By Zevan | February 4, 2009
Actionscript:
-
[SWF(width=900,height=390,backgroundColor=0x000000,frameRate=30)]
-
-
var canvas:BitmapData=new BitmapData(4,8,false,0xFFFFFFFF);
-
var pixNum:int = canvas.width * canvas.height;
-
-
var frame:Bitmap = Bitmap(addChild(new Bitmap(canvas)));
-
frame.scaleX = frame.scaleY = canvas.width * 10;
-
frame.x = stage.stageWidth / 2 - frame.width / 2;
-
frame.y =20;
-
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.autoSize = TextFieldAutoSize.LEFT;
-
txt.defaultTextFormat = new TextFormat("Verdana", 8, 0xFFFFFF);
-
txt.x = frame.x - 3;
-
txt.y = frame.y + frame.height + 10;
-
-
var s:String, a:Number = 0, d:Number = 0;
-
var r:Number = 0xFFFFFFFF / (stage.stageWidth-20) ;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
a += (d - a) / 8;
-
-
d = (mouseX-10) * r;
-
-
s = Math.max(0,Math.min(0xFFFFFFFF, a)).toString(2);
-
-
if (s.length <pixNum){
-
while(s.length-1 <pixNum){
-
s = "0" + s;
-
}
-
}
-
-
txt.text = s;
-
-
canvas.lock();
-
canvas.fillRect(canvas.rect, 0xFFFFFF);
-
for (var i:int = 0; i<pixNum; i++){
-
if (s.charAt(i) == "0"){
-
canvas.setPixel(i % 4, i / 4, 0x000000);
-
}
-
}
-
canvas.unlock();
-
}
Similar to yesterdays post... this snippet visualizes binary numbers. Move your mouse left and right to change the value of the number that's being displayed.
Here are a few stills:
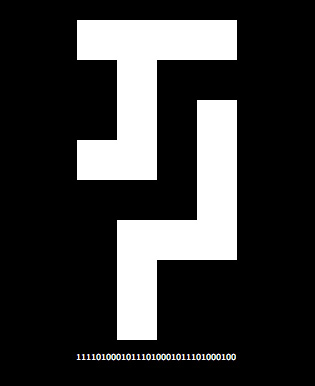
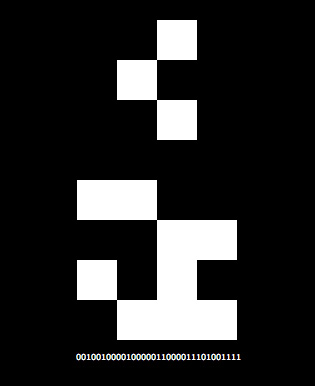
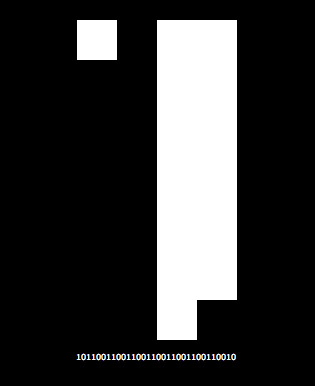
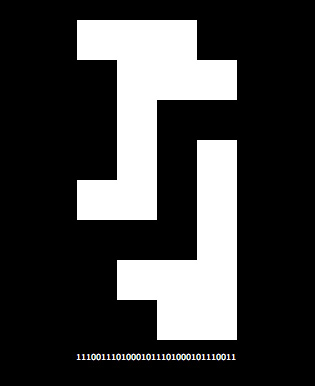
This code uses the mouse position to choose which binary number to display. Going all the way to the left of the screen will set this number to 0 and going all the way to the right will set it to 4294967295... that number may look arbitrary unless you see it in in binary 11111111111111111111111111111111 or in hexadecimal 0xFFFFFFFF.
By Zevan | February 3, 2009
Actionscript:
-
[SWF(width=320,height=512,backgroundColor=0x000000,frameRate=30)]
-
-
var canvas:BitmapData=new BitmapData(32,512,false,0xFFFFFF);
-
addChild(new Bitmap(canvas)).scaleX = 10;
-
-
var a:uint ;
-
var s:String;
-
var m:Number = 0;
-
var d:Number = 0;
-
var mi:int ;
-
var r:Number = 0xFFFFFF / stage.stageWidth;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function onLoop(evt:Event):void {
-
-
d = mouseX * r;
-
m += (d - m) / 30;
-
-
mi = int(m);
-
-
canvas.lock();
-
canvas.fillRect(canvas.rect, 0xFFFFFF);
-
-
a = 0xFFFFFFFF;
-
for (var i:int = 0; i<512; i++) {
-
s = (a -= mi).toString(2);
-
for (var j:int = 0; j<s.length; j++) {
-
if (s.charAt(j)=="0") {
-
canvas.setPixel(j, i, 0x000000);
-
}
-
}
-
}
-
canvas.unlock();
-
}
The above uses setPixel() to visualize numbers in binary format. You can move your mouse left and right to change an incremental counting value....
Here's a still generated by this snippet:
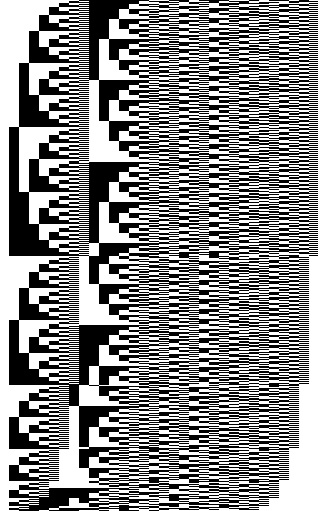
By Zevan | February 2, 2009
Actionscript:
-
package {
-
import adobe.utils.*;
-
import flash.accessibility.*;
-
import flash.display.*;
-
import flash.errors.*;
-
import flash.events.*;
-
import flash.external.*;
-
import flash.filters.*;
-
import flash.geom.*;
-
import flash.media.*;
-
import flash.net.*;
-
import flash.printing.*;
-
import flash.profiler.*;
-
import flash.sampler.*;
-
import flash.system.*;
-
import flash.text.*;
-
import flash.ui.*;
-
import flash.utils.*;
-
import flash.xml.*;
-
-
dynamic public class Snippet extends MovieClip {
-
public function Snippet() {
-
// paste your snippet here (functions and all)
-
}
-
}
-
}
This snippet imports all flash packages and is dynamic... you can copy actionsnippet code into the constructor of this file if you use Flex, FlashDevelop, TextMate etc... I tested it with a bunch of snippets and it seems to work nicely.
When I first teach classes in AS3 this is the template I use:
Actionscript:
-
package{
-
import flash.display.*;
-
import flash.events.*;
-
-
public class Main extends Sprite{
-
// etc...
-
}
-
}
Display and events cover a lot of ground..... next one I find myself adding is flash.geom, followed by flash.net... I'd say those are my top 4 most frequently used packages.... I do lots of text layout in the Flash IDE, otherwise flash.text would be in there....
By Zevan | February 1, 2009
Actionscript:
-
package {
-
import flash.display.*;
-
-
public class Main extends MovieClip{
-
-
private static var _display:MovieClip;
-
private static var _stage:Stage;
-
-
// use getters instead of public static vars so they
-
// cannot be reset outside of Main
-
public static function get display():MovieClip{
-
return _display;
-
}
-
-
public static function get stage():Stage{
-
return _stage;
-
}
-
-
public function Main(){
-
Main._display = this;
-
Main._stage = stage;
-
-
// test out the static references
-
var t:Test = new Test();
-
}
-
}
-
}
-
-
class Test{
-
public function Test(){
-
// test out the static references
-
with(Main.display.graphics){
-
lineStyle(1, 0xFF0000);
-
for (var i:int = 0; i<100; i++){
-
lineTo(Math.random() * Main.stage.stageWidth,
-
Math.random() * Main.stage.stageHeight);
-
}
-
}
-
}
-
}
This snippet creates two private static variables that reference the stage and the main timeline/document class. It then uses getters to regulate the use of these static vars so that they cannot be reset from outside the Main class.
Sometimes the amount of extra coding you need to do to maintain a valid stage reference can be cumbersome... similarly, passing references of the document class all around your app can be annoying. If you don't mind using two global vars in your app... this trick can come in handy.
What's nice about using getters here is that if someone tries to do this:
Actionscript:
-
Main.display = new MovieClip();
they'll get an error... in flex, you even see that little red ex pop up next to this line of code if you write it
... that wouldn't happen with a public static var....