By Zevan | March 22, 2010
I was messing with Catumull Rom spline code from awhile back and this this came out:
Actionscript:
-
[SWF(width = 500, height= 500)]
-
var pnts:Array = new Array();
-
// make 8 control points
-
for (var i:int = 0; i<8; i++){
-
pnts.push(dot(50 + Math.random() * 80 * i, Math.random()*(stage.stageHeight-40)+20));
-
}
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
curve(pnts);
-
}
-
function tangent(pk1:Sprite, pk_1:Sprite){
-
return new Point((pk1.x - pk_1.x) / 2, (pk1.y - pk_1.y) / 2);
-
}
-
// all math from http://en.wikipedia.org/wiki/Cubic_Hermite_spline
-
function curve(p:Array, res:Number=.05):void{
-
var px:Number = 0;
-
var py:Number = 0;
-
var pIter:int = p.length - 1;
-
var m:Array = [];
-
m[0] = tangent(p[1], p[0]);
-
for (var i:int = 1; i<pIter; i++){
-
m[i] = tangent(p[i + 1], p[i - 1]);
-
}
-
m[pIter] = tangent(p[pIter], p[pIter - 1]);
-
for (var t:Number = 0; t <1; t+=res){
-
var t_2:Number = t * t;
-
var _1_t:Number = 1 - t;
-
var _2t:Number = 2 * t;
-
var h00:Number = (1 + _2t) * (_1_t) * (_1_t);
-
var h10:Number = t * (_1_t) * (_1_t);
-
var h01:Number = t_2 * (3 - _2t);
-
var h11:Number = t_2 * (t - 1);
-
for (var k:int = 0; k <pIter; k++){
-
var k1:int = k + 1;
-
var pk:Sprite = p[k];
-
var pk1:Sprite = p[k1];
-
var mk:Point = m[k];
-
var mk1:Point = m[k1];
-
-
px = h00 * pk.x + h10 * mk.x + h01 * pk1.x + h11 * mk1.x;
-
py = h00 * pk.y + h10 * mk.y + h01 * pk1.y + h11 * mk1.y;
-
if (k == 0){
-
graphics.moveTo(px, py);
-
}else{
-
graphics.lineTo(px, py);
-
}
-
-
// canvas.setPixel(px, py, 0xFFFFFF);
-
}
-
}
-
}
-
// draggable dot
-
function dot(xp:Number, yp:Number, col:uint = 0xFF0000, rad:Number=4):Sprite {
-
var s:Sprite = Sprite(addChild(new Sprite));
-
s.x = xp;
-
s.y = yp;
-
-
with(s.graphics) beginFill(col), drawCircle(0,0,rad);
-
s.buttonMode = true;
-
s.addEventListener(MouseEvent.MOUSE_DOWN, onDrag);
-
return s;
-
}
-
function onDrag(evt:MouseEvent):void {
-
evt.currentTarget.startDrag()
-
}
-
stage.addEventListener(MouseEvent.MOUSE_UP, onUp);
-
stage.addEventListener(MouseEvent.MOUSE_DOWN,onDown);
-
function onDown(evt:MouseEvent):void{
-
dotsVisible(false);
-
}
-
function onUp(evt:MouseEvent):void{
-
stopDrag();
-
dotsVisible(true);
-
}
-
-
function dotsVisible(bool:Boolean):void{
-
for (var i:int = 0; i<pnts.length; i++){
-
pnts[i].visible = bool;
-
}
-
}
You can see the swf over at wonderfl.
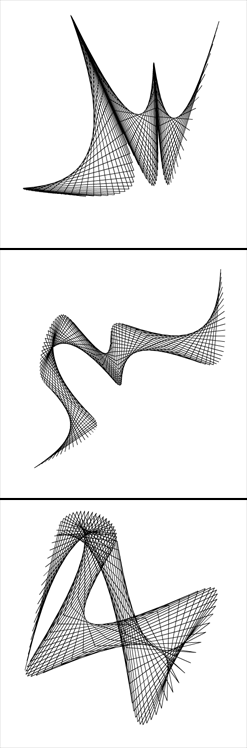
By Zevan | March 20, 2010
We're still working on this long term project for medialab prado in madrid. Here is a camera test from today. It uses frame differencing and places names of colors on the areas of motion.
Click here to view the swf file... make sure to move around a bit in front of your web-cam.
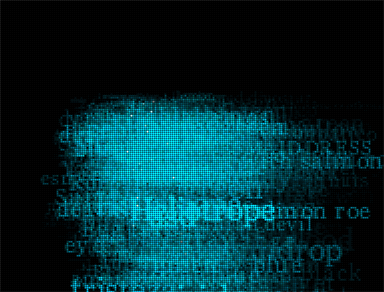
By Zevan | March 19, 2010
Actionscript:
-
[SWF(width = 500, height = 500)]
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = stage.stageWidth / 2;
-
var centerY:Number = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
// data
-
var points:Array = [];
-
var i:int = 0;
-
var pointNum : int = Math.max(2,int(mouseX / 12))
-
-
var radius:Number = 200;
-
var step:Number = TWO_PI / pointNum;
-
var theta:Number = step / 2;
-
for (i = 0; i<pointNum; i++){
-
var xp:Number = centerX + radius * Math.cos(theta);
-
var yp:Number = centerY + radius * Math.sin(theta);
-
points[i] = new Point(xp, yp);
-
theta += step;
-
}
-
// render
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for ( i = 0; i<pointNum; i++){
-
var a:Point = points[i];
-
for (var j:int = 0; j<pointNum; j++){
-
var b:Point = points[j];
-
if (a != b){
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
}
-
}
I've been using this geometric shape for lots of different things recently. Including during consulting gigs as a helpful visualization. Just move your mouse left and right... I particularly like the simpler forms you get by moving your mouse to the left (triangles squares and simple polygons):
While not entirely related this wikipedia article is interesting.
[EDIT : Thanks to martin for reminding me that I can do away with the if statement here in the above code ]
Actionscript:
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (i = 0; i<pointNum; i++) {
-
var a:Point=points[i];
-
for (var j:int = i+1; j<pointNum; j++) {
-
var b:Point=points[j];
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
graphics.drawCircle(a.x, a.y, 10);
I implemented that change over at wonderfl and it works nicely
By Zevan | March 15, 2010
Actionscript:
-
[SWF(width = 500, height=500, backgroundColor=0x000000)]
-
-
var clockNum:int = 100;
-
var clocks:Vector.<Function> = new Vector.<Function>(clockNum, true);
-
-
var clockContainer:Sprite = Sprite(addChild(new Sprite()));
-
clockContainer.x = stage.stageWidth / 2;
-
clockContainer.y = stage.stageHeight / 2;
-
buildClocks();
-
runClocks();
-
-
function buildClocks():void{
-
for (var i:int = 0; i<clockNum; i++){
-
var theta:Number = Math.random() * Math.PI * 2;
-
var radius:Number = Math.random() * 200;
-
var xp:Number = radius * Math.cos(theta);
-
var yp:Number = radius * Math.sin(theta);
-
clocks[i] = makeClock(xp,yp,Math.random() * Math.PI * 2);
-
}
-
}
-
function runClocks():void{
-
addEventListener(Event.ENTER_FRAME, onRunClocks);
-
}
-
function onRunClocks(evt:Event):void{
-
for (var i:int = 0; i<clockNum; i++){
-
clocks[i]();
-
}
-
clockContainer.rotationX = clockContainer.mouseY / 30;
-
clockContainer.rotationY = -clockContainer.mouseX / 30;
-
}
-
function makeClock(x:Number, y:Number, time:Number=0):Function{
-
var radius:Number = Math.random() * 20 + 5;
-
var border:Number = radius * 0.2;
-
var smallRadius:Number = radius - radius * 0.3;
-
-
var clock:Sprite = Sprite(clockContainer.addChild(new Sprite()));
-
clock.x = x;
-
clock.y = y;
-
clock.z = 100 - Math.random() * 200;
-
clock.rotationX = Math.random() * 40 - 20;
-
clock.rotationY = Math.random() * 40 - 20;
-
clock.rotationZ = Math.random() * 360;
-
return function():void{
-
with (clock.graphics){
-
clear();
-
lineStyle(1,0xFFFFFF);
-
drawCircle(0,0,radius + border);
-
var xp:Number = smallRadius * Math.cos(time/2);
-
var yp:Number = smallRadius * Math.sin(time/2);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
xp = radius * Math.cos(time);
-
yp = radius * Math.sin(time);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
}
-
time+=0.1;
-
}
-
}
You can go check the swf out at wonderfl.net...
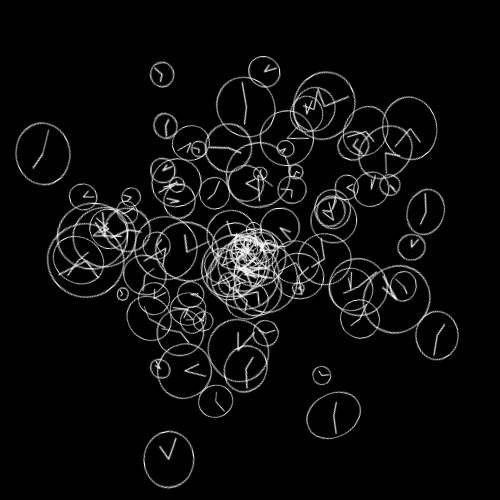