Actionscript:
-
import com.actionsnippet.qbox.*;
-
-
var sim:QuickBox2D = new QuickBox2D(this, {debug:true});
-
// use the box2D default renderer (debug : true)
-
-
sim.createStageWalls();
-
-
// anchor
-
var pre:QuickObject = sim.addCircle({x:9, y:3, radius:.5, density:0});
-
-
// create a chain of boxes
-
for (var i:int = 0; i<12; i++){
-
var curr:QuickObject = sim.addBox({x:10 + i, y:3, width:.9, height:.9, angularDamping:1});
-
-
// currently always adds a b2DistanceJoint
-
sim.addJoint({a:pre.body, b:curr.body,
-
x1:9 + i, y1: 3, x2: 10 + i, y2:3, collideConnected:false});
-
pre = curr;
-
}
-
-
// add a circle, use CCD (isBullet)
-
sim.addCircle({x:20, y:10, radius:1, isBullet:true});
-
-
// start simulation
-
sim.start();
-
sim.mouseDrag();
This snippet uses my QuickBox2D library to create a chain. QuickBox2D is a wrapper for Box2DFlashAS3 that greatly simplifies world setup, instantiation and graphical skinning of rigid bodies (more info on yesterdays post)...
See the swf here.
Download Box2DFlashAS3 here
Download QuickBox2D Alpha 104 here
See yesterdays post for additional information. I'm still in the process of writing some docs and possibly a tutorial for QuickBox2D... hopefully I'll finish with that soon...
If your somewhat familiar with Box2D and just want to just dig right in, the following scrap of information may help:
// Methods of the QuickBox2D class, used for creating rigid bodies and joints
addCircle();
addBox();
addPoly();
// currently only creates b2DistanceJoints
addJoint();
these function all take one parameter Object as their argument. The common parameter properties for rigid bodies are:
// QuickBox2D simpleRender settings:
lineColor:0x000000
lineAlpha:1
lineThickness:0
fillColor:0xCCCCCC
fillAlpha:1
// wrapped Box2D properties
x:3
y:3
density:1
friction:0.5
restitution:0.2
angle: 0
linearDamping:0
angularDamping:0
isBullet:false,
fixedRotation:false
allowSleep: true
isSleeping:false
// advanced use
maskBits:0xFFFF
categoryBits:1
groupIndex:0
//and the specific param properties are:
addCircle() : radius
addBox() : width, height
addPoly() : verts
//Joints have the following properties... not going to bother explaining these until I write the docs:
addJoint() : a, b, x1, x2, y1, y2
By Zevan | April 30, 2009
Actionscript:
-
[SWF(backgroundColor = 0x333333)]
-
-
import com.actionsnippet.qbox.*;
-
-
// setting debug = true, will use the Box2D debug rendering
-
var sim:QuickBox2D=new QuickBox2D(this, {debug:false, gravityY:10});
-
-
// creates static boxes on all sides of the screen
-
sim.createStageWalls({lineAlpha:0, fillColor:0xFF9900});
-
-
// add 25 boxes
-
for (var i:int = 0; i<25; i++){
-
var xp:Number = 3 + (i % 5);
-
var yp:Number = 1 + int( i / 5);
-
sim.addBox({x:xp, y:yp, width:1, height:1, fillColor: i * 10 <<16, lineAlpha:0, angularDamping:5});
-
}
-
-
sim.addBox({x:7, y:10, width:3, height:.2, angle:-.3, density:0,lineAlpha:0, fillColor:0xFF9900});
-
-
sim.addCircle({x:3, y:14, radius:2, fillColor:0xCC0000, lineColor:0x333333});
-
-
sim.addPoly({x:13, y:5, verts:[[1,0,2,2,1,1.33],[1,0,1,1.33,0,2]], angle: .4, density:1});
-
-
// begins the simulation
-
sim.start();
-
-
// all non-static objects can be dragged
-
sim.mouseDrag();
I've been messing with Box2D for the past few weeks - I decided to create a library (QuickBox2D) for quick prototyping. This snippet makes use of this library, so in order to run it you'll need to download the zip included at the end of this post...
If you don't already know, Box2D is a great physics library created by Erin Catto. It was ported to AS3 by Matthew Bush and John Nesky. You can download the AS3 version of the library here.
Today's snippet creates this swf (click any image to view demo):
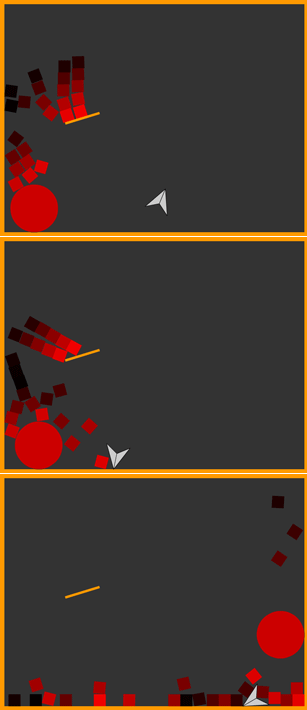
QuickBox2D
QuickBox2D simplifies Box2D instantiation and makes it easy to skin rigid bodies with Library assets... normally you need to do something like this to create a box in Box2D:
Actionscript:
-
var bodyDef:b2BodyDef = new b2BodyDef();
-
bodyDef.position.Set(3, 3);
-
var boxDef:b2PolygonDef = new b2PolygonDef();
-
boxDef.SetAsBox(1, 1);
-
boxDef.density = 1;
-
var body:b2Body = w.CreateBody(bodyDef);
-
body.CreateShape(boxDef);
-
body.SetMassFromShapes();
and this doesn't include setting up the physics world and the main loop to run the simulation... after writing a good deal of repetitive code I decided to make QuickBox2D. After writing lots of QuickBox2D style instantiation I decided to create an editor so that I could just draw circles, boxes, polygons and joints and then test and save them in real time (will be posting this editor in the near future). The next few posts will be about this mini-library and I'll be writing up some real documentation for it... as of now, it doesn't have any...
You can download Box2D here
You can download QuickBox2D here
and you can view some AS3 Box2D demos here
Also posted in QuickBox2D | Tagged actionscript, flash |
By Zevan | April 29, 2009
Actionscript:
-
[SWF(width = 800, height = 800)]
-
var a:Number = 0.19;
-
var b:Number = .9;
-
var c:Number = 1.3
-
var xn1:Number = 5;
-
var yn1:Number = 0;
-
var xn:Number, yn:Number;
-
-
var scale:Number =40;
-
var iterations:Number = 20000;
-
-
function f(x:Number):Number{
-
// too lazy to simplify this at the moment
-
return((x + .1 + x * (a - c) * x) / (1.1 + a * (c*c + a*a) * x * x )) * 1.3;
-
}
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(800,800,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
-
c += ((stage.stageWidth/2 - mouseX) / 8000 - c) / 2;
-
-
xn1 = 0;
-
yn1 = 0;
-
for (var i:int = 0; i<iterations; i++){
-
xn = xn1;
-
yn = yn1;
-
-
xn1 = -xn - a + c + f(yn);
-
yn1 = -xn + c * f(xn * yn);
-
canvas.setPixel( 380 + xn1 * scale, 450 + yn1 * scale, 0x000000);
-
}
-
}
I was randomly messing around with strange attractors a few weeks back and this is one of the better results.... I arbitrarily altered equations until I got a nice result. You can move your mouse left and right to alter some of the params. Here is what it looks like when your mouse is in the middle of the screen:
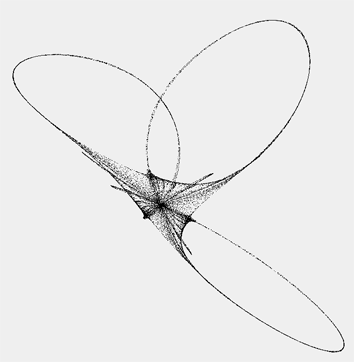
By Zevan | April 22, 2009
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
| var buttonsWidth:Number = 0;
var buttonsA:Sprite = new Sprite();
var buttonsB:Sprite = new Sprite();
var nav:Sprite = new Sprite();
var buttonData:Array = ["one", "two", "three", "four", "five", "six",
"seven", "eight", "nine", "ten"];
buildNav();
function buildNav():void{
nav.addChild(buttonsA);
nav.addChild(buttonsB);
addChild(nav);
buildButtons(buttonsA);
buttonsWidth = buttonsA.width;
buildButtons(buttonsB);
buttonsB.x = buttonsWidth;
}
function buildButtons(container:Sprite):void{
for (var i:int = 0; i<buttonData.length; i++){
var b:MovieClip = new MovieClip();
with(b.graphics){
lineStyle(0,0x000000);
beginFill(0xCCCCCC);
drawRect(0,0,100,30);
}
b.x = i * b.width;
var txt:TextField = new TextField();
txt.scaleX = txt.scaleY = 1.5
txt.selectable = false;
txt.multiline = false;
txt.autoSize = TextFieldAutoSize.LEFT;
txt.mouseEnabled = false;
txt.x = 3;
txt.text = buttonData[i];
b.buttonMode = true;
b.addChild(txt)
container.addChild(b);
}
}
var velX:Number = 0;
var navSpeed:Number = 8;
var leftSide:Number = stage.stageWidth / 3;
var rightSide:Number = leftSide * 2;
addEventListener(Event.ENTER_FRAME, onRunNav);
function onRunNav(evt:Event):void {
if (mouseY < 100){
if (mouseX < leftSide){
velX = navSpeed;
}
if (mouseX > rightSide){
velX = -navSpeed;
}
if (nav.x < -buttonsWidth){
nav.x = -navSpeed;
}
if (nav.x > -navSpeed){
nav.x = -buttonsWidth
}
}
velX *=.9;
nav.x += velX;
} |
This snippet creates a navigation that will scroll to the left or right forever - the buttons will simply repeat. I'm not a big fan of this kind of navigation - especially if you have lots of buttons, but it seems to be a common request. This technique can be modified for use in a side-scroller style game.
Just added a new syntax highlighter, please posts comments if you have any issues viewing this snippet.
Also posted in UI | Tagged actionscript, flash |