By Zevan | March 12, 2010
Actionscript:
-
initOperators();
-
-
trace(zipWith("-", [1,2,3], [1,2,3]));
-
trace(zipWith("+", [1,2,3], [1,2,3]));
-
trace(zipWith("*", [1,2,3], [1,2,3]));
-
trace(zipWith("+", [1,1,1,3], [4,5,6,7]));
-
trace(zipWith("<<", [2, 4], [1,1]));
-
/*
-
outputs:
-
-
0,0,0
-
2,4,6
-
1,4,9
-
5,6,7,10
-
4,8
-
*/
-
-
function zipWith(op:String, a:Array, b:Array):Array{
-
var aLeng:int = a.length;
-
var bLeng:int = b.length;
-
var leng:Number = (aLeng <bLeng) ? aLeng : bLeng;
-
var zipped:Array = [];
-
-
if (!this[op])return [];
-
-
for (var i:int = 0; i<leng; i++){
-
zipped[i]=this[op](a[i], b[i]);
-
}
-
return zipped;
-
}
-
-
function initOperators():void{
-
this["+"]=function(a:Number, b:Number):Number{ return a + b };
-
this["-"]=function(a:Number, b:Number):Number{ return a - b };
-
this["/"]=function(a:Number, b:Number):Number{ return a / b };
-
this["*"]=function(a:Number, b:Number):Number{ return a * b };
-
this["%"]=function(a:Number, b:Number):Number{ return a % b };
-
-
this["&"]=function(a:Number, b:Number):Number{ return a & b };
-
this["<<"]=function(a:Number, b:Number):Number{ return a <<b };
-
this["|"]=function(a:Number, b:Number):Number{ return a | b };
-
this[">>"]=function(a:Number, b:Number):Number{ return a>> b };
-
this[">>>"]=function(a:Number, b:Number):Number{ return a>>> b };
-
this["^"]=function(a:Number, b:Number):Number{ return a ^ b };
-
}
This snippet is basically like the haskell zipWith() function. It can combines two arrays into one array given a single function. In this case I defined a bunch of operator functions, but it would work with any kind of function that takes two arguments and returns a value. You could extend this to work with strings and do other strange things I guess.
If you have yet to go play with haskell ... go do it now.
By Zevan | February 19, 2009
Actionscript:
-
// best thing about this are the random seeds
-
var s1:Number= 0xFFFFFF;
-
var s2:Number = 0xCCCCCC;
-
var s3:Number= 0xFF00FF;
-
-
// saw this algorithm on this somewhat annoying thread:
-
// http://www.reddit.com/r/programming/comments/7yjlc/why_you_should_never_use_rand_plus_alternative/
-
// additional googling brought me to this: http://wakaba.c3.cx/soc/kareha.pl/1100499906/
-
// and finally to this ( didn't understand most of this one) www.ams.org/mcom/1996-65-213/S0025-5718-96-00696-5/S0025-5718-96-00696-5.pdf
-
-
function rand():Number {
-
s1=((s1&4294967294)<<12)^(((s1<<13)^s1)>>19);
-
s2=((s2&4294967288)<<4)^(((s2<<2)^s2)>>25);
-
s3=((s3&4294967280)<<17)^(((s3<<3)^s3)>>11);
-
var r:Number = (s1^s2^s3) * 2.3283064365e-10;
-
r = (r<0) ? r+=1 : r;
-
return r;
-
}
-
-
// see a visual comparison between this and actionscript's Math.random()
-
-
var canvas:BitmapData = new BitmapData(400,400,false, 0xCCCCCC);
-
addChild(new Bitmap(canvas));
-
-
var posX:Number;
-
var posY:Number;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function onLoop(evt:Event):void {
-
-
for (var i:int = 0; i<100; i++){
-
posX = rand() * 180
-
posY = rand() * 180
-
canvas.setPixel( 100 + posX, 10 + posY, 0x000000);
-
}
-
-
for (i = 0; i<100; i++){
-
posX = Math.random() * 180
-
posY = Math.random() * 180
-
canvas.setPixel( 100 + posX, 210 + posY, 0x000000);
-
}
-
}
The above snippet demo's an alternative random number generator that uses the Tausworthe method.
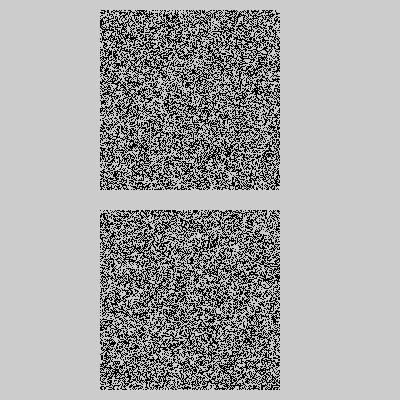
Top square is Tausworthe and bottom is Math.random()
I don't actually know much about this, or fully understand how it works. I just saw it on a random reddit thread, googled about it for a few minutes and then ported it to actionscript.
There's lots of talk about what the BEST random number generator is.... which one is the fastest, which one is the most random. etc... I don't really know much about that, especially since I'm just playing around with code and I don't need a RNG for some scientific experiment. For me, what's nice about this approach is the three random seeds. For some reason, Math.random() doesn't have a place where you can enter a seed for random numbers. Random seeds are very useful when you want to do something random but want to be able to replicate the random results at a later time. For instance:
Actionscript:
-
var s1:Number= 0xFFFFFF;
-
var s2:Number = 0xCCCCCC;
-
var s3:Number= 0xFF00FF;
-
-
trace("first three values:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
s1 = 0xFF;
-
s2 = 0xEFEFEF;
-
s3 = 19008;
-
-
trace("\nfirst three values with different seeds:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
s1 = 0xFFFFFF;
-
s2 = 0xCCCCCC;
-
s3 = 0xFF00FF;
-
-
trace("\noriginal three values:");
-
trace(rand());
-
trace(rand())
-
trace(rand())
-
-
function rand():Number {
-
s1=((s1&4294967294)<<12)^(((s1<<13)^s1)>>19);
-
s2=((s2&4294967288)<<4)^(((s2<<2)^s2)>>25);
-
s3=((s3&4294967280)<<17)^(((s3<<3)^s3)>>11);
-
var r:Number = (s1^s2^s3) * 2.3283064365e-10;
-
r = (r<0) ? r+=1 : r;
-
return r;
-
}
-
-
/*
-
outputs:
-
first three values:
-
0.051455706589931385
-
0.050584114155822715
-
0.417276361484596
-
-
first three values with different seeds:
-
0.6032885762463134
-
0.9319786790304015
-
0.8631882804934321
-
-
original three values:
-
0.051455706589931385
-
0.050584114155822715
-
0.417276361484596
-
*/
I stumbled upon a bunch of other random number algorithms, maybe I'll throw them in a class in the next couple days.
Also posted in misc | Tagged actionscript, flash |
By Zevan | January 8, 2009
Actionscript:
-
// tracing out large numbers will go into exponent notation
-
trace(1000000000000000000000000);
-
// outputs: 1e+24
-
-
var a:String = "1000000000000000000000009";
-
var b:String = "12000000000000000000000001";
-
-
// bigAdd function adds two large numbers
-
trace(bigAdd(a, b));
-
// outputs: 13000000000000000000000010
-
-
// unclear what regular addition is doing here:
-
trace(int(a) + int(b));
-
// outputs: -3758096384
-
-
function bigAdd(a:String, b:String):String{
-
var answer:String="";
-
var carry:int = 0;
-
var zeros:int = 0;
-
var i:int = 0;
-
var max:int = 0;
-
-
// obtain max variable and prepend zeros to the shortest string
-
if (a.length> b.length){
-
max = a.length;
-
zeros = max - b.length;
-
for (i= 0; i<zeros; i++) {
-
b = "0" + b;
-
}
-
}else if (b.length> a.length){
-
max = b.length;
-
zeros = max - a.length;
-
for (i= 0; i<zeros; i++) {
-
a = "0" + a ;
-
}
-
}else{
-
// a and b have same number of digets
-
max = a.length;
-
}
-
-
// add each character starting with the last (max - 1),
-
// carry the 1 if the sum has a length of 2
-
for (i = max - 1; i> -1; i--){
-
var sum:String = String(int(a.charAt(i)) + int(b.charAt(i)) + carry);
-
-
if (sum.length == 2){
-
answer = sum.charAt(1) + answer;
-
carry = 1;
-
}else{
-
carry = 0;
-
answer = sum + answer;
-
}
-
}
-
if (carry == 1){
-
answer = 1 + answer;
-
}
-
return answer;
-
}
This snippet demos a function called bigAdd(), which can be used to add very large numbers that the standard numerical datatypes (int, Number, etc) won't really work with. It uses a string based addition algorithm.
When I decided to write this snippet I dug around for awhile trying to find a nice elegant algorithm to port, something done without prepending zeros etc.... but I couldn't find one that suited my needs... so I just created this one from scratch. There's room for optimization and improvement but it works well enough for just playing around.
BigDecimal & BigInteger
A few months back I needed to do some programming with very large numbers. I dug around for awhile and eventually found out that java has a built in BigInteger andBigDecimal class.... I used java to write the program I had in mind, but thought it would be fun to eventually create something similar for ActionScript....
By Zevan | November 10, 2008
Actionscript:
-
for(var i:int = 2; i<37; i++){
-
trace((10000).toString(i));
-
}
-
/*
-
outputs:
-
10011100010000
-
111201101
-
2130100
-
310000
-
114144
-
41104
-
23420
-
14641
-
10000
-
7571
-
5954
-
4723
-
3904
-
2e6a
-
2710
-
20a4
-
1cfa
-
18d6
-
1500
-
11e4
-
kec
-
iki
-
h8g
-
g00
-
ekg
-
dja
-
cl4
-
bpo
-
b3a
-
aci
-
9og
-
961
-
8m4
-
85p
-
7ps
-
*/
I had either totally forgotten about or never known about the Number, uint, int etc... .toString() radix argument. Brock mentioned it to me in conversation and I've been having fun with it ever since. It's especially nice for tracing out hex numbers:
Actionscript:
-
var red:Number = 0xFF0000;
-
trace("decimal:");
-
trace(red);
-
trace("hex:");
-
trace(red.toString(16));
Also posted in strings | Tagged decimal, hex, toString |