Actionscript:
-
var xp:Number = 0;
-
var yp:Number = 0;
-
var t:Number = 0;
-
var r:Number = 200;
-
x = stage.stageWidth / 2;
-
y = stage.stageHeight / 2;
-
-
graphics.lineStyle(0,0x000000);
-
addEventListener(Event.ENTER_FRAME, onRun);
-
function onRun(evt:Event):void {
-
r = 200 * Math.cos(t / 10);
-
xp = r * Math.pow(Math.cos(t), 3);
-
yp = r * Math.pow(Math.sin(t), 3);
-
if (t == 0){
-
graphics.moveTo(xp, yp);
-
}else{
-
graphics.lineTo(xp, yp);
-
}
-
t += 0.1;
-
}
While browsing mathworld I decided to do a variation on this curve . The above snippet will draw something like this:
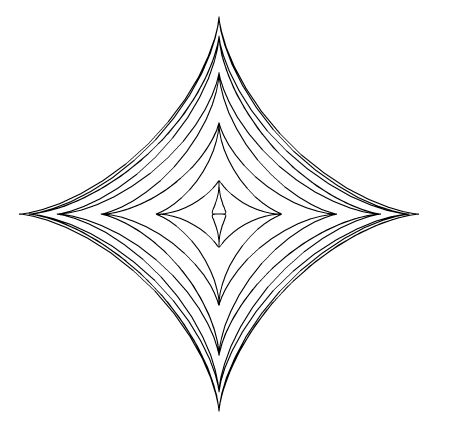
Posted in Math, misc | Also tagged as3, flash |
Actionscript:
-
[SWF(width = 500, height=500)]
-
var ring:MovieClip = createRing();
-
ring.x = stage.stageWidth / 2;
-
ring.y = stage.stageHeight / 2;
-
addChild(ring);
-
-
function createRing(sectionNum:int = 30):MovieClip{
-
var container:MovieClip = new MovieClip();
-
container.circles = [];
-
container.theta = 0;
-
container.thetaDest = 0;
-
var step:Number = (Math.PI * 2) / sectionNum;
-
for (var i:int = 0; i<sectionNum; i++){
-
var c:MovieClip = new MovieClip();
-
with (c.graphics){
-
lineStyle(0,0x000000);
-
beginFill(0xCCCCCC);
-
drawCircle(0,0,20);
-
}
-
c.thetaOffset = step * i;
-
container.addChild(c);
-
container.circles.push(c);
-
}
-
container.addEventListener(Event.ENTER_FRAME, onRun);
-
return container;
-
}
-
function onRun(evt:Event):void {
-
var container:MovieClip = MovieClip(evt.currentTarget);
-
var num:int = container.circles.length;
-
for (var i:int = 0; i<num; i++){
-
var c:MovieClip = container.circles[i];
-
var angle:Number = container.theta + c.thetaOffset;
-
c.x = 200 * Math.cos(angle);
-
c.y = 100 * Math.sin(angle);
-
c.scaleX = (100 + c.y) / 120 + 0.2;
-
c.scaleY = c.scaleX;
-
}
-
container.circles.sortOn("y", Array.NUMERIC);
-
for (i = 0; i<num; i++){
-
container.addChild(container.circles[i]);
-
}
-
if (container.mouseX <-100){
-
container.thetaDest -= 0.05;
-
}
-
if (container.mouseX> 100){
-
container.thetaDest += 0.05;
-
}
-
container.theta += (container.thetaDest - container.theta) / 12;
-
-
}
This snippet shows how to create a 3D ring navigation using sine and cosine. Have a look:
Actionscript:
-
makeFlyer();
-
-
function makeFlyer():void{
-
var thing:MovieClip = new MovieClip();
-
thing.x = 200;
-
thing.y = 200;
-
-
addChild(thing);
-
-
var prop:Shape = new Shape();
-
with (prop.graphics){
-
lineStyle(0,0x000000);
-
beginFill(0x000000);
-
moveTo(-100,0);
-
curveTo(-100, -30, 0, 0);
-
curveTo(100, 30, 100, 0);
-
curveTo(100, -30, 0, 0);
-
curveTo(-100, 30, -100, 0);
-
}
-
prop.scaleX = prop.scaleY = 0.5;
-
var container:MovieClip = new MovieClip();
-
//container.x = -50;
-
container.addChild(prop);
-
container.scaleY = 0.6;
-
thing.addChild(container);
-
-
var body:Shape = new Shape();
-
with (body.graphics){
-
lineStyle(0, 0x000000);
-
beginFill(0x000000);
-
lineTo(0,80);
-
drawCircle(0,80,10);
-
}
-
thing.addChild(body);
-
thing.velX = 0;
-
thing.velY = 0;
-
thing.posX = thing.x;
-
thing.posY = thing.y;
-
thing.theta = 0;
-
thing.prop = prop;
-
thing.addEventListener(Event.ENTER_FRAME, onRun);
-
}
-
function onRun(evt:Event):void{
-
var t:MovieClip = MovieClip(evt.currentTarget);
-
t.prop.rotation += 10
-
t.velY = 3 * Math.cos(t.theta);
-
t.velX = 3 * Math.sin(t.theta / 2);
-
t.theta += 0.05
-
t.posX += t.velX;
-
t.posY += t.velY;
-
-
t.x = t.posX;
-
t.y = t.posY;
-
}
This snippet creates a small flying object that moves with sine and cosine.
Have a look at the swf...
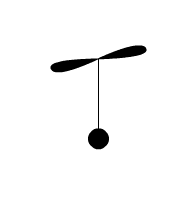
Posted in motion | Also tagged as3, flash |
By Zevan | April 30, 2010
So there are 434 posts on this site to date. I hope to keep posting but it isn't always easy to come up with new ideas. Another project I've been working on is a series of drawings and interactive animations over at my other website (shapevent). I've been creating entries for this part of shapevent pretty regularly - go have a look:
http://www.shapevent.com/log/
Posted in Announcements, projects | Also tagged as3, flash |