Actionscript:
-
[SWF(width = 600, height = 700, frameRate=24)]
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight,false, 0xFFFFFF);
-
addChild(new Bitmap(canvas));
-
-
var maxBranches:int = 600;
-
var branches:int = 0;
-
var startX:Number = 300
-
makeBranch(startX,690,30,-60, 60);
-
-
function makeBranch(xp:Number, yp:Number, step:Number, min:Number, max:Number):void {
-
var vectors:Shape = Shape(addChild(new Shape()));
-
var cX:Number, cY:Number, eX:Number, eY:Number
-
var dcX:Number=xp, dcY:Number=yp, deX:Number=xp, deY:Number=yp;
-
var theta:Number = (min + Math.random()*(max-min) - 90) * Math.PI / 180;
-
cX = xp + step * Math.cos(theta);
-
cY = yp + step * Math.sin(theta);
-
theta = (min + Math.random()*(max-min)-90) * Math.PI / 180;
-
eX = cX + step * Math.cos(theta);
-
eY = cY + step * Math.sin(theta);
-
var run:Function = function():void{
-
dcX += (cX - dcX) / 2;
-
dcY += (cY - dcY) / 2;
-
deX += (eX - deX) / 8;
-
deY += (eY - deY) / 8;
-
with(vectors.graphics){
-
clear();
-
beginFill(0xFFFFFF,0.8);
-
lineStyle(0,0x000000,0.8);
-
moveTo(startX, yp);
-
lineTo(xp, yp);
-
curveTo(dcX, dcY, deX, deY);
-
lineTo(startX, deY);
-
}
-
if (Math.abs(dcX - cX) <1 && Math.abs(deX - eX) <1 && Math.abs(dcY - cY) <1 && Math.abs(deY - eY) <1){
-
canvas.draw(vectors);
-
removeChild(vectors);
-
if (branches <maxBranches){
-
setTimeout(makeBranch, 10, deX, deY, step - Math.random(), -90, 90);
-
branches++;
-
if (int(Math.random()*2) == 1){
-
setTimeout(makeBranch, 10, deX, deY, step - Math.random()*3, -90, 90);
-
branches++;
-
}
-
}
-
}else{
-
setTimeout(arguments.callee, 1000 / 24);
-
}
-
}();
-
}
This snippet uses a technique similar to what you might use to create a recursive tree. A bit of additional logic is added for bezier branches, filled shapes and animation.
WARNING: may run slow on older machines
Have a look at the swf...
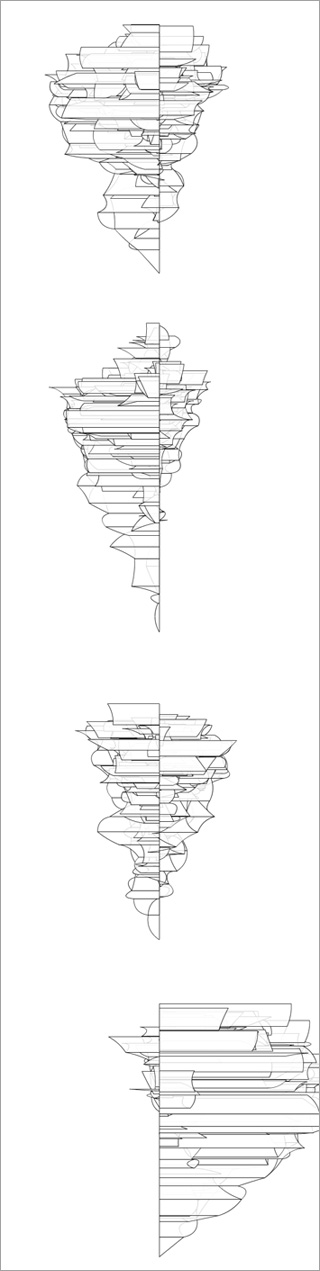
Actionscript:
-
var counter:Function = function(count:Array):Function{
-
var leng:int = count.length;
-
var index:int = 0;
-
return counter = function(reset:*=""):Function{
-
if (reset=="reset"){
-
index = 0;
-
return counter;
-
}else
-
if (reset is Array){
-
count = reset;
-
return counter;
-
}
-
trace(count[index % leng]);
-
index++;
-
return counter;
-
}
-
}
-
-
// first time it's called you pass an array
-
trace("count through the array:");
-
// you can optionally call trigger the counter by calling the newly
-
// returned function
-
counter(["a","b","c"])();
-
counter();
-
counter();
-
trace("change the array:");
-
// here we choose to simply set the array and not trigger the counter
-
counter([10,20,40,60,80]);
-
counter();
-
counter()
-
trace("reset the counter:");
-
// reset and the counter is called 3 times
-
counter("reset")()()();
-
-
/*outputs :
-
count through the array:
-
a
-
b
-
c
-
change the array:
-
10
-
20
-
reset the counter:
-
10
-
20
-
40
-
*/
Today I felt like messing around with functions and this is the result. This snippet shows a strange and interesting technique to create a function that iterates through a given array in a few different ways.
I believe the technical term for this kind of thing is a continuation...
There are a bunch of other posts like this on actionsnippet. Some are purely experimental, others are actually quite useful... these can all be found in the functions category.
Also posted in dynamic | Tagged actionscript, as3, flash |
Actionscript:
-
function create(obj:Class, props:Object):*{
-
var o:* = new obj();
-
for (var p:String in props){
-
o[p] = props[p];
-
}
-
return o;
-
}
-
-
// test out the function
-
-
var txt:TextField = create(TextField, {x:200, y:100, selectable:false, text:"hello there", textColor:0xFF0000, defaultTextFormat:new TextFormat("_sans", 20)});
-
addChild(txt);
-
-
var s:Sprite = Sprite(addChild(create(Sprite, {x:100, y:100, rotation:45, alpha:.5})));
-
-
with (s.graphics) beginFill(0xFF0000), drawRect(-20,-20,40,40);
-
-
var blur:BlurFilter = create(BlurFilter, {blurX:2, blurY:8, quality:1});
-
-
s.filters = [blur];
This snippet shows a function called create() that takes two arguments. The first argument is the name of a class to instantiate. The second is an Object with a list of properties to set on a newly created instance of the class (referenced in the first argument).
This could be particularly useful for TextFields which for some reason have no arguments in their constructor.
This will currently only work for classes that have either all optional constructor arguments or no constructor arguments.
By Zevan | April 17, 2009
Actionscript:
-
var boxDefaults:Object = {x:10, y:10, width:100, height:100, lineThickness:0, lineColor:0x000000, lineAlpha:1, fillColor:0xCCCCCC, fillAlpha:1}
-
function drawBox(params:Object=null):void {
-
var p:Object=setDefaults(boxDefaults, params);
-
graphics.lineStyle(p.lineThickness, p.lineColor, p.lineAlpha);
-
graphics.beginFill(p.fillColor, p.fillAlpha);
-
graphics.drawRect(p.x, p.y, p.width, p.height);
-
}
-
-
function setDefaults(defaults:Object, params:Object=null):Object {
-
if (params==null) {
-
params = new Object();
-
}
-
for (var key:String in defaults) {
-
if (params[key]==null) {
-
params[key]=defaults[key];
-
}
-
}
-
return params;
-
}
-
-
// test it out... notice that all object properties are optional and have default values
-
drawBox();
-
-
drawBox({x:200, y:200, lineColor:0xFF0000, lineThickness:2, fillColor:0xCCCC00});
-
-
drawBox({x:200, y:320, width:50, height:150, lineAlpha:0, fillColor:0x416CCF});
This is a technique I've been using recently... inspired by tweening engines. I find this to be suitable when a function or object constructor has lots and lots of arguments (80% of the time if I have a function or object constructor with too many arguments I re-think my design, but every now and then I use this technique).
Basically, the function or object constructor takes one argument of type Object - once passed into the function this Object argument is passed to the setDefault() function which populates it with any properties that it's missing - each property has a default value. As a result you end up with an easy to read function call with a variable number of arguments and well thought out default values.
This snippet just draws a box (not very interesting or usefull) - I wanted to showcase the technique using a simple function. As a real world example... I recently used this technique in a mini-library I created for use with Box2D... will be posting the library in the next few days.... it's a library for fast prototyping and custom rendering.
Also posted in dynamic | Tagged acitonscript, flash |