Actionscript:
-
var ui:QuickUI = new QuickUI();
-
ui.x = 20;
-
ui.y = 260;
-
ui.addEventListener(Event.CHANGE, onChange);
-
addChild(ui);
-
-
var spriteA:Sprite = makeSprite(150,150,0xFF0000);
-
spriteA.addEventListener(MouseEvent.CLICK, onShowAddProps);
-
-
var spriteB:Sprite = makeSprite(350,150, 0xCC6600);
-
spriteB.addEventListener(MouseEvent.CLICK, onShowExcludeProps);
-
-
var spriteC:Sprite = makeSprite(550,150, 0x550066);
-
spriteC.addEventListener(MouseEvent.CLICK, onShowExcludeProps);
-
-
function onChange(evt:Event):void{
-
// updates the property on the current target object
-
ui.updateObject(evt.target);
-
}
-
function onShowAddProps(evt:MouseEvent):void{
-
ui.rows = 3;
-
// onle show the following properties
-
// add(property, label)
-
ui.add("name", "name:");
-
ui.add("x", "x location:");
-
ui.add("y", "y location:");
-
ui.add("scaleX", "x scale:");
-
ui.add("buttonMode", "show hand cursor");
-
ui.create(evt.currentTarget);
-
ui.window({title:"Select Properties: "+ evt.currentTarget.name});
-
}
-
-
function onShowExcludeProps(evt:MouseEvent):void {
-
ui.rows = 5;
-
// don't show a few select properties - if add() is not called
-
// all properties will be shown
-
ui.exclude("doubleClickEnabled");
-
ui.exclude("useHandCursor");
-
// build the UI, give two custom labels to x and y properties
-
ui.create(evt.currentTarget, {x:"x loc:", y:"y loc:"});
-
// optionally render a window behind the UI elements
-
ui.window({title:"All Properties: " + evt.currentTarget.name});
-
}
-
-
-
function makeSprite(xp:Number, yp:Number, col:uint):Sprite{
-
var s:Sprite = Sprite(addChild(new Sprite()));
-
s.x = xp;
-
s.y = yp;
-
s.buttonMode = true;
-
with (s.graphics) beginFill(col), drawRect(-40, -40, 80, 80);
-
return s;
-
}
This snippet is my first stab at creating a library that makes certain types of UI creation very easy. It works by automatically creating input text fields and check boxes for all public properties that make sense with that type of UI. It has a long way to go, but this is a good start
Take a look at the swf to get an idea of what this snippet does.
Download the source for the library and the fla for the above demo.
I hope to get this library to the point that it will at least be useful for internal UI - that is, for level editors and mini-cms systems.
Also posted in dynamic | Tagged actionscript, flash |
Actionscript:
-
package{
-
-
import flash.display.*;
-
import flash.events.*;
-
import flash.geom.*;
-
-
public class QuickCheckBox extends Sprite{
-
-
private var _checked:Boolean;
-
private var _bitmap:Bitmap;
-
private var _canvas:BitmapData;
-
-
private static var checked:BitmapData;
-
private static var unchecked:BitmapData;
-
private static var bg:Shape;
-
private static var ex:Shape;
-
private static var pnt:Point;
-
-
// static init for BitmapData and drawing
-
{
-
trace("only render your graphics once");
-
pnt = new Point(0,0);
-
checked = new BitmapData(10,10, true, 0x00000000);
-
unchecked = new BitmapData(10, 10, true, 0x00000000);
-
bg = new Shape();
-
with(bg.graphics) lineStyle(0,0x000000), beginFill(0xEFEFEF), drawRect(0,0,9,9);
-
unchecked.draw(bg);
-
ex = new Shape();
-
with(ex.graphics) {
-
lineStyle(2,0x333333), moveTo(3, 3),
-
lineTo(7, 7), moveTo(7, 3), lineTo(3, 7);
-
}
-
checked.draw(bg);
-
checked.draw(ex);
-
}
-
-
public function QuickCheckBox(value:Boolean = false):void{
-
_checked = value;
-
_canvas = new BitmapData(10,10, true, 0x00000000);
-
_bitmap = new Bitmap(_canvas);
-
addChild(_bitmap);
-
buttonMode = true;
-
render();
-
addEventListener(MouseEvent.CLICK, onClick);
-
}
-
-
public function render():void{
-
if (_checked){
-
_canvas.copyPixels(QuickCheckBox.checked, _canvas.rect, pnt);
-
}else{
-
_canvas.copyPixels(unchecked, _canvas.rect, pnt);
-
}
-
}
-
-
private function onClick(evt:Event):void{
-
_checked = !_checked;
-
render();
-
this.dispatchEvent(new Event(Event.CHANGE, true));
-
}
-
-
public function get checked():Boolean{
-
return _checked;
-
}
-
-
public function set checked(val:Boolean):void{
-
_checked = val;
-
render();
-
}
-
}
-
}
This checkbox class uses a static initializer. Static initializers can come in handy to initialize some static variables for all class instances to make use of. In this case I'm using the static initializer to create checked and unchecked BitmapData objects - all instances of QuickCheckBox use these two static BitmapData objects rather than create their own unique ones.
Here is some client code if you want to test this class out:
Actionscript:
-
for (var i:int = 0; i<100; i++){
-
var checkBox:QuickCheckBox = new QuickCheckBox(true);
-
checkBox.x =100 + i % 10 * 12;
-
checkBox.y = 100 + int(i / 10) * 12;
-
// uncheck a few checkboxes
-
if (checkBox.x == checkBox.y){
-
checkBox.checked = false;
-
}
-
addChild( checkBox);
-
// checkBox dispatches a change event
-
checkBox.addEventListener(Event.CHANGE, onChange);
-
}
-
function onChange(evt:Event):void{
-
trace(evt.currentTarget.checked);
-
}
-
/*outputs
-
only render your graphics once
-
*/
This draws 100 checkboxes, - you'll also notice in the output window that the trace statement from QuickCheckBox only runs once.
Here are 100 instances of QuickCheckBox:
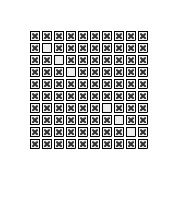
Also posted in BitmapData, OOP | Tagged actionscript, flash |
Actionscript:
-
var prevBtn:Sprite = makeArrow(100, 100);
-
prevBtn.rotation = 180;
-
var nextBtn:Sprite = makeArrow(130, 100);
-
-
var index:int = 0;
-
var vals:Array = [1,2,3,4,5,6,7,8,9,10,11];
-
var leng:int = vals.length;
-
-
trace(vals[index]);
-
-
prevBtn.addEventListener(MouseEvent.CLICK, onPrev);
-
function onPrev(evt:MouseEvent):void {
-
index--;
-
if (index <0){
-
index = leng - 1;
-
}
-
trace(vals[index%leng]);
-
}
-
nextBtn.addEventListener(MouseEvent.CLICK, onNext);
-
function onNext(evt:MouseEvent):void {
-
index++;
-
trace(vals[index%leng]);
-
}
-
-
function makeArrow(xp:Number, yp:Number):Sprite {
-
var s:Sprite = Sprite(addChild(new Sprite()));
-
s.buttonMode = true;
-
with(s.graphics) beginFill(0x666666), moveTo(0,-10), lineTo(20, 0), lineTo(0,10);
-
s.x = xp;
-
s.y = yp;
-
return s;
-
}
Previous and next buttons....
Also posted in misc | Tagged actionscript, flash |
By Zevan | April 22, 2009
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
| var buttonsWidth:Number = 0;
var buttonsA:Sprite = new Sprite();
var buttonsB:Sprite = new Sprite();
var nav:Sprite = new Sprite();
var buttonData:Array = ["one", "two", "three", "four", "five", "six",
"seven", "eight", "nine", "ten"];
buildNav();
function buildNav():void{
nav.addChild(buttonsA);
nav.addChild(buttonsB);
addChild(nav);
buildButtons(buttonsA);
buttonsWidth = buttonsA.width;
buildButtons(buttonsB);
buttonsB.x = buttonsWidth;
}
function buildButtons(container:Sprite):void{
for (var i:int = 0; i<buttonData.length; i++){
var b:MovieClip = new MovieClip();
with(b.graphics){
lineStyle(0,0x000000);
beginFill(0xCCCCCC);
drawRect(0,0,100,30);
}
b.x = i * b.width;
var txt:TextField = new TextField();
txt.scaleX = txt.scaleY = 1.5
txt.selectable = false;
txt.multiline = false;
txt.autoSize = TextFieldAutoSize.LEFT;
txt.mouseEnabled = false;
txt.x = 3;
txt.text = buttonData[i];
b.buttonMode = true;
b.addChild(txt)
container.addChild(b);
}
}
var velX:Number = 0;
var navSpeed:Number = 8;
var leftSide:Number = stage.stageWidth / 3;
var rightSide:Number = leftSide * 2;
addEventListener(Event.ENTER_FRAME, onRunNav);
function onRunNav(evt:Event):void {
if (mouseY < 100){
if (mouseX < leftSide){
velX = navSpeed;
}
if (mouseX > rightSide){
velX = -navSpeed;
}
if (nav.x < -buttonsWidth){
nav.x = -navSpeed;
}
if (nav.x > -navSpeed){
nav.x = -buttonsWidth
}
}
velX *=.9;
nav.x += velX;
} |
This snippet creates a navigation that will scroll to the left or right forever - the buttons will simply repeat. I'm not a big fan of this kind of navigation - especially if you have lots of buttons, but it seems to be a common request. This technique can be modified for use in a side-scroller style game.
Just added a new syntax highlighter, please posts comments if you have any issues viewing this snippet.
Also posted in motion | Tagged actionscript, flash |