max(0,Math.min(0xFFFFFFFF, a)).toString(2);
-
-
if (s.length <pixNum){
-
while(s.length-1 <pixNum){
-
s = "0" + s;
-
}
-
}
-
-
txt.text = s;
-
-
canvas.lock();
-
canvas.fillRect(canvas.rect, 0xFFFFFF);
-
for (var i:int = 0; i<pixNum; i++){
-
if (s.charAt(i) == "0"){
-
canvas.setPixel(i % 4, i / 4, 0x000000);
-
}
-
}
-
canvas.unlock();
-
}
Similar to yesterdays post... this snippet visualizes binary numbers. Move your mouse left and right to change the value of the number that's being displayed.
Here are a few stills:
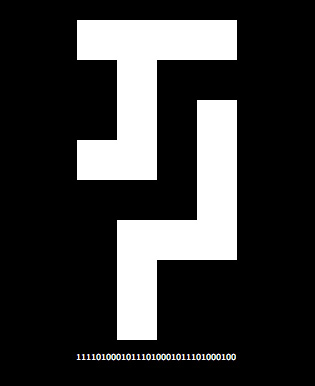
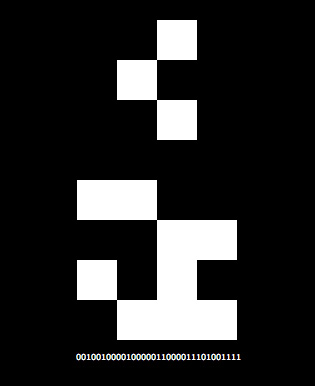
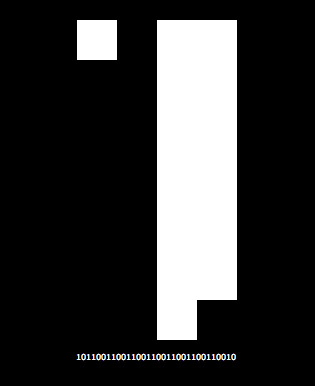
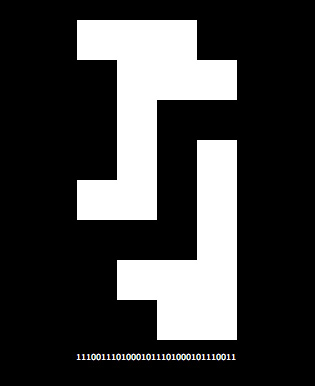
This code uses the mouse position to choose which binary number to display. Going all the way to the left of the screen will set this number to 0 and going all the way to the right will set it to 4294967295... that number may look arbitrary unless you see it in in binary 11111111111111111111111111111111 or in hexadecimal 0xFFFFFFFF.
Also posted in BitmapData | Tagged actionscript, flash |
By Zevan | January 23, 2009
Actionscript:
-
[SWF(width=700, height=400, backgroundColor=0x000000, frameRate=30)]
-
-
var points:Array = new Array();
-
var index:int = -1;
-
function polar(thetaInc:Number, radius:Number):Point{
-
index++;
-
if (!points[index]) points[index] = 0;
-
return Point.polar(radius, points[index] += thetaInc)
-
}
-
///////////////////////////////////////////////////
-
// test it out:
-
-
var canvas:BitmapData = new BitmapData(700, 400, false, 0xFFFFFF);
-
-
addChild(new Bitmap(canvas, "auto", true));
-
-
var p0:Point = new Point(200, 200);
-
var p1:Point = new Point(500, 200);
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
var rad:Point = polar(.05, 4);
-
-
for (var i:int= 0; i<100; i++){
-
-
// reset index;
-
index = -1;
-
-
p0 = p0.add(polar(.025, 2).add(polar(-.05,rad.x)));
-
canvas.setPixel(p0.x, p0.y, 0x000000);
-
-
p1 = p1.add(polar(.025, 2).add(polar(-.05,rad.x).add(polar(.1, rad.y))));
-
canvas.setPixel(p1.x, p1.y, 0x000000);
-
}
-
}
This is pretty much the same as yesterdays... I just changed the way I use the polar() function to draw two more shapes:
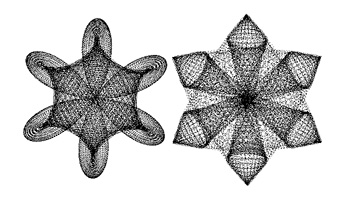