By Zevan | March 22, 2009
Actionscript:
-
stage.frameRate = 30;
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = 200;
-
var centerY:Number = 200;
-
var p:Array = new Array();
-
var zpos:Number;
-
var xpos:Number;
-
var ypos:Number;
-
var depth:Number;
-
var canvas:BitmapData = new BitmapData(400,400,true,0xFF000000);
-
addChild(new Bitmap(canvas));
-
-
var dy:Number = 0
-
var dx:Number = 0;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
canvas.fillRect(canvas.rect, 0xFF000000);
-
-
dx += (mouseX / 100 - dx)/12;
-
dy += (mouseY / 100 - dy)/12;
-
var xp:Number, yp:Number, zp:Number;
-
-
canvas.lock();
-
for (var a:Number =0; a <TWO_PI; a+=.08){
-
for (var b:Number =0; b <TWO_PI; b+=.08){
-
xp = (70 + 40 * Math.cos(a)) * Math.cos(b) ;
-
yp = (70 + 40 * Math.cos(a)) * Math.sin(b);
-
zp = 40 * Math.sin(a);
-
calc3D(xp, yp, zp, dx, dy);
-
convert3D();
-
canvas.setPixel(p[0], p[1], 0xFFFFFF);
-
}
-
}
-
canvas.unlock();
-
}
-
function calc3D(px:Number, py:Number, pz:Number, rotX:Number=0, rotY:Number=0):void {
-
// I first learned this from code by Andries Odendaal - www.wireframe.co.za
-
zpos=pz*Math.cos(rotX)-px*Math.sin(rotX) ;
-
xpos=pz*Math.sin(rotX)+px*Math.cos(rotX) ;
-
ypos=py*Math.cos(rotY)-zpos*Math.sin(rotY) ;
-
zpos=py*Math.sin(rotY)+zpos*Math.cos(rotY);
-
}
-
function convert3D():void {
-
depth = 1/((zpos/200)+1);
-
p[0] = xpos * depth + centerX;
-
p[1] = ypos * depth + centerY;
-
}
This code draws a rotating 3D torus using setPixel().
Also posted in BitmapData | Tagged actionscript, flash |
By Zevan | January 20, 2009
Actionscript:
-
var blends:Array = [BlendMode.ADD, BlendMode.DARKEN, BlendMode.DIFFERENCE, BlendMode.HARDLIGHT, BlendMode.INVERT, BlendMode.LIGHTEN, BlendMode.MULTIPLY, BlendMode.OVERLAY, BlendMode.SCREEN, BlendMode.SUBTRACT];
and a little later...
Actionscript:
-
displace.perlinNoise(150,150, 3, 30, true, false,0,true);
-
var currentBlend:String = blends[ blendCount % blends.length];
-
displace.draw(radial, null ,null, currentBlend);
-
blendCount++;
The above are excepts from a recommendation I made in the comments of yesterdays post...
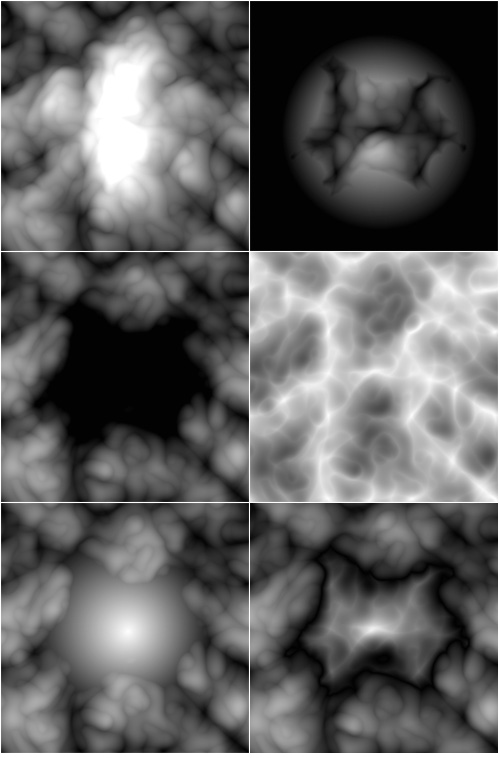
Try some different blend modes.... take a look at the swf here.
Also posted in BitmapData, misc | Tagged actionscript, flash |
By Zevan | January 19, 2009
Actionscript:
-
[SWF(width=500, height=500, backgroundColor=0xCCCCCC, frameRate=30)]
-
-
var canvas:BitmapData = new BitmapData(500, 500, true, 0xFF000000);
-
addChild(new Bitmap(canvas));
-
-
// create a radial gradient
-
var radial:Shape = new Shape();
-
var m:Matrix = new Matrix()
-
m.createGradientBox(500,500,0,0,0);
-
radial.graphics.beginGradientFill(GradientType.RADIAL, [0xFFFFFF, 0x000000], [1, 1], [0, 200], m, SpreadMethod.PAD);
-
-
radial.graphics.drawRect(0,0,500,500);
-
radial.x = radial.y = 0;
-
-
var displace:BitmapData = new BitmapData(500, 500, false,0xFF000000);
-
displace.perlinNoise(150,150, 3, 30, true, false,0,true);
-
-
// try different blendmodes here
-
displace.draw(radial, null ,null, BlendMode.LIGHTEN);
-
-
var displacementMap:DisplacementMapFilter = new DisplacementMapFilter(displace, new Point(0,0), 1, 1, 0, 0, DisplacementMapFilterMode.WRAP);
-
-
var scale:Number = 50 / stage.stageWidth;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.copyPixels(displace, canvas.rect, new Point(0,0));
-
-
displacementMap.scaleX = 25 - mouseX * scale ;
-
displacementMap.scaleY = 25 - mouseY * scale ;
-
-
canvas.applyFilter(canvas, canvas.rect, new Point(0,0), displacementMap);
-
}
This one is hard to describe, so take a look at the swf first.
I think displacement maps are underused - they're very powerful. In this snippet I use a DisplacementMapFilter to create a parallax effect on some perlin noise and a radial gradient. The result is an abstract texture that looks 3D.
I got the idea from a demo for the alternative game engine. The first time I saw this demo I had no idea how they were doing it... but after looking at it a few times, I noticed some distortion around the neck area of the model... at that point I recognized it as a displacement map and whipped up a demo (using a drawings as the source image). The alternativa demo also has some color stuff happening to simulate lighting... I'm assuming that's done with a ColorMatrixFilter or two, but I'm not sure.
As in the alternativa demo, this technique could be used with 2 images... one depth map rendered out from your favorite 3D app and one textured render....
Also posted in BitmapData, misc | Tagged actionscript, flash |
By Zevan | January 2, 2009
Actionscript:
-
var centerX:Number=stage.stageWidth/2;
-
var centerY:Number=stage.stageHeight/2;
-
// 1 to 2 ratio
-
// try others 1 / 1.5 etc...
-
var theta:Number = Math.atan(1 / 2);
-
-
var cosX:Number=Math.cos(theta);
-
var sinX:Number=Math.sin(theta);
-
var pnt:Point = new Point();
-
-
function iso3D(x:Number, y:Number, z:Number):Point {
-
pnt.x = centerX + (x-z) * cosX;
-
pnt.y = centerY - (x+z) * sinX - y;
-
return pnt;
-
}
-
-
var p:Point = iso3D(0,0,0);
-
-
graphics.beginFill(0x000000);
-
graphics.drawCircle(p.x, p.y, 2);
-
-
// x axis positive
-
trace("x = red");
-
for (var i:int = 1; i<10; i++){
-
graphics.beginFill(0xFF0000);
-
p = iso3D(i*10, 0, 0);
-
graphics.drawCircle(p.x, p.y, 2);
-
}
-
-
// y axis positive
-
trace("y = green");
-
for (i= 1; i<10; i++){
-
graphics.beginFill(0x00FF00);
-
p = iso3D(0, i * 10, 0);
-
graphics.drawCircle(p.x, p.y, 2);
-
}
-
-
// z axis positive
-
trace("z = blue");
-
for (i= 1; i<10; i++){
-
graphics.beginFill(0x0000FF);
-
p = iso3D(0, 0, i * 10);
-
graphics.drawCircle(p.x, p.y, 2);
-
}
The above code demos a conversion from isometric coordinates to cartesian coordinates. It draws out part of the x, y and z axis using the iso3D() function.
I've always faked isometric conversion by just tweaking numbers. A few years ago I created some strange isometric engines (here's another example). The other day when I wrote the isometric voxels snippet I just created the iso3D() function with a little trial and error. As you'll see it's not exactly the same as the one here. This new conversion is the result of some googling. The updated conversion is pretty close to the one that I wrote for the voxel post, but has one less multiplication... and a clearer place to control the scale ratio ...
Also posted in misc | Tagged actionscript, flash, isometric |