By Zevan | April 12, 2009
Actionscript:
-
var matrix:Matrix3D = new Matrix3D();
-
-
const PARTICLE_NUM:int = 100000;
-
var verts:Vector.<Number> = new Vector.<Number>();
-
var pVerts:Vector.<Number> = new Vector.<Number>();
-
var uvts:Vector.<Number> = new Vector.<Number>();
-
-
for (var i:int = 0; i<PARTICLE_NUM; i++){
-
verts.push(Math.random()*250 - 125);
-
verts.push(Math.random()*250 - 125);
-
verts.push(Math.random()*250 - 125);
-
-
pVerts.push(0), pVerts.push(0);
-
uvts.push(0), uvts.push(0), uvts.push(0);
-
}
-
-
var canvas:BitmapData = new BitmapData(400,400,false, 0x000000);
-
addChild(new Bitmap(canvas));
-
var dx:Number=0;
-
var dy:Number=0;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
dx += (mouseX - dx)/4;
-
dy += (mouseY - dy)/4;
-
-
matrix.identity();
-
matrix.appendRotation(dy,Vector3D.X_AXIS);
-
matrix.appendRotation(dx,Vector3D.Y_AXIS);
-
matrix.appendTranslation(200, 200, 0);
-
-
Utils3D.projectVectors(matrix, verts, pVerts, uvts);
-
-
canvas.lock();
-
canvas.fillRect(canvas.rect, 0x000000);
-
var leng:int = pVerts.length;
-
for (var i:int = 0; i<leng; i+=2){
-
canvas.setPixel( pVerts[i], pVerts[i + 1], 0xFFFFFF);
-
}
-
canvas.unlock();
-
}
The above shows an easy way to use Utils3D.projectVectors() to move some pixels around in 3D. Since the 3D math is done behind the scenes by the flash player it runs quite fast...
By Zevan | April 11, 2009
Actionscript:
-
var a:Vector.<Sprite> = new Vector.<Sprite>();
-
-
trace("unsorted");
-
for (var i:int = 0; i<10; i++){
-
var s:Sprite = new Sprite();
-
s.x = int(Math.random()*100);
-
a.push(s);
-
trace(s.x);
-
}
-
-
quickSortOn(a, "x", 0, a.length-1);
-
-
trace("sorted");
-
for (i= 0; i<10; i++){
-
trace(a[i].x);
-
}
-
-
// modified code from kirupa.com
-
// http://www.kirupa.com/developer/actionscript/quickSort.htm
-
function quickSortOn(a:Vector.<Sprite>, prop:String, left:int, right:int):void {
-
var i:int = 0, j:int = 0, pivot:Sprite, tmp:Sprite;
-
i=left;
-
j=right;
-
pivot = a[Math.round((left+right)*.5)];
-
while (i<=j) {
-
while (a[i][prop]<pivot[prop]) i++;
-
while (a[j][prop]>pivot[prop]) j--;
-
if (i<=j) {
-
tmp=a[i];
-
a[i]=a[j];
-
i++;
-
a[j]=tmp;
-
j--;
-
}
-
}
-
if (left<j) quickSortOn(a, prop, left, j);
-
if (i<right) quickSortOn(a, prop, i, right);
-
}
-
/* outputs something like:
-
unsorted
-
26
-
33
-
20
-
63
-
7
-
68
-
75
-
39
-
67
-
53
-
sorted
-
7
-
20
-
26
-
33
-
39
-
53
-
63
-
67
-
68
-
75
-
*/
This demo is my first quick stab at using at a sortOn() function for the Vector class. It sorts a Vector of Sprites by their x property.
Recently there were a few times when I was prototyping ideas and suddenly realized that I needed to change my Vector to an Array because I needed to use sortOn().(If you don't already know, there is no built in sortOn() method for the Vector class). In the past I spent some time with sorting algorithms, bubble, insertion etc... so I knew I could pretty easily write my own sortOn(), but I also realized that a generic implementation wouldn't be easy/possible without loosing the type of the Vector. What I mean is, if you have a Vector of Sprites, you need a sorting method that takes a Vector.< Sprite > type as an argument (as seen above), if you have a Vector of TextFields you need a Vector.< TextField > type as an argument. You could of course use a generic type, but this kind of defeats the purpose of using a vector in the first place...
I will likely post a revised version of this in the near future with a slightly improved implementation of QuickSort. I haven't spent that much time with this, but If I recall correctly this is not the ideal implementation. I ported this code from a nice Kirupa tutorial and modified it to sort based on a property...
By Zevan | April 10, 2009
Actionscript:
-
var xn:Number = 1;
-
var xn1:Number = 0
-
var square:Number =39;
-
-
trace("find the sqrt of ", square);
-
trace("Math.sqrt: ", Math.sqrt(square))
-
-
// no starting approximation, just try up to 35 iterations
-
for(var i:int = 0; i<35; i++){
-
xn1 = .5 * (xn + square / xn);
-
if (xn1== xn){
-
trace("other sqrt: ", xn1);
-
break;
-
}
-
xn = xn1;
-
}
-
/*outputs
-
find the sqrt of 39
-
Math.sqrt: 6.244997998398398
-
other sqrt: 6.244997998398398
-
*/
I was reading about calculating square roots - not for speed optimization purposes, just to see some of the different ways to go about it. The above snippet uses the Babylonian method to find the square root of a number. I left out the first step of guessing at the square root... so this snippet is by no means efficient...
Posted in Math | Tagged actionscript, flash |
Actionscript:
-
// Superformula (equations from):
-
// http://www.geniaal.be/downloads/AMJBOT.pdf
-
// http://en.wikipedia.org/wiki/Superformula
-
const TWO_PI:Number = Math.PI * 2;
-
function superShape(a:Number, b:Number, m:Number, n1:Number, n2:Number, n3:Number, pnt:Point, scale:Number):void{
-
var r:Number = 0
-
var p:Number = 0;
-
var xp:Number = 0, yp:Number = 0;
-
while(p <= TWO_PI){
-
var ang:Number = m * p / 4;
-
with(Math){
-
r = pow(pow(abs(cos(ang) / a), n2) + pow(abs(sin(ang) / b), n3),-1/n1);
-
xp = r * cos(p);
-
yp = r * sin(p);
-
}
-
p += .01;
-
canvas.setPixel(pnt.x + xp *scale, pnt.y + yp * scale, 0xFFFFFF);
-
}
-
}
-
// test it out:
-
var canvas:BitmapData = new BitmapData(700,600,false, 0x000000);
-
addChild(new Bitmap(canvas, "auto", true));
-
-
superShape(1, 1, 5, 23, 23, 23, new Point(100,80), 30);
-
superShape(1, 1, 5, 13, 13, 3, new Point(200,80), 30);
-
superShape(1, 1, 8, 3, 13, 3, new Point(300,80), 30);
-
superShape(10,8, 16, 30, 13, 3, new Point(450,80), 30);
-
superShape(1,1, 1, .5, .5, .5, new Point(100,190), 100);
-
-
for (var i:int = 0; i <150; i++){
-
superShape(1,1, 2, 1+i/800, 4, 8-i * .1, new Point(550,350), 50);
-
}
-
for (i = 0; i <20; i++){
-
superShape(1.1,1.2, 6, 2 + i , 4, 9 - i, new Point(200,350), 50);
-
}
The above snippet demos a function that will draw Supershapes using the Superformula...
From wikipedia:
The Superformula appeared in a work by Johan Gielis. It was obtained by generalizing the superellipse, named and popularized by Piet Hein...
Here is the result of the above code:
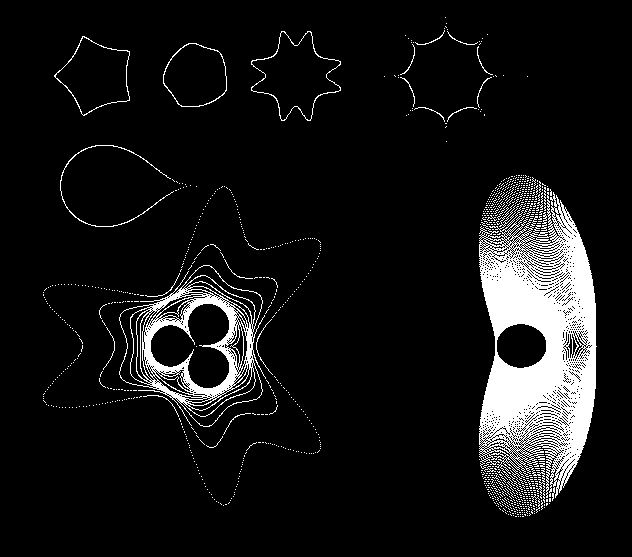
You can read more about the Superformula here in the original paper by Gielis.
wikipedia entry...
3d Supershapes by Paul Bourke