By Zevan | August 15, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Common.Math.*;
-
-
[SWF (backgroundColor=0x000000, width=700, height=600, frameRate=60)]
-
-
var sim:QuickBox2D = new QuickBox2D(this);
-
-
sim.setDefault({fillColor:0xCCCCCC, lineColor:0x3355AA});
-
-
sim.createStageWalls();
-
-
var box:QuickObject = sim.addBox({x:10, y:10, density:0});
-
var circle:QuickObject = sim.addCircle({x:10, y:13});
-
-
sim.addJoint({type:"prismatic", a:box.body, b:circle.body, axis:new b2Vec2(1, 0), upperTranslation:3, lowerTranslation:-3, enableLimit:true, motorSpeed:10, maxMotorForce:10, enableMotor:true});
-
-
sim.start();
-
sim.mouseDrag();
Simple prismatic joint demo. Prismatic joints are odd, it took me awhile to realize how to use them and what they can be used for... Plan on using them in the creation of some Box2D machines in the near futrure...
Check out the swf
By Zevan | August 14, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Common.Math.*;
-
-
[SWF (backgroundColor=0x000000, width=700, height=600)]
-
-
var sim:QuickBox2D = new QuickBox2D(this,{gravityY:10});
-
-
sim.setDefault({fillColor:0x113366, fillAlpha:0.8, lineColor:0x3355AA});
-
sim.createStageWalls();
-
-
var boxA:QuickObject = sim.addBox({x:10, y:8, width:4, height:0.5, density:10});
-
var boxB:QuickObject = sim.addBox({x:5, y:5, density:10});
-
boxB.body.SetLinearVelocity(new b2Vec2(5, 0));
-
-
var rev1:QuickObject = sim.addJoint({type:"revolute", a:sim.w.GetGroundBody(), b:boxA.body, anchor:boxA.body.GetWorldCenter()})
-
-
var rev2:QuickObject = sim.addJoint({type:"prismatic", a:sim.w.GetGroundBody(), axis:new b2Vec2(1, 0), b:boxB.body, anchor:boxB.body.GetWorldCenter()})
-
-
sim.addJoint({type:"gear", a:boxA.body, b:boxB.body, joint1:rev1.joint, joint2:rev2.joint});
-
-
sim.start();
-
sim.mouseDrag();
Simple gear joint demo - meant to serve as a reference for QuickBox2D...
Have a look at the swf...
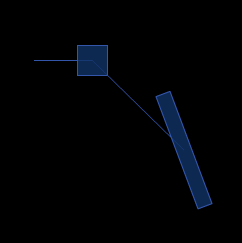
By Zevan | August 13, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
-
[SWF (backgroundColor=0x000000, width=700, height=600)]
-
-
var sim:QuickBox2D = new QuickBox2D(this);
-
-
sim.setDefault({fillColor:0x113366, fillAlpha:0.8, lineColor:0x3355AA});
-
-
sim.createStageWalls();
-
-
var boxA:QuickObject = sim.addBox({x:10, y:8, width:4, height:0.5, angularDamping:1});
-
var boxB:QuickObject = sim.addBox({x:7, y:8, width:3, height:0.25, angle:-.5, angularDamping:1});
-
var boxC:QuickObject = sim.addBox({x:12, y:2, width:2, height:1});
-
-
var rev:QuickObject = sim.addJoint({type:"revolute", a:boxA.body, b:sim.w.GetGroundBody()});
-
// add a red dot to boxA
-
with (boxA.userData.graphics) lineStyle(), beginFill(0xFF0000), drawCircle(0,0,2);
-
-
var rev2:QuickObject = sim.addJoint({type:"revolute", a:boxB.body, b:sim.w.GetGroundBody()});
-
with (boxB.userData.graphics) lineStyle(), beginFill(0xFF0000), drawCircle(0,0,2);
-
-
sim.start();
-
sim.mouseDrag();
In order to use gear joints (covered in tomorrows post) you'll need to know how to connect to the ground body...
Check out the swf...
By Zevan | August 12, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Dynamics.Joints.*;
-
-
[SWF (backgroundColor=0x222222, width=700, height=600)]
-
-
var sim:QuickBox2D = new QuickBox2D(this);
-
-
sim.setDefault({fillColor:0x000000, lineColor:0xCCCCCC});
-
sim.createStageWalls();
-
-
var legA:QuickObject = sim.addBox({x:5, y:11, width:2, height:0.2,groupIndex:-2});
-
var three:QuickObject = sim.addBox({x:6.8 + 1.8, y:11, width:2, height:0.2,groupIndex:-2});
-
var legB:QuickObject = sim.addBox({x:6.8, y:11, width:2, height:0.5,groupIndex:-2});
-
sim.setDefault({type:"revolute"});
-
-
var anchorX:Number = legA.x + (legB.x - legA.x) / 2;
-
var anchorY:Number = legA.y;
-
var revJointA:QuickObject = sim.addJoint({a:legA.body, b:legB.body, x1:anchorX, y1:anchorY,enableMotor:true, maxMotorTorque:80});
-
-
anchorX = legB.x + (three.x - legB.x) / 2;
-
-
var revJointB:QuickObject = sim.addJoint({a:legB.body, b:three.body, x1:anchorX, y1:anchorY, enableMotor:true, maxMotorTorque:80});
-
-
setWalkDir(6);
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
if (legB.x <4){
-
setWalkDir(6);
-
}else if (legB.x> 19){
-
setWalkDir(-6);
-
}
-
}
-
function setWalkDir(dir:Number):void{
-
var j:b2RevoluteJoint;
-
j = revJointA.joint as b2RevoluteJoint
-
j.SetMotorSpeed(dir);
-
j = revJointB.joint as b2RevoluteJoint
-
j.SetMotorSpeed(dir * -1);
-
}
-
-
sim.start();
-
sim.mouseDrag();
This demo is a bit more complex than the last two - mostly because it makes use of motors...
Have a look at the swf...
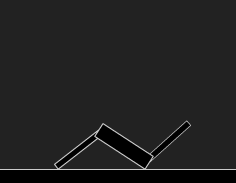