Actionscript:
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.autoSize = TextFieldAutoSize.LEFT;
-
txt.x = txt.y = 20;
-
txt.text = "click anywhere to load an image file...";
-
-
var fileRef:FileReference= new FileReference();
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
function onDown(evt:MouseEvent):void{
-
fileRef.browse([new FileFilter("Images", "*.jpg;*.gif;*.png")]);
-
fileRef.addEventListener(Event.SELECT, onSelected);
-
stage.removeEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
}
-
function onSelected(evt:Event):void{
-
fileRef.addEventListener(Event.COMPLETE, onLoaded);
-
fileRef.load();
-
fileRef.removeEventListener(Event.SELECT, onSelected);
-
}
-
function onLoaded(evt:Event):void{
-
var loader:Loader = new Loader();
-
loader.loadBytes(evt.target.data);
-
addChild(loader);
-
fileRef.removeEventListener(Event.COMPLETE, onLoaded);
-
}
This snippet shows how to use the FileReference.load() method to load an image into flash player RAM and display it on the stage.
Posted in external data, misc | Also tagged as3, flash |
Actionscript:
-
var counter:Function = function(count:Array):Function{
-
var leng:int = count.length;
-
var index:int = 0;
-
return counter = function(reset:*=""):Function{
-
if (reset=="reset"){
-
index = 0;
-
return counter;
-
}else
-
if (reset is Array){
-
count = reset;
-
return counter;
-
}
-
trace(count[index % leng]);
-
index++;
-
return counter;
-
}
-
}
-
-
// first time it's called you pass an array
-
trace("count through the array:");
-
// you can optionally call trigger the counter by calling the newly
-
// returned function
-
counter(["a","b","c"])();
-
counter();
-
counter();
-
trace("change the array:");
-
// here we choose to simply set the array and not trigger the counter
-
counter([10,20,40,60,80]);
-
counter();
-
counter()
-
trace("reset the counter:");
-
// reset and the counter is called 3 times
-
counter("reset")()()();
-
-
/*outputs :
-
count through the array:
-
a
-
b
-
c
-
change the array:
-
10
-
20
-
reset the counter:
-
10
-
20
-
40
-
*/
Today I felt like messing around with functions and this is the result. This snippet shows a strange and interesting technique to create a function that iterates through a given array in a few different ways.
I believe the technical term for this kind of thing is a continuation...
There are a bunch of other posts like this on actionsnippet. Some are purely experimental, others are actually quite useful... these can all be found in the functions category.
Posted in dynamic, functions | Also tagged as3, flash |
Actionscript:
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight,false, 0xCCCCCC);
-
addChild(new Bitmap(canvas));
-
var prevX:Number;
-
var prevY:Number;
-
var brush:Shape = new Shape();
-
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
stage.addEventListener(MouseEvent.MOUSE_UP, onUp);
-
function onDown(evt:MouseEvent):void{
-
prevX = mouseX;
-
prevY = mouseY;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
}
-
-
function onUp(evt:MouseEvent):void{
-
removeEventListener(Event.ENTER_FRAME, onLoop);
-
}
-
-
function onLoop(evt:Event):void {
-
brush.x = mouseX;
-
brush.y = mouseY;
-
with (brush.graphics){
-
clear();
-
lineStyle(3, 0x000000);
-
lineTo(prevX-mouseX, prevY-mouseY);
-
}
-
canvas.draw(brush, brush.transform.matrix);
-
prevX = mouseX;
-
prevY = mouseY;
-
}
If you write a drawing program with the Graphics class alone, you'll notice that eventually the flash player will start to slow down. This could take quite some time, but it will eventually happen. Because the Graphics class is entirely vector based, the flash player needs to keep track of every single point that makes up any line in your drawing. If you draw something and then erase it by drawing white vector lines over it, flash still needs to know about that thing that you erased.
The snippet I wrote yesterday reminded me of the technique used in the above snippet. In yesterdays post every line is drawn using the graphics class. As a result, eventually the flash player begins to choke.
Today's snippet draws a vector between the previous mouse location and the current mouse location - this vector is then drawn onto a BitmapData object and the vector is cleared. So rather than creating vector graphics that are continuously increasing in complexity, your just changing the color of pixels around on a BitmapData.
To see a visual explanation take a look at the below swf. I slowed it down to 5fps, I tinted the vector red and scaled up the stage so you can differentiate between what's vector and what's bitmap:
Click to see the swf...
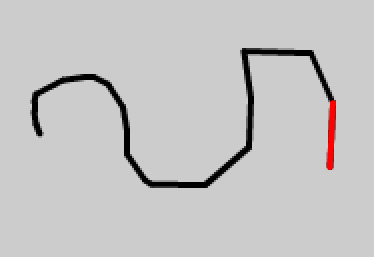
Posted in BitmapData, Graphics | Also tagged as3, flash |
Actionscript:
-
var canvas:Shape = Shape(addChild(new Shape()));
-
var gestures:Array=[];
-
var gestureNum:int = 0;
-
var capGesture:Array;
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
stage.addEventListener(MouseEvent.MOUSE_UP, onUp);
-
function onDown(evt:MouseEvent):void{
-
capGesture=[];
-
addEventListener(Event.ENTER_FRAME, onCapture);
-
-
canvas.graphics.lineStyle(3, 0xFF0000);
-
canvas.x = mouseX;
-
canvas.y = mouseY;
-
canvas.graphics.moveTo(0, 0);
-
}
-
function onUp(evt:MouseEvent):void{
-
gestures.push(capGesture.concat());
-
gestureNum++;
-
canvas.graphics.clear();
-
removeEventListener(Event.ENTER_FRAME, onCapture);
-
}
-
function onCapture(evt:Event):void{
-
capGesture.push(new Point(canvas.mouseX, canvas.mouseY));
-
canvas.graphics.lineTo(canvas.mouseX, canvas.mouseY);
-
}
-
-
var currGesture:Array;
-
var drawing:Boolean = false;
-
var lineThickness:Number = 0;
-
var lineColor:Number = 0x000000;
-
var index:int = 0;
-
var pnt:Point;
-
var trans:Matrix = new Matrix();
-
var i:int
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
if (gestureNum> 0){
-
if (!drawing){
-
currGesture = gestures[int(Math.random() * gestureNum)].concat();
-
trans.identity();
-
trans.rotate(Math.random()*6.28);
-
var scale:Number = Math.random() * 2 + .1;
-
trans.scale(scale, scale);
-
trans.tx = Math.random() * stage.stageWidth
-
trans.ty = Math.random() * stage.stageHeight
-
for (i = 0; i<currGesture.length; i++){
-
currGesture[i] = trans.transformPoint(currGesture[i]);
-
}
-
lineThickness = Math.random() * Math.random() * 50;
-
if (int(Math.random()*10) ==1){
-
var col:uint = uint(Math.random()*255);
-
lineColor = col <<16 | col <<8 | col;
-
}
-
index = 0;
-
drawing = true;
-
graphics.lineStyle(lineThickness, lineColor);
-
}else{
-
for (i = 0; i<10; i++){
-
if (drawing == true){
-
pnt = currGesture[index];
-
if (index == 0){
-
graphics.moveTo(pnt.x, pnt.y);
-
}else{
-
graphics.lineTo(pnt.x, pnt.y);
-
}
-
index++;
-
if (index == currGesture.length){
-
drawing = false;
-
}
-
}
-
}
-
}
-
}
-
}
This snippet is an idea I have been meaning to try for sometime. It's a mini-drawing program. You can draw single gestures (shapes, letters etc...) and the program then randomly scales, rotates, tints and translates these gestures repeatedly on the canvas. You can continue to draw as it does this, the more gestures you draw, the more the program will have to randomly choose from.
Have a look at the swf here...
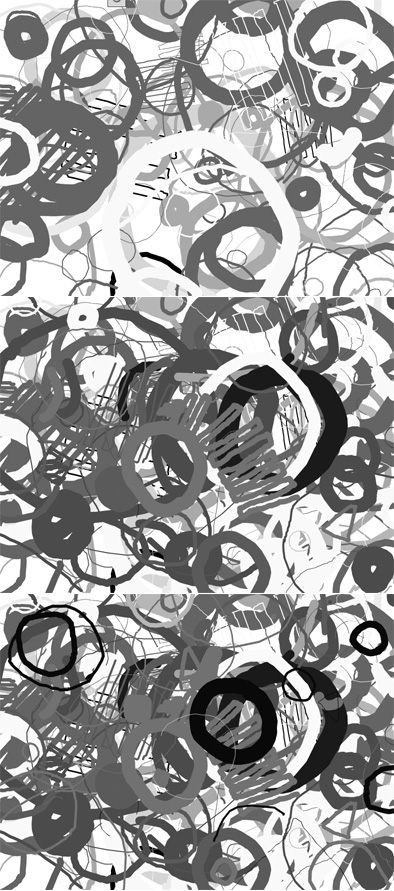
Posted in Graphics, misc, motion | Also tagged as3, flash |