By Zevan | November 27, 2009
Actionscript:
-
egyptianMultiply(12, 99);
-
-
// trace(12 * 99) // test to make sure it works
-
-
/* outputs:
-
/64 768
-
/32 384
-
16 192
-
8 96
-
4 48
-
/2 24
-
/1 12
-
---
-
1188
-
*/
-
-
-
function egyptianMultiply(valueA:Number, valueB:Number):void {
-
-
var left:Array = [];
-
var right:Array = []
-
-
// swap if valueB is smaller than value A
-
if (valueB <valueA){
-
var swap:int = valueB;
-
valueB = valueA;
-
valueA = swap;
-
}
-
-
// create left and right columns
-
var currentLeft:int = 1;
-
var currentRight:int = valueA;
-
-
while (currentLeft <valueB){
-
left.push(currentLeft);
-
right.push(currentRight);
-
currentRight += currentRight;
-
currentLeft += currentLeft;
-
}
-
-
-
// add up the right column based on the left
-
currentLeft = 0;
-
var rightSum:int;
-
var leftSum:int;
-
var i:int = left.length - 1;
-
-
while (currentLeft != valueB){
-
-
leftSum = currentLeft + left[i];
-
// if the next number causes the sum
-
// to go above valueB, skip it
-
if (leftSum <= valueB){
-
currentLeft = leftSum;
-
rightSum += right[i];
-
trace("/" + left[i] + " " + right[i]);
-
} else {
-
trace(" " + left[i] + " " + right[i]);
-
}
-
i--;
-
}
-
trace("---");
-
trace(rightSum);
-
}
Someone mentioned egyptian multiplication to me yesterday... So read a little about it here and whipped up this example. For some reason I decided to do it in processing ... once it worked I ported it to ActionScript.
The above link describing the technique I used is from http://www.jimloy.com/... I haven't spent much time digging around the site, but it appears to have some pretty nice stuff on it...
If you're curious, here is the original processing version:
JAVA:
-
-
-
-
int valueA = 10;
-
int valueB = 8;
-
-
if (valueB <valueA){
-
int swap = valueB;
-
valueB = valueA;
-
valueA = swap;
-
}
-
-
int currentLeft = 1;
-
int currentRight = valueA;
-
while (currentLeft <valueB){
-
left.add(currentLeft);
-
right.add(currentRight);
-
currentRight += currentRight;
-
currentLeft += currentLeft;
-
}
-
-
currentLeft = 0;
-
-
int result = 0;
-
int i = left.size() - 1;
-
while (currentLeft != valueB){
-
-
int temp
= currentLeft
+ (Integer) left.
get(i
);
-
if (temp <= valueB){
-
-
currentLeft = temp;
-
-
println("/" + left.get(i) + " " + right.get(i));
-
} else {
-
println(" " + left.get(i) + " " + right.get(i));
-
}
-
-
i--;
-
}
-
println("---");
-
println(result);
After writing, this I took a look at the wikipedia entry... I also found myself on this short page about a scribe called Ahmes. (I recommend reading this if you are interested in PI)
Posted in Math, misc | Also tagged as3, flash, processing |
By Zevan | November 25, 2009
Actionscript:
-
var container:Sprite = new Sprite();
-
container.x = stage.stageWidth / 2;
-
container.y = stage.stageHeight / 2;
-
addChild(container);
-
-
var redBox:Sprite = new Sprite();
-
redBox.graphics.beginFill(0xFF0000);
-
redBox.graphics.drawRect(-50,-250,100,500);
-
redBox.rotationZ = 10;
-
container.addChild(redBox);
-
-
var logos:Array = []
-
var elements:Array = [];
-
elements.push({element:redBox, z:0});
-
-
// add the logos
-
for (var i:int = 0; i<6; i++){
-
var logoContainer:MovieClip = new MovieClip();
-
var logoText:TextField = new TextField();
-
logoText.defaultTextFormat = new TextFormat("_sans", 50);
-
logoText.text = "LOGO";
-
logoText.autoSize = "left";
-
logoText.selectable= false;
-
-
logoText.x = -logoText.width / 2;
-
logoText.y = -logoText.height / 2;
-
logoContainer.addChild(logoText);
-
logoText.backgroundColor = 0xFFFFFF;
-
-
container.addChild(logoContainer);
-
logos.push(logoContainer);
-
elements.push({element:logoContainer, z:0});
-
}
-
-
var ang:Number = -Math.PI / 2;
-
var rotationSpeed:Number = 0.05;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
var dx:Number = (mouseY - stage.stageHeight / 2) / 10;
-
var dy:Number = (mouseX - stage.stageWidth / 2) / 10;
-
container.rotationX += (dx - container.rotationX) / 4;
-
container.rotationY += (dy - container.rotationY) / 4;
-
-
ang += rotationSpeed;
-
for (var i:int = 0; i<logos.length; i++){
-
var logo:Sprite = logos[i];
-
logo.x = 150 * Math.cos(ang + i);
-
logo.z = 150 * Math.sin(ang + i);
-
logo.alpha = 1 - logo.z / 200;
-
logo.rotationY = -Math.atan2(logo.z, logo.x) / Math.PI * 180 - 90;
-
}
-
-
// z-sort
-
for (i = 0; i<elements.length; i++){
-
elements[i].z = elements[i].element.transform.getRelativeMatrix3D(this).position.z;
-
}
-
-
elements.sortOn("z", Array.NUMERIC | Array.DESCENDING);
-
for (i = 0; i<elements.length; i++) {
-
container.addChild(elements[i].element);
-
}
-
}
A student of mine was having trouble creating a 3D logo for a client. I created this snippet to help explain how some of the fp10 3D stuff works.... z-sorting etc... The code could be optimized a bit... but it works nicely...
Have a look at the swf...
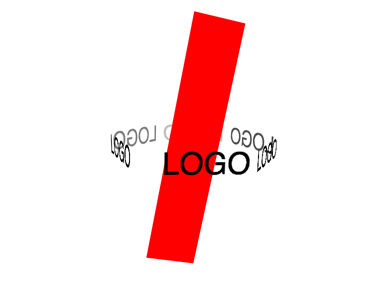
Posted in 3D, misc, motion | Also tagged as3, flash, fp10 |
By Zevan | November 23, 2009
Actionscript:
-
var connect:Function = function(xp:Number, yp:Number, col:uint=0):Function{
-
graphics.lineStyle(0,col);
-
graphics.moveTo(xp, yp);
-
var line:Function = function(xp:Number, yp:Number):Function{
-
graphics.lineTo(xp, yp);
-
return line;
-
}
-
return line;
-
}
-
-
// draw a triangle
-
connect(200,100)(300,300)(100,300)(200, 100);
-
-
// draw a box
-
connect(100,100, 0xFF0000)(150,100)(150,150)(100, 150)(100,100);
This is one of those techniques that I never really get tired of. It's pretty useless, but fun to play around with every now and then. This draws the somewhat boring looking picture below:
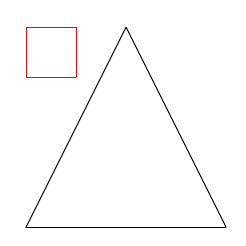
[EDIT]
A few people pointed out that this could be simplified with arguments.callee... So here is an example... it does the same thing as the original code...
Actionscript:
-
var connect:Function = function(xp:Number, yp:Number, col:uint=0):Function{
-
graphics.lineStyle(0,col);
-
graphics.moveTo(xp, yp);
-
return function(xp:Number, yp:Number):Function{
-
graphics.lineTo(xp, yp);
-
return arguments.callee;
-
}
-
}
Posted in functions, misc | Also tagged as3, flash |
By Zevan | November 18, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Dynamics.*
-
-
stage.frameRate = 60;
-
-
var sim:QuickBox2D = new QuickBox2D(this, {debug:true});
-
-
// get at the b2DebugDraw instance
-
var debug:b2DebugDraw = sim.w.m_debugDraw;
-
debug.m_drawScale = 30.0;
-
debug.m_fillAlpha = 0.5;
-
debug.m_alpha = 0.5;
-
debug.m_lineThickness = 1.0;
-
debug.m_drawFlags = 0xFF;
-
-
sim.createStageWalls();
-
-
for (var i:int = 0; i<10; i++){
-
sim.addBox({x:3 + i, y:3 + i, width:2, height:0.5});
-
}
-
sim.addCircle({x:12, y:5, radius:2});
-
-
sim.start();
-
sim.mouseDrag();
Note: This snippet requires the QuickBox2D library
This snippet shows an easy way to get at the settings for Box2D's debug renderer.
Have a look at the swf...
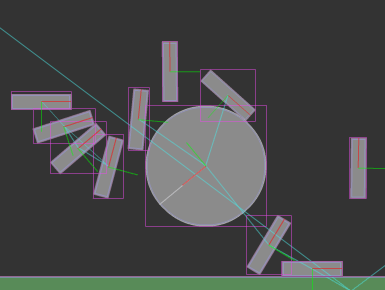