Actionscript:
-
[SWF(width = 600, height = 500, backgroundColor=0xFFFFFF)]
-
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight,false, 0xFFFFFF);
-
addChild(new Bitmap(canvas));
-
-
var matrix:Matrix = new Matrix();
-
var grid:Sprite = new Sprite();
-
grid.filters = [new DropShadowFilter(4, 0, 0, 0.05, 20, 10)];
-
matrix.rotate(Math.PI / 4);
-
matrix.scale(1, 0.6);
-
matrix.translate(stage.stageWidth / 2, stage.stageHeight / 2 + 50);
-
grid.transform.matrix = matrix;
-
-
var rowCol:int = 9;
-
var spikeNum:Number = rowCol * rowCol;
-
var diameter:Number = 30;
-
var space:Number = diameter + 10;
-
var halfGridSize:Number = rowCol * space / 2;
-
var radius:Number;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
radius = diameter / 2;
-
grid.y -= 0.9;
-
grid.graphics.clear();
-
for (var i:Number = 0; i<spikeNum; i++){
-
var xp:Number = i % rowCol;
-
var yp:Number = int(i / rowCol);
-
drawSpike(xp * space - halfGridSize, yp * space - halfGridSize, (xp + yp) / 4, 0, -yp);
-
}
-
canvas.draw(grid, grid.transform.matrix);
-
diameter -= 0.5;
-
if (diameter <3){
-
removeEventListener(Event.ENTER_FRAME, onLoop);
-
}
-
}
-
function drawSpike(xp:Number, yp:Number, rot:Number = 0, xOff:Number=0, yOff:Number = 0):void{
-
matrix.createGradientBox(diameter, diameter, rot, xp - radius + yOff, yp - radius + xOff);
-
with (grid.graphics){
-
beginGradientFill(GradientType.LINEAR, [0xFFFFFF, 0x999999], [1,1], [0, 255], matrix, SpreadMethod.PAD);
-
drawCircle(xp, yp, radius);
-
}
-
}
This snippet draws some isometric cones. Here are some images created by tweaking a few of the values:
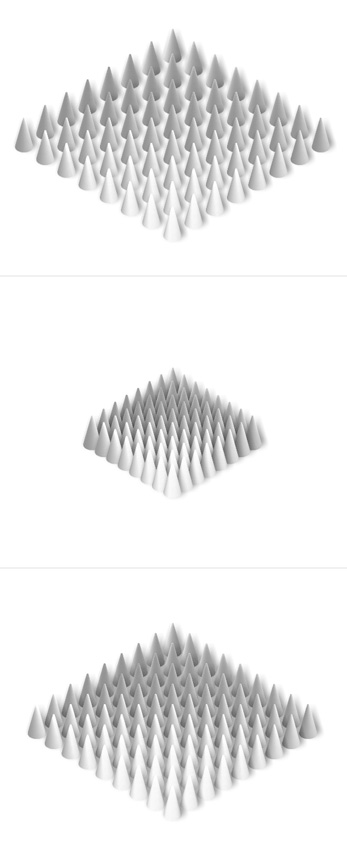
Actionscript:
-
var grid:Sprite = Sprite(addChild(new Sprite()));
-
var matrix:Matrix = new Matrix();
-
// make the grid sprite look isometric using the transform.matrix property
-
matrix.rotate(Math.PI / 4);
-
matrix.scale(1, 0.5);
-
matrix.translate(stage.stageWidth / 2, stage.stageHeight / 2);
-
grid.transform.matrix = matrix;
-
-
// draw a grid of circles to show that it does in fact look isometric
-
var rowCol:Number = 8;
-
var num:Number = rowCol * rowCol;
-
-
var diameter:Number = 40;
-
var radius:Number = diameter / 2;
-
var space:Number = diameter + 10;
-
var halfGridSize:Number = rowCol * space / 2;
-
-
grid.graphics.beginFill(0xFF0000);
-
for (var i:int = 0; i<num; i++){
-
grid.graphics.drawCircle(i % 8 * space - halfGridSize, int(i / 8) * space - halfGridSize, radius);
-
}
This snippet shows how to use transform.matrix to make a DisplayObject look isometric. This can also be achieved with nesting.
In the case of this grid of red circles, we rotate it 45 degrees, scale it 50% on the y and move it to the center of the stage (lines 4-6).
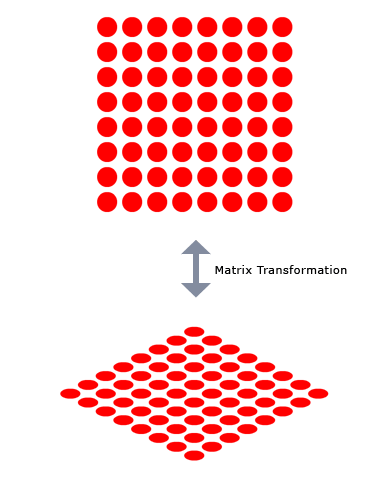
Didn't get a chance to post today but will do two posts tomorrow... been looking into things related to convex and concave functions... here are some links related to that topic:
http://en.wikipedia.org/wiki/Convex_function
http://www.economics.utoronto.ca/osborne/MathTutorial/CVNF.HTM
Will probably have at least two snippets related to this in the near future...
Actionscript:
-
[SWF(width = 628, height=500)]
-
var trans:Matrix = new Matrix();
-
var pnt:Point = new Point();
-
x = stage.stageWidth / 2;
-
y = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
graphics.clear();
-
graphics.lineStyle(10, 0x000000, 1, false, LineScaleMode.NORMAL, CapsStyle.SQUARE);
-
pnt.x = 0;
-
pnt.y = 0;
-
trans.identity();
-
trans.translate(15,15);
-
trans.rotate(mouseX/10 * Math.PI / 180);
-
graphics.moveTo(pnt.x, pnt.y);
-
for (var i:int = 0; i<12; i++){
-
pnt = trans.transformPoint(pnt);
-
graphics.lineTo(pnt.x, pnt.y);
-
}
-
}
I was thinking about processing and OpenGL today... I always really enjoyed using matrix stuff, working with the matrix stack, using push() and pop() etc... Understanding how to use matrices is pretty important when your doing advanced graphics in actionscript. If you haven't messed with matrices, this snippet is a good place to start. It uses Matrix.transformPoint() to draw a series of connected lines that are controlled by the x location of the mouse. Back before flash 8, this kind of thing was usually done using trig or MovieClip nesting...
I posted another snippet on this topic awhile back.
Posted in matrix, motion |