By Zevan | March 19, 2010
Actionscript:
-
[SWF(width = 500, height = 500)]
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = stage.stageWidth / 2;
-
var centerY:Number = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
// data
-
var points:Array = [];
-
var i:int = 0;
-
var pointNum : int = Math.max(2,int(mouseX / 12))
-
-
var radius:Number = 200;
-
var step:Number = TWO_PI / pointNum;
-
var theta:Number = step / 2;
-
for (i = 0; i<pointNum; i++){
-
var xp:Number = centerX + radius * Math.cos(theta);
-
var yp:Number = centerY + radius * Math.sin(theta);
-
points[i] = new Point(xp, yp);
-
theta += step;
-
}
-
// render
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for ( i = 0; i<pointNum; i++){
-
var a:Point = points[i];
-
for (var j:int = 0; j<pointNum; j++){
-
var b:Point = points[j];
-
if (a != b){
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
}
-
}
I've been using this geometric shape for lots of different things recently. Including during consulting gigs as a helpful visualization. Just move your mouse left and right... I particularly like the simpler forms you get by moving your mouse to the left (triangles squares and simple polygons):
While not entirely related this wikipedia article is interesting.
[EDIT : Thanks to martin for reminding me that I can do away with the if statement here in the above code ]
Actionscript:
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (i = 0; i<pointNum; i++) {
-
var a:Point=points[i];
-
for (var j:int = i+1; j<pointNum; j++) {
-
var b:Point=points[j];
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
graphics.drawCircle(a.x, a.y, 10);
I implemented that change over at wonderfl and it works nicely
Posted in Graphics, Math, misc | Also tagged as3, flash |
By Zevan | March 15, 2010
Actionscript:
-
[SWF(width = 500, height=500, backgroundColor=0x000000)]
-
-
var clockNum:int = 100;
-
var clocks:Vector.<Function> = new Vector.<Function>(clockNum, true);
-
-
var clockContainer:Sprite = Sprite(addChild(new Sprite()));
-
clockContainer.x = stage.stageWidth / 2;
-
clockContainer.y = stage.stageHeight / 2;
-
buildClocks();
-
runClocks();
-
-
function buildClocks():void{
-
for (var i:int = 0; i<clockNum; i++){
-
var theta:Number = Math.random() * Math.PI * 2;
-
var radius:Number = Math.random() * 200;
-
var xp:Number = radius * Math.cos(theta);
-
var yp:Number = radius * Math.sin(theta);
-
clocks[i] = makeClock(xp,yp,Math.random() * Math.PI * 2);
-
}
-
}
-
function runClocks():void{
-
addEventListener(Event.ENTER_FRAME, onRunClocks);
-
}
-
function onRunClocks(evt:Event):void{
-
for (var i:int = 0; i<clockNum; i++){
-
clocks[i]();
-
}
-
clockContainer.rotationX = clockContainer.mouseY / 30;
-
clockContainer.rotationY = -clockContainer.mouseX / 30;
-
}
-
function makeClock(x:Number, y:Number, time:Number=0):Function{
-
var radius:Number = Math.random() * 20 + 5;
-
var border:Number = radius * 0.2;
-
var smallRadius:Number = radius - radius * 0.3;
-
-
var clock:Sprite = Sprite(clockContainer.addChild(new Sprite()));
-
clock.x = x;
-
clock.y = y;
-
clock.z = 100 - Math.random() * 200;
-
clock.rotationX = Math.random() * 40 - 20;
-
clock.rotationY = Math.random() * 40 - 20;
-
clock.rotationZ = Math.random() * 360;
-
return function():void{
-
with (clock.graphics){
-
clear();
-
lineStyle(1,0xFFFFFF);
-
drawCircle(0,0,radius + border);
-
var xp:Number = smallRadius * Math.cos(time/2);
-
var yp:Number = smallRadius * Math.sin(time/2);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
xp = radius * Math.cos(time);
-
yp = radius * Math.sin(time);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
}
-
time+=0.1;
-
}
-
}
You can go check the swf out at wonderfl.net...
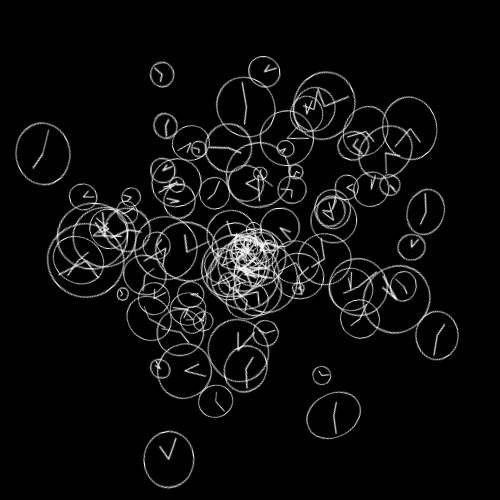
Posted in 3D, Graphics, misc, motion | Also tagged as3, flash |
By Zevan | March 14, 2010
Here is a quick sunday quiz. Create some kind of clock with as little code as possible. Even just a textfield and the date object would cut it, but preferably try to do something a little more interesting. Post your results in the comments. I'll post my version on monday EOD.
[EDIT: my clock can be found here which I'll count as tomorrows post]
The last clock post I did was about the 24 hour clock have a look at that here.
Posted in Quiz | Also tagged as3, flash, Quiz |
By Zevan | March 12, 2010
Actionscript:
-
initOperators();
-
-
trace(zipWith("-", [1,2,3], [1,2,3]));
-
trace(zipWith("+", [1,2,3], [1,2,3]));
-
trace(zipWith("*", [1,2,3], [1,2,3]));
-
trace(zipWith("+", [1,1,1,3], [4,5,6,7]));
-
trace(zipWith("<<", [2, 4], [1,1]));
-
/*
-
outputs:
-
-
0,0,0
-
2,4,6
-
1,4,9
-
5,6,7,10
-
4,8
-
*/
-
-
function zipWith(op:String, a:Array, b:Array):Array{
-
var aLeng:int = a.length;
-
var bLeng:int = b.length;
-
var leng:Number = (aLeng <bLeng) ? aLeng : bLeng;
-
var zipped:Array = [];
-
-
if (!this[op])return [];
-
-
for (var i:int = 0; i<leng; i++){
-
zipped[i]=this[op](a[i], b[i]);
-
}
-
return zipped;
-
}
-
-
function initOperators():void{
-
this["+"]=function(a:Number, b:Number):Number{ return a + b };
-
this["-"]=function(a:Number, b:Number):Number{ return a - b };
-
this["/"]=function(a:Number, b:Number):Number{ return a / b };
-
this["*"]=function(a:Number, b:Number):Number{ return a * b };
-
this["%"]=function(a:Number, b:Number):Number{ return a % b };
-
-
this["&"]=function(a:Number, b:Number):Number{ return a & b };
-
this["<<"]=function(a:Number, b:Number):Number{ return a <<b };
-
this["|"]=function(a:Number, b:Number):Number{ return a | b };
-
this[">>"]=function(a:Number, b:Number):Number{ return a>> b };
-
this[">>>"]=function(a:Number, b:Number):Number{ return a>>> b };
-
this["^"]=function(a:Number, b:Number):Number{ return a ^ b };
-
}
This snippet is basically like the haskell zipWith() function. It can combines two arrays into one array given a single function. In this case I defined a bunch of operator functions, but it would work with any kind of function that takes two arguments and returns a value. You could extend this to work with strings and do other strange things I guess.
If you have yet to go play with haskell ... go do it now.