By Zevan | November 25, 2009
Actionscript:
-
var container:Sprite = new Sprite();
-
container.x = stage.stageWidth / 2;
-
container.y = stage.stageHeight / 2;
-
addChild(container);
-
-
var redBox:Sprite = new Sprite();
-
redBox.graphics.beginFill(0xFF0000);
-
redBox.graphics.drawRect(-50,-250,100,500);
-
redBox.rotationZ = 10;
-
container.addChild(redBox);
-
-
var logos:Array = []
-
var elements:Array = [];
-
elements.push({element:redBox, z:0});
-
-
// add the logos
-
for (var i:int = 0; i<6; i++){
-
var logoContainer:MovieClip = new MovieClip();
-
var logoText:TextField = new TextField();
-
logoText.defaultTextFormat = new TextFormat("_sans", 50);
-
logoText.text = "LOGO";
-
logoText.autoSize = "left";
-
logoText.selectable= false;
-
-
logoText.x = -logoText.width / 2;
-
logoText.y = -logoText.height / 2;
-
logoContainer.addChild(logoText);
-
logoText.backgroundColor = 0xFFFFFF;
-
-
container.addChild(logoContainer);
-
logos.push(logoContainer);
-
elements.push({element:logoContainer, z:0});
-
}
-
-
var ang:Number = -Math.PI / 2;
-
var rotationSpeed:Number = 0.05;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
var dx:Number = (mouseY - stage.stageHeight / 2) / 10;
-
var dy:Number = (mouseX - stage.stageWidth / 2) / 10;
-
container.rotationX += (dx - container.rotationX) / 4;
-
container.rotationY += (dy - container.rotationY) / 4;
-
-
ang += rotationSpeed;
-
for (var i:int = 0; i<logos.length; i++){
-
var logo:Sprite = logos[i];
-
logo.x = 150 * Math.cos(ang + i);
-
logo.z = 150 * Math.sin(ang + i);
-
logo.alpha = 1 - logo.z / 200;
-
logo.rotationY = -Math.atan2(logo.z, logo.x) / Math.PI * 180 - 90;
-
}
-
-
// z-sort
-
for (i = 0; i<elements.length; i++){
-
elements[i].z = elements[i].element.transform.getRelativeMatrix3D(this).position.z;
-
}
-
-
elements.sortOn("z", Array.NUMERIC | Array.DESCENDING);
-
for (i = 0; i<elements.length; i++) {
-
container.addChild(elements[i].element);
-
}
-
}
A student of mine was having trouble creating a 3D logo for a client. I created this snippet to help explain how some of the fp10 3D stuff works.... z-sorting etc... The code could be optimized a bit... but it works nicely...
Have a look at the swf...
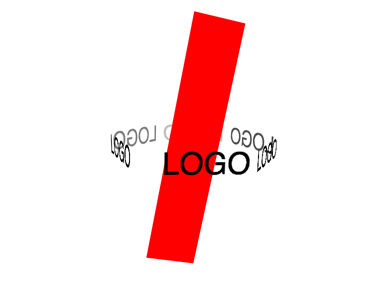
Also posted in 3D, misc | Tagged actionscript, as3, flash, fp10 |
Actionscript:
-
package {
-
import flash.display.Shape;
-
import flash.display.Sprite;
-
import flash.geom.Point;
-
-
/**
-
* Re: http://board.flashkit.com/board/showthread.php?t=797453
-
* @author makc
-
* @license WTFPLv2
-
*/
-
public class BouncingBall extends Sprite{
-
public function BouncingBall () {
-
r = 10;
-
ball = new Shape;
-
ball.graphics.beginFill (0);
-
ball.graphics.drawCircle (0, 0, r);
-
addChild (ball);
-
v = new Point;
-
v.x = Math.random ();
-
v.y = Math.random ();
-
V = 1 + 20 * Math.random ();
-
v.normalize (V);
-
R = 200; X = 465 / 2; Y = 465 / 2;
-
graphics.lineStyle (0);
-
graphics.drawCircle (X, Y, R);
-
ball.x = X + 100;
-
ball.y = Y - 100;
-
addEventListener ("enterFrame", loop);
-
}
-
private var r:Number;
-
private var ball:Shape;
-
private var v:Point;
-
private var V:Number;
-
private var R:Number;
-
private var X:Number;
-
private var Y:Number;
-
private function loop (e:*):void {
-
ball.x += v.x;
-
ball.y += v.y;
-
// R-r vector
-
var P:Point = new Point (X - ball.x, Y - ball.y);
-
if (P.length> Math.sqrt ((R - r) * (R - r))) {
-
// normalize R-r vector
-
P.normalize (1);
-
// project v onto it
-
var vp:Number = v.x * P.x + v.y * P.y;
-
// subtract projection
-
v.x -= 2 * vp * P.x;
-
v.y -= 2 * vp * P.y;
-
v.normalize (V);
-
// move away from bounding circle
-
P = new Point (X - ball.x, Y - ball.y);
-
while (P.length> Math.sqrt ((R - r) * (R - r))) {
-
ball.x += v.x;
-
ball.y += v.y;
-
P = new Point (X - ball.x, Y - ball.y);
-
}
-
}
-
}
-
}
-
}
Makc3d said I could choose one of his excellent wonderfl.net pieces and submit it to the contest. This snippet creates a ball that bounces off the inside of a circle. I thought this was a pretty unique way to go about doing this - and found it easy to add gravity and other cool features to it.
Have a look at the swf over at wonderfl....
Some Makc3d links:
>> http://makc3d.wordpress.com/
>>http://code.google.com/p/makc/
>> http://wonderfl.net/user/makc3d
Makc3d elaborated on his code a bit via e-mail. He said that his technique sacrifices accuracy for simplicity... and that if you simplify too much it would be easy to break the code. "e.g. comment out piece of code where it says "move away from bounding circle""...
Here is a picture that Makc3d drew explaining why you need to multiply by 2:
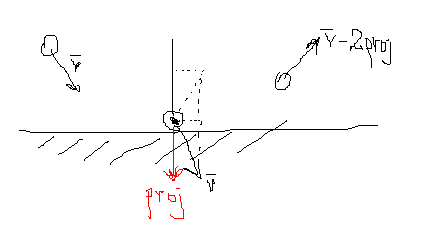
By Zevan | November 7, 2009
Since the release of QuickBox2D 1.0 two bugs were discovered by numerous developers. The first bug was the inability to properly destroy group objects. The second bug was a small memory leak that caused most QuickObjects to remain in memory. Both of these bugs are now resolved.
Download QuickBox2D 1.1
Testing the memory leak. In QuickBox2D 1.0 if you created and destroyed 100s of rigid bodies, the ram would very slowly rise... Sometimes it would get garbage collected, but the memory would never fully be released. I created a simple test to make sure this is fixed in 1.1:
Actionscript:
-
import com.actionsnippet.qbox.*;
-
-
var sim:QuickBox2D = new QuickBox2D(this, {debug:false});
-
-
sim.createStageWalls();
-
-
var levelParts:Array = [];
-
const TWO_PI:Number = Math.PI * 2;
-
-
// destroys and then creates a bunch of rigid bodies
-
// called every second
-
buildRandomLevel();
-
setInterval(buildRandomLevel, 1000);
-
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.x = txt.y = 30;
-
txt.backgroundColor = 0xFFFFFF;
-
sim.start();
-
-
// display amount of ram used, to make sure garbage collection is working
-
sim.addEventListener(QuickBox2D.STEP, onStep);
-
function onStep(evt:Event):void{
-
txt.text = (System.totalMemory / 100000).toFixed(2) + "mb";
-
}
-
-
function buildRandomLevel():void{
-
// destroy all rigid bodies
-
for (var i:int = 0; i<levelParts.length; i++){
-
levelParts[i].destroy();
-
}
-
levelParts = [];
-
// create a bunch of circles and boxes
-
for (i = 0; i<16; i++){
-
var rad:Number = 0.4 ;
-
levelParts.push(sim.addCircle({x:1 + i * rad * 4, y : 2, radius:rad - Math.random()*0.3}));
-
-
var rot:Number = Math.random() * TWO_PI;
-
levelParts.push(sim.addBox({x:4+Math.random() * i * 2, y:4+Math.random()*i,
-
width:3, height:0.25, angle:rot, density:0}));
-
}
-
}
This snippet creates and destroys a bunch of rigid bodies again and again.... On my macbook 2.6 Ghz intel core duo... the ram runs between 79mb and 112mb. I let it run for 40 minutes and this did not change - it always eventually returned down to 79mb.
Have a look at the swf...
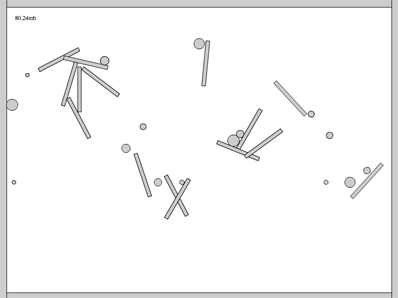
Thanks to all the people who gave feedback that lead to the discover of these bugs.
By Zevan | October 29, 2009
Actionscript:
-
[SWF(width = 100, height = 100)]
-
var circle:Shape = new Shape();
-
with(circle.graphics) beginFill(0x000000), drawCircle(20,20,20);
-
-
var currFrame:Frame;
-
-
// populate the linked list
-
generateAnimation();
-
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight,false, 0x000000);
-
addChild(new Bitmap(canvas));
-
var loc:Point = new Point(20, 20);
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
// clear the canvas
-
canvas.fillRect(canvas.rect, 0x000000);
-
// draw the current frame
-
canvas.copyPixels(currFrame.bitmap, currFrame.bitmap.rect, loc, null, null, true);
-
// get the next frame of the animation
-
currFrame = currFrame.next;
-
}
-
-
// generate and capture 40 bitmaps by altering the colorTransform of the circle shape
-
function generateAnimation():void{
-
var channel:uint = 0;
-
var ct:ColorTransform = new ColorTransform();
-
var increase:Boolean = true;
-
var firstFrame:Frame;
-
var pFrame:Frame;
-
for (var i:int = 0; i<40; i++){
-
if (increase){
-
channel += 10;
-
if (channel == 200){
-
increase = false;
-
}
-
}else{
-
channel -= 10;
-
}
-
ct.color = channel <<16 | channel <<8 | channel;
-
circle.transform.colorTransform = ct;
-
// populate linked list
-
currFrame = capture(circle);
-
if (pFrame){
-
pFrame.next = currFrame;
-
}
-
if (i == 0){
-
firstFrame = currFrame;
-
}
-
pFrame = currFrame;
-
}
-
// close the list
-
currFrame.next = firstFrame;
-
currFrame = firstFrame;
-
}
-
-
// create the Frame instance and draw the circle to it
-
// preserving the colorTransform information
-
function capture(target:Shape):Frame{
-
var frame:Frame = new Frame();
-
frame.bitmap = new BitmapData(target.width, target.height, true, 0x00000000);
-
frame.bitmap.draw(target, null, target.transform.colorTransform);
-
return frame;
-
}
Requires this little Frame class
Actionscript:
-
package {
-
import flash.display.*;
-
final public class Frame{
-
public var bitmap:BitmapData;
-
public var next:Frame;
-
}
-
}
This is a small test I did today to see how easy it would be to use a circular linked list to loop an animation of bitmaps. I did this because I was thinking about using some animated sprites in conjunction with Utils3D.projectVectors() to do an orthographic 3D demo with lots of animating sprites. In the past I've had up to 7,000 animated sprites running nicely using arrays and copyPixels... figured it would be interesting to try and do the same with a circular linked list.
When compiled, this test simply draws a circle that fades from black to gray and back again... Pretty boring, but I threw it up over at wonderfl anyway... check it out.
I recently saw a few tweets (forget who from) about using the final keyword on linked list nodes... haven't tested it myself but it's supposed to be faster...