Actionscript:
-
package {
-
import flash.display.Sprite;
-
public class Chaining extends Sprite{
-
public function Chaining(){
-
print(n(100).divide(2).plus(2));
-
// outputs 52
-
print(n(100).plus(n(10).multiply(2)));
-
// outputs 120
-
}
-
}
-
}
-
-
function print(n:Num):void{
-
trace(n.getValue());
-
}
-
function n(n:Number):Num{
-
return new Num(n);
-
}
-
-
class Num{
-
private var value:Number;
-
-
public function Num(n:Number):void{
-
value = n;
-
}
-
private function convert(n:*):Number{
-
if (n is Num) n = n.getValue();
-
return n;
-
}
-
public function getValue():Number{
-
return value;
-
}
-
public function plus(n:*):Num{
-
n = convert(n);
-
value += n;
-
return this;
-
}
-
public function minus(n:*):Num{
-
n = convert(n);
-
value -= n;
-
return this;
-
}
-
public function multiply(n:*):Num{
-
n = convert(n);
-
value *= n;
-
return this;
-
}
-
public function divide(n:*):Num{
-
n = convert(n);
-
value /= n;
-
return this;
-
}
-
}
This snippet is meant to be run as a document class. It shows how one might go about designing a class to make extensive use of function chaining.
Also posted in OOP | Tagged actionscript, flash |
By Zevan | March 28, 2009
Actionscript:
-
var Pnt:Function = function(xp:Number, yp:Number){
-
-
var x:Number = xp;
-
var y:Number = yp
-
-
this.setX = function(v:int):void{
-
x = v;
-
}
-
this.setY = function(v:int):void{
-
y = v;
-
}
-
this.getX = function():int {
-
return x;
-
}
-
this.getY = function():int {
-
return y;
-
}
-
}
-
-
var p:Object = new Pnt(10,10);
-
-
trace(p.getX(), p.getY());
-
p.setX(100);
-
trace(p.getX());
-
-
/*
-
outputs:
-
10, 10
-
100
-
*/
Another way to define and instantiate Objects on the timeline. Interesting, but I don't recommend it over actual classes...
Also posted in OOP, Object | Tagged actionscript, flash |
By Zevan | March 27, 2009
Actionscript:
-
var multBy2Add3:Function = add(3, mult(2));
-
-
trace(multBy2Add3(10));
-
// 10 * 2 = 20
-
// 20 + 3 = 23
-
-
var add2AndSquare = sq(add(2));
-
-
trace(add2AndSquare(8));
-
// 8 + 2 = 10
-
// 10 * 10 = 100
-
-
var multBy2_3times:Function = repeat(3,mult(2));
-
-
trace(multBy2_3times(3));
-
// 3 * 2 = 6;
-
// 6 * 2 = 12;
-
// 12 * 2 = 24
-
-
// you can also chain for even less readability
-
trace(sq(mult(5,add(1)))(4));
-
// 4 + 1 = 5
-
// 5 * 5 = 25;
-
// 25 * 25 = 625;
-
-
/*
-
outputs:
-
12
-
100
-
24
-
625
-
*/
-
-
// function composition
-
const F:Function = function(a:*):*{return a};
-
function mult(scalar:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number){
-
return f(n) * scalar;
-
}
-
}
-
-
function add(off:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number){
-
return f(n) + off;
-
}
-
}
-
function sq(f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number){
-
var v:Number = f(n);
-
return v * v;
-
}
-
}
-
function repeat(times:int, f:Function):Function {
-
if (f == null) f = F;
-
return function (n:Number){
-
var v:Number = n;
-
for (var i:int = 0; i<times; i++) v = f(v);
-
return v;
-
}
-
}
The above shows some interesting function composition... this demo contains the following functions:
mult();
add();
sq();
repeat();
These functions are designed to be combined to create new functions.
Also posted in Math | Tagged actionscript, flash |
By Zevan | March 23, 2009
Actionscript:
-
[SWF(width=800, height=600)]
-
-
var canvas:Graphics;
-
var graphData:Array = sineData();
-
-
var graph0:Shape = Shape(addChild(new Shape()));
-
graph0.x = 50;
-
graph0.y = 150;
-
-
var graph1:Shape = Shape(addChild(new Shape()));
-
graph1.x = 400;
-
graph1.y = 150;
-
-
var graph2:Shape = Shape(addChild(new Shape()));
-
graph2.x = 50;
-
graph2.y = 400;
-
-
// use graphData to draw 3 different looking graphs:
-
-
canvas = graph0.graphics;
-
axis(lines(graphData));
-
-
canvas = graph1.graphics;
-
axis(dots(graphData, 0xFF0000), 0xFFCC00, 2);
-
-
canvas = graph2.graphics;
-
axis(dots(dots(lines(lines(graphData, 0xCCCCCC, 20))), 0x0022FF, 0, 4), 0xFF);
-
-
-
// generate data
-
function sineData():Array{
-
var dat:Array = new Array();
-
for (var i:int = 0; i<60; i++){
-
dat.push(new Point(i * 4, (30 + i) * Math.sin(i * 24 * Math.PI/180)));
-
}
-
return dat;
-
}
-
-
// render lines
-
function lines(dat:Array, col:uint=0x000000, thick:Number=0):Array{
-
canvas.lineStyle(thick, col);
-
canvas.moveTo(dat[0].x, dat[0].y)
-
for (var i:int = 1; i<dat.length; i++){
-
canvas.lineTo(dat[i].x, dat[i].y);
-
}
-
return dat;
-
}
-
-
// render dots
-
function dots(dat:Array, col:uint=0xFF0000, thick:Number=0, rad:Number=1.5):Array{
-
canvas.lineStyle(thick, col);
-
for (var i:int = 0; i<dat.length; i++){
-
canvas.drawCircle(dat[i].x, dat[i].y, rad);
-
}
-
return dat;
-
}
-
-
// render graph axis
-
function axis(dat:Array, col:uint=0x000000, thick:Number=0):Array{
-
var d:Array = dat.concat();
-
d.sortOn("y", Array.NUMERIC);
-
var lastIndex:int = d.length - 1;
-
var minY:Number = d[0].y;
-
var maxY:Number = d[lastIndex].y;
-
d.sortOn("x", Array.NUMERIC);
-
var minX:Number = d[0].x;
-
var maxX:Number = d[lastIndex].x;
-
canvas.lineStyle(thick, col, .2);
-
canvas.moveTo(minX, 0);
-
canvas.lineTo(maxX, 0);
-
canvas.lineStyle(thick, col);
-
canvas.moveTo(minX, minY);
-
canvas.lineTo(minX, maxY);
-
canvas.lineTo(maxX, maxY);
-
return dat;
-
}
This is something I've been meaning to post for awhile. Finally had time to write it today... It contains functions that are designed to be nested for the purpose of rendering a small data set in a few different ways...
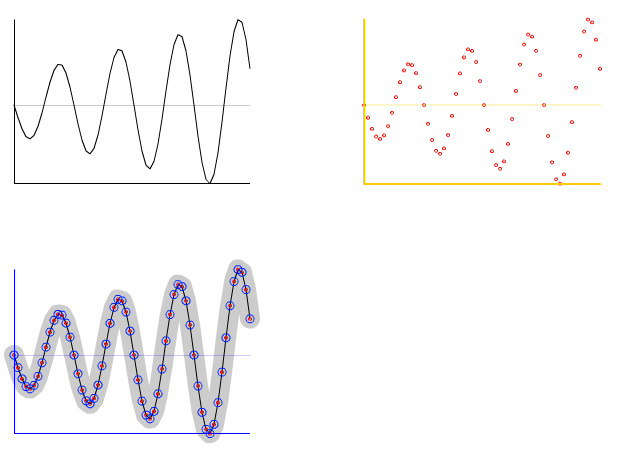
The upper left image is rendered with axis labels and lines... and it defaults to the color black (line 21):
axis(lines(graphData));
The upper right image is rendered with yellow axis and red dots (line 24):
axis(dots(graphData, 0xFF0000), 0xFFCC00, 2);
etc... (line 27)
axis(dots(dots(lines(lines(graphData, 0xCCCCCC, 20))), 0x0022FF, 0, 4), 0xFF);
Alternatively you could write each function call on one line:
lines(graphData, 0xCCCCCC, 20);
lines(graphData);
dots(graphData);
dots(graphData, 0x0022FF, 0, 4)
axis(graphData, 0xFF);
NOTE: If you think this post is insane, please read the warning page of this site...
Also posted in Graphics, misc | Tagged actionscript, flash |