By Zevan | December 11, 2009
Actionscript:
-
[SWF(width = 700, height=700, frameRate=12)]
-
var cmds:Array = [];
-
-
var loader:URLLoader = new URLLoader();
-
var req:URLRequest = new URLRequest("http://search.twitter.com/search.atom");
-
var vars:URLVariables = new URLVariables();
-
vars.q = "#asgraph";
-
// results per page
-
vars.rpp = "100";
-
vars.page = 1;
-
-
req.data = vars;
-
req.method = URLRequestMethod.GET;
-
-
loader.addEventListener(Event.COMPLETE, onLoaded);
-
loader.load(req);
-
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.defaultTextFormat = new TextFormat("_sans", 12);
-
with (txt){ x=10, y=10, autoSize="left"; }
-
txt.htmlText = "loading...";
-
-
function onLoaded(evt:Event):void{
-
removeChild(txt);
-
var searchData:XML = new XML(loader.data);
-
var atom:Namespace = searchData.namespace("");
-
-
var leng:int = searchData.atom::entry.length() -1;
-
for (var i:int = leng; i>=0; i--){
-
var cmd:String =
-
searchData.atom::entry[i].atom::title.toString().replace(/\#asgraph/g,
-
"");
-
// added this to ignore words starting with @
-
cmd = cmd.replace(/@(\w+)/g, "");
-
cmds.push(cmd);
-
}
-
-
var time:Timer = new Timer(100, cmds.length);
-
time.addEventListener(TimerEvent.TIMER, onTick);
-
time.start();
-
}
-
function onTick(evt:TimerEvent):void{
-
render(parseFunctions(cmds[evt.target.currentCount - 1]));
-
graphics.endFill();
-
graphics.lineStyle();
-
}
-
-
// parse and run Graphics class commands
-
function parseFunctions(dat:String):Array{
-
var a:Array = dat.split(";") ;
-
for (var i:int = 0; i<a.length-1; i++){
-
a[i] = a[i].split(/\(\)|\(|\)/g);
-
var f:String = a[i][0] = a[i][0].replace(/\s/g,"");
-
a[i] = a[i].splice(0, a[i].length - 1);
-
if (a[i].length> 1){
-
a[i] = a[i][1].split(",");
-
a[i].unshift(f);
-
}
-
}
-
return a.splice(0,a.length - 1);
-
}
-
function render(p:Array):void {
-
for (var i:int = 0; i<p.length; i++) {
-
try{
-
graphics[p[i][0]].apply(graphics,p[i].splice(1));
-
}catch(e:Error){};
-
}
-
}
This is a simple idea I had awhile back... This snippet searches twitter for the #asgraph hashtag and if it finds standard Graphics class method calls it renders them.
So if you tweet something likethis :
#asgraph beginFill(0xFF); drawCircle(200,200,10);
it will get rendered into the below swf (you'll need to refresh to see your tweet get rendered):
This movie requires Flash Player 9
Here is a direct link to the swf
By Zevan | December 4, 2009
Actionscript:
-
...
-
// loop through until we find the root note
-
// grab the third and the fifth and exit the loop
-
for (var i:int = 0; i<leng; i++){
-
if (cMajor[i] == note){
-
third = cMajor[(i + 2) % leng];
-
fifth = cMajor[(i + 4) % leng];
-
break;
-
}
-
}
-
-
// we may need a double sharp on the middle note
-
var sharpFlatDouble:String = sharpFlat;
-
-
// check if this is a sharp, check if it is A or D
-
// if it is add the symbol for double sharp
-
if (sharpFlat == "#"){
-
if (note == "D" || note == "A"){
-
sharpFlatDouble = "x";
-
}
-
}
-
...
If your working on some code... just randomly copy a piece of it and paste it in the comments... This code is from a program that generates any major scale (it's still not finished). Feel free to post code chunks in any language...
[EDIT] the code doesn't need to work on its own... you can just randomly copy from something your working on...
Posted in misc | Tagged actionscript, as3, flash |
By Zevan | December 1, 2009
Actionscript:
-
[SWF(width = 800, height = 600, frameRate = 60)]
-
import com.actionsnippet.qbox.*;
-
stage.frameRate = 60;
-
-
var sim:QuickBox2D = new QuickBox2D(this);
-
-
sim.createStageWalls();
-
-
sim.start();
-
-
var output:TextField = new TextField();
-
output.text = "Click anywhere to add points to a polygon. Hit any key to test.\n\n";
-
output.x = output.y = 50;
-
with(output) width = 300, height = 400, border = true, selectable = true, wordWrap = true, multiline = true;
-
addChild(output);
-
-
function display(str:*):void{
-
output.appendText(str.toString() + "\n");
-
}
-
-
var points:Array = [];
-
var poly:Shape = new Shape();
-
addChild(poly);
-
-
stage.addEventListener(MouseEvent.CLICK, onClick);
-
stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPressed);
-
-
function onClick(evt:MouseEvent):void {
-
if (points.length == 0){
-
poly.graphics.beginFill(0xCCCCCC);
-
poly.graphics.lineStyle(1, 0xFF0000);
-
poly.graphics.moveTo(mouseX, mouseY);
-
}else{
-
poly.graphics.lineTo(mouseX, mouseY);
-
}
-
poly.graphics.drawCircle(mouseX, mouseY, 2);
-
-
points.push(mouseX / 30.0, mouseY / 30.0);
-
}
-
-
function onKeyPressed(evt:KeyboardEvent):void {
-
// average all points
-
var avgX:Number=0
-
var avgY:Number = 0;
-
-
for (var i:int = 0; i<points.length; i+=2){
-
avgX += points[i];
-
avgY += points[i + 1];
-
}
-
-
avgX /= points.length/2;
-
avgY /= points.length/2;
-
avgX = avgX;
-
avgY = avgY;
-
-
// subtract averages and fix decimal place
-
for (i = 0; i<points.length; i+=2){
-
var yp:int = i + 1;
-
points[i] -= avgX;
-
points[yp] -= avgY;
-
points[i] = Number(points[i].toFixed(2));
-
points[yp] = Number(points[yp].toFixed(2));
-
}
-
-
display("points array:");
-
display(points);
-
-
try{
-
var p:QuickObject = sim.addPoly({x:avgX, y:avgY, points:points});
-
p.userData.graphics.beginFill(0xFF0000);
-
p.userData.graphics.drawCircle(0,0,5);
-
}catch(e:*){
-
display("Invalid polygon data!");
-
}
-
-
poly.graphics.clear();
-
points = [];
-
}
This snippet shows the basic concepts needed to go about creating a polygon editor for QuickBox2D. I have an unreleased editor that I use for my QuickBox2D projects, at some point I may release it... but for now I figured I'd post this extremely simplified version for people to expand on.
Have a look at the swf here...
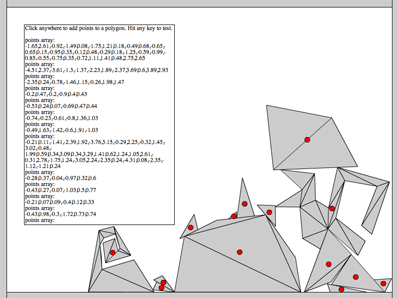