By Zevan | March 19, 2010
Actionscript:
-
[SWF(width = 500, height = 500)]
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = stage.stageWidth / 2;
-
var centerY:Number = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
// data
-
var points:Array = [];
-
var i:int = 0;
-
var pointNum : int = Math.max(2,int(mouseX / 12))
-
-
var radius:Number = 200;
-
var step:Number = TWO_PI / pointNum;
-
var theta:Number = step / 2;
-
for (i = 0; i<pointNum; i++){
-
var xp:Number = centerX + radius * Math.cos(theta);
-
var yp:Number = centerY + radius * Math.sin(theta);
-
points[i] = new Point(xp, yp);
-
theta += step;
-
}
-
// render
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for ( i = 0; i<pointNum; i++){
-
var a:Point = points[i];
-
for (var j:int = 0; j<pointNum; j++){
-
var b:Point = points[j];
-
if (a != b){
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
}
-
}
I've been using this geometric shape for lots of different things recently. Including during consulting gigs as a helpful visualization. Just move your mouse left and right... I particularly like the simpler forms you get by moving your mouse to the left (triangles squares and simple polygons):
While not entirely related this wikipedia article is interesting.
[EDIT : Thanks to martin for reminding me that I can do away with the if statement here in the above code ]
Actionscript:
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (i = 0; i<pointNum; i++) {
-
var a:Point=points[i];
-
for (var j:int = i+1; j<pointNum; j++) {
-
var b:Point=points[j];
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
graphics.drawCircle(a.x, a.y, 10);
I implemented that change over at wonderfl and it works nicely
Also posted in Graphics, misc | Tagged actionscript, as3, flash |
If you're at all interested in watching me free from code. I recorded a video of me coding this snippet (which is about 11 minutes long or so).
In the video I create a few functions that allow you to draw shapes like these:
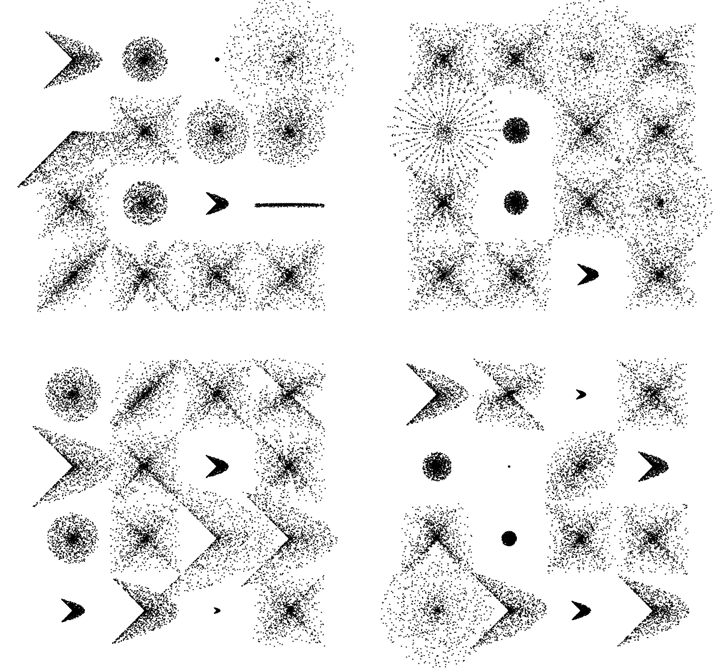
Mathematically this stuff is really simple ... the free form nature of the video takes a less technical perspective as you'll see (I even made a few funny mistakes).
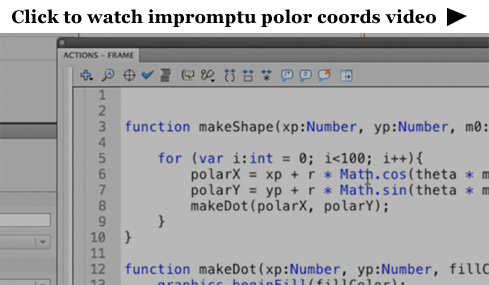
Actionscript:
-
[SWF(width = 600, height = 600)]
-
var dotNum:int = 1000;
-
var dotRad:Number = 0.5;
-
-
x = 120
-
y = 100;
-
-
// extra stuff to display what the functions can do
-
stage.addEventListener(MouseEvent.CLICK, onDrawAll);
-
-
function onDrawAll(evt:Event):void{
-
graphics.clear();
-
for (var i:int = 0; i<16; i++){
-
var m:Number;
-
-
var rad:Number = 120;
-
var xp:Number = i % 4 * rad
-
var yp:Number = int(i / 4) * rad
-
-
var type:int = int(Math.random() * 4);
-
if (type == 0){
-
makeShape(xp, yp, rad-60, Math.random() , 1);
-
}else if (type == 1){
-
makeShape(xp, yp, rad-60, 1, Math.random());
-
}
-
else if (type == 2){
-
m = Math.random() * 2;
-
makeShape(xp, yp, rad-Math.random()*120, m, m);
-
}
-
else if (type == 3){
-
m = Math.random() * 2;
-
makeShape(xp, yp, rad-Math.random()*120, m, m/2);
-
}
-
}
-
}
-
-
// main part from the video
-
function makeShape(xp:Number, yp:Number,
-
maxRad:Number = 100,m0:Number=1,
-
m1:Number=1):void{
-
var polarX:Number;
-
var polarY:Number;
-
var radius:Number;
-
graphics.lineStyle(0, 0);
-
var theta:Number = Math.random() * Math.PI * 2;
-
for (var i:int = 0; i<dotNum; i++){
-
radius = Math.random() * maxRad
-
polarX = xp + radius * Math.cos(theta * m0);
-
polarY = yp + radius * Math.sin(theta * m1);
-
theta += 0.1;
-
-
makeDot(polarX, polarY);
-
-
}
-
}
-
-
function makeDot(xp:Number, yp:Number, fillColor:uint = 0x000000):void{
-
graphics.beginFill(fillColor);
-
graphics.drawCircle(xp, yp, dotRad);
-
graphics.endFill();
-
}
Here it is over at wonderf:
By Zevan | February 4, 2010
Today's quiz is not multiple choice. Instead, your task is to write a lisp style math parser. This may sound tricky, but it's surprisingly simple. (well... not simple exactly, it's just simple compared to what one might assume).
Lisp uses prefix notation... where the operator is placed before the operands:
10 * 10
becomes:
* 10 10
You could think of this as a function "*" with two arguments (10, 10). In Lisp this is enclosed with parens:
(* 10 10)
Let's see a few more examples:
100 / 2 + 10
becomes:
(+ (/ 100 2) 10)
...
2 * 4 * 6 * 7
becomes:
(* 2 4 6 7)
...
(2 + 2) * (10 - 2) * 2
becomes
(* (+ 2 2) (- 10 2) 2)
Remember, thinking "functions" really helps. The above can be though of as:
multiply( add(2, 2), subtract(10 , 2), 2)
You should create a function called parsePrefix() that takes a string and returns a number:
Here is some code to test if your parser works properly:
Actionscript:
-
trace(parsePrefix("(* 10 10)"));
-
-
trace(parsePrefix("(* 1 (+ 20 2 (* 2 7) 1) 2)"));
-
-
trace(parsePrefix("(/ 22 7)"));
-
-
trace(parsePrefix("(+ (/ 1 1) (/ 1 2) (/ 1 3) (/ 1 4))"));
-
-
/* Should trace out:
-
100
-
74
-
3.142857142857143
-
2.083333333333333
-
*/
I highly recommend giving this a try, it was one of those cases where I assumed it would be much trickier than it was.
I've posted my solution here.
Also posted in Quiz | Tagged actionscript, as3, flash, Lisp, Quiz |
By Zevan | November 29, 2009
Actionscript:
-
// print some floats as fractions
-
printFraction(0.5);
-
printFraction(0.75);
-
printFraction(0.48);
-
-
// try something a little more complex
-
var float:Number = 0.98765432;
-
trace("\na more complex example:");
-
printFraction(float);
-
var frac:Array = asFraction(float);
-
trace("double check it:");
-
trace(frac[0] + "/" + frac[1] +" = " + frac[0] / frac[1]);
-
-
/* outputs:
-
0.5 = 1/2
-
0.75 = 3/4
-
0.48 = 12/25
-
-
a more complex example:
-
0.98765432 = 12345679/12500000
-
double check it:
-
12345679/12500000 = 0.98765432
-
*/
-
-
-
function printFraction(n:Number):void{
-
var frac:Array = asFraction(n);
-
trace(n + " = " + frac[0] + "/" + frac[1]);
-
}
-
-
// takes any value less than one and returns an array
-
// with the numerator at index 0 and the denominator at index 1
-
function asFraction(num:Number):Array{
-
var decimalPlaces:int = num.toString().split(".")[1].length;
-
var denom:Number = Math.pow(10, decimalPlaces);
-
return reduceFraction(num * denom, denom);
-
}
-
-
// divide the numerator and denominator by the GCF
-
function reduceFraction(numerator:int, denominator:Number):Array{
-
// divide by the greatest common factor
-
var divisor:int = gcf(numerator, denominator);
-
if (divisor){
-
numerator /= divisor;
-
denominator /= divisor;
-
}
-
return [numerator, denominator];
-
}
-
-
// get the greatest common factor of two integers
-
function gcf(a:int, b:int):int{
-
var remainder:int;
-
var factor:Number = 0;
-
var maxIter:int = 100;
-
var i:int = 0;
-
while (1){
-
if (b> a){
-
var swap:int = a;
-
a = b;
-
b = swap;
-
}
-
remainder = a % b;
-
a = b;
-
b = remainder
-
if (remainder == 0){
-
factor = a;
-
break;
-
}else if (remainder==1){
-
break;
-
}else if (i> maxIter){
-
trace("failed to calculate gcf");
-
break;
-
}
-
i++;
-
}
-
return factor;
-
}
This snippet contains a few functions for calculating fractions based on float values. Writing this brought back some memories from grade school math.
Also posted in misc | Tagged actionscript, as3, flash |