Actionscript:
-
trace(formatNum(1000));
-
trace(formatNum(10000000));
-
trace(formatNum(1000000.39485));
-
-
function formatNum(num:Number):String {
-
var newStr:String = "";
-
var str:String = num.toString();
-
-
var parts:Array = str.split(".");
-
str = parts[0];
-
var end:String = (parts[1]) ? "." + parts[1] : "";
-
-
var i:int = str.length;
-
while(i--> 0){
-
var char:String = str.charAt(i);
-
if ((str.length - i) % 3 == 0){
-
newStr = "," + char +newStr;
-
}else{
-
newStr = char + newStr;
-
}
-
}
-
return newStr + end;
-
}
-
/*
-
outputs:
-
1,000
-
10,000,000
-
1,000,000.39485
-
*/
The function formatNum() returns a string given a number argument ... it adds commas where appropriate and takes into account decimal values. This is useful anywhere you need to display numbers over 1,000, in a game, in a calculator app etc...
Actionscript:
-
[SWF(width = 600, height = 600)]
-
var a:Number = 0.02;
-
var b:Number = .9998;
-
-
var xn1:Number = 5;
-
var yn1:Number = 0;
-
var xn:Number, yn:Number;
-
-
var scale:Number = 10;
-
var iterations:Number = 20000;
-
-
function f(x:Number):Number{
-
var x2:Number = x * x;
-
return a * x + (2 * (1 - a) * x2) / (1 + x2);
-
}
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(600,600,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
a = mouseY / 1000;
-
xn1 = mouseX / 30;
-
yn1 = 0;
-
for (var i:int = 0; i<iterations; i++){
-
xn = xn1;
-
yn = yn1;
-
-
xn1 = b * yn + f(xn);
-
yn1 = -xn + f(xn1);
-
canvas.setPixel( 280 + xn1 * scale, 300 + yn1 * scale, 0x000000);
-
}
-
}
Notice the setup for this is very similar to yesterdays flames attractor post.
Back in october of last year I stumbled upon the excellent subblue website by Tom Beddard. I was REALLY impressed by a blog post about Gumowski / Mira patterns. If you haven't seen it you should go take a look.
I'd never heard of Gumowski / Mira patterns before and made a mental note to go and try to read about them and maybe find an equation to port to actionscript or processing. Anyway, a few days ago I decided to go ahead and look up the math and... this is the result.
I got equation over at mathworld...
For simplicity I intentionally made the actionscript code look as much like the mathworld equation as possible. Using f for my function name and using xn1, yn1 etc... There are a few speed optimizations that could be made but I wanted this snippet to be very readable.
Here are a few examples of what this code will generate:
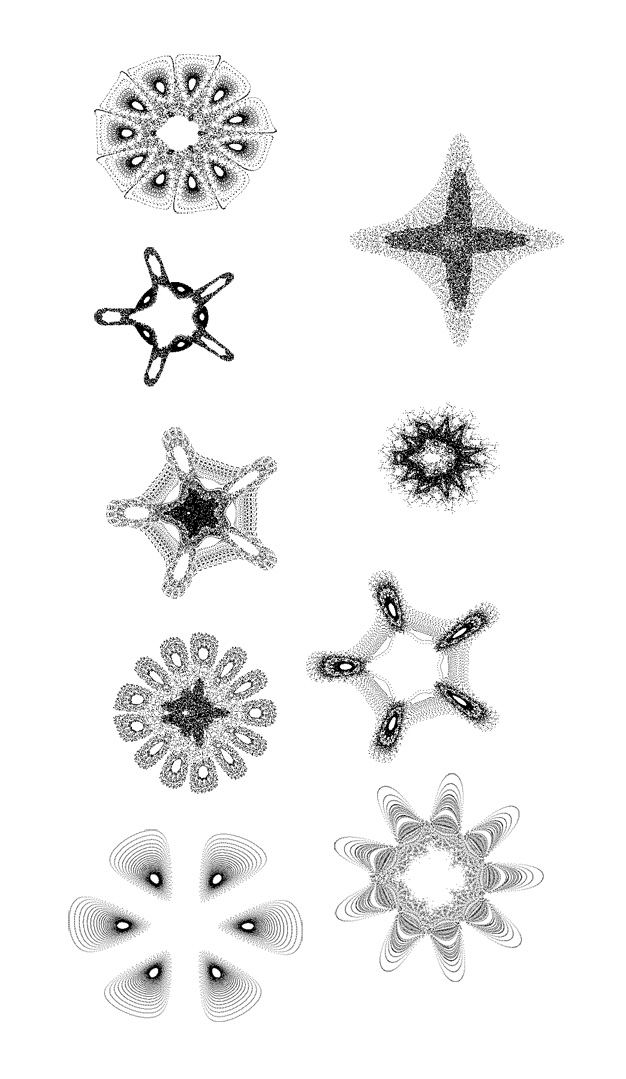
Actionscript:
-
[SWF(width = 600, height = 600)]
-
var a:Number = 0.02;
-
-
var xn:Object = new Object();
-
-
var scale:Number = 20;
-
var iterations:Number = 10000;
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(600,600,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
a = mouseX / 100;
-
-
// equations from here: http://www.discretedynamics.net/Attractors/Flames.htm
-
// i used object syntax so that I could really reflect the math notation, changing these to
-
// 3 variables will significantly improve performance:
-
xn["n-1"] = mouseX / 200;
-
xn["n"] = mouseY / 200;
-
xn["n+1"] = 0;
-
-
for (var i:int = 0; i<iterations; i++){
-
-
xn["n+1"] = ((a * xn["n"]) / (1 + (xn["n-1"] * xn["n-1"]))) - ((xn["n-1"]) / (1 + (xn["n"] * xn["n"])));
-
-
canvas.setPixel( 280 + xn["n-1"] * scale, 300 + xn["n"] * scale, 0x000000);
-
-
xn["n-1"] = xn["n"];
-
xn["n"] = xn["n+1"];
-
}
-
}
This is an attractor that vaguely resembles flames, I wrote this code so that it would be very similar to the math notation to help anyone who wonders how to go from notation to code. You could replace the Object syntax with 3 separate variables for increased performance.
See the equation here:
http://www.discretedynamics.net/Attractors/Flames.htm
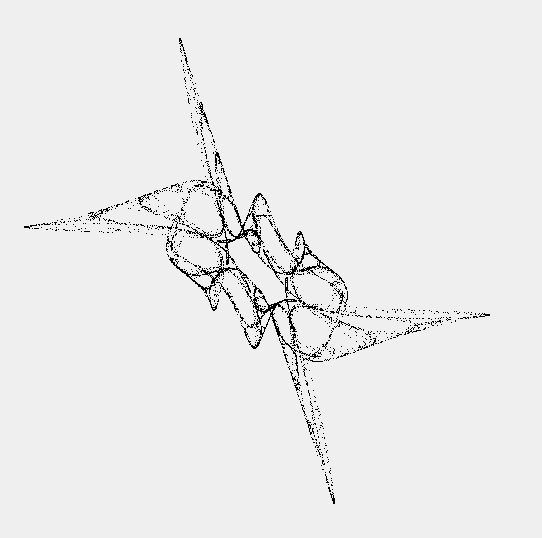
This snippet will create something that looks like this and is reactive to the mouse location
Actionscript:
-
[SWF(backgroundColor=0x000000, frameRate=30)]
-
-
var elements:Array = new Array();
-
for (var i:int = 0; i<200; i++){
-
var c:MovieClip= MovieClip(addChild(new MovieClip()));
-
c.x = Math.random()*(stage.stageWidth + 100) - 50;
-
c.y = Math.random()*stage.stageHeight;
-
with(c.graphics) lineStyle(2, 0xFFFFFF,3), drawCircle(0,0,2 + c.y / 20);
-
c.startX = c.x;
-
elements.push(c);
-
}
-
-
var offset:Number = 0;
-
var t:Number = 0;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
t+=.1;
-
offset = 200 * Math.cos(t);
-
for (var i:int = 0; i<elements.length; i++){
-
elements[i].x = elements[i].startX + offset / ((stage.stageWidth - elements[i].y) / 80);
-
}
-
}
I wrote this snippet originally to automatically add parallax motion to a bunch of quick drawings I did within the flash IDE. Each movieClip in the elements array is moved from left to right based on it's y position. Clips with a higher y value will oscillate more from left to right than clips with lower y values.
Here is the original drawing I used this on.
And here is what the above snippet will create.
Posted in motion | Tagged actionscript, flash |