By Zevan | February 17, 2009
Actionscript:
-
var dial:Sprite = Sprite(addChild(new Sprite()));
-
with(dial.graphics) lineStyle(1, 0x000000), beginFill(0xCCCCCC), drawCircle(0,0,100), lineTo(0,0);
-
dial.x = stage.stageWidth / 2;
-
dial.y = stage.stageHeight / 2;
This snippet shows how you can combine drawCircle() and lineTo() to quickly draw a clock/dial primitive shape. I'll use this in tomorrows snippet...
By Zevan | February 16, 2009
Actionscript:
-
[SWF(width=720,height=360,backgroundColor=0x000000,frameRate=30)]
-
-
// ported from here:
-
//http://www.cs.rit.edu/~ncs/color/t_convert.html
-
-
function hsv(h:Number, s:Number, v:Number):Array{
-
var r:Number, g:Number, b:Number;
-
var i:int;
-
var f:Number, p:Number, q:Number, t:Number;
-
-
if (s == 0){
-
r = g = b = v;
-
return [Math.round(r * 255), Math.round(g * 255), Math.round(b * 255)];
-
}
-
-
h /= 60;
-
i = Math.floor(h);
-
f = h - i;
-
p = v * (1 - s);
-
q = v * (1 - s * f);
-
t = v * (1 - s * (1 - f));
-
-
switch( i ) {
-
case 0:
-
r = v;
-
g = t;
-
b = p;
-
break;
-
case 1:
-
r = q;
-
g = v;
-
b = p;
-
break;
-
case 2:
-
r = p;
-
g = v;
-
b = t;
-
break;
-
case 3:
-
r = p;
-
g = q;
-
b = v;
-
break;
-
case 4:
-
r = t;
-
g = p;
-
b = v;
-
break;
-
default: // case 5:
-
r = v;
-
g = p;
-
b = q;
-
break;
-
}
-
return [Math.round(r * 255), Math.round(g * 255), Math.round(b * 255)];
-
}
-
-
-
//
-
// -- test it out by drawing a few things
-
//
-
-
var canvas:BitmapData = new BitmapData(720, 360, false, 0x000000);
-
-
addChild(new Bitmap(canvas));
-
-
canvas.lock();
-
var size:int = canvas.width * canvas.height;
-
var xp:int, yp:int, c:Array, i:int;
-
-
for (i = 0; i<size; i++){
-
xp = i % 360;
-
yp = i / 360;
-
c = hsv(xp, 1, yp / 360);
-
canvas.setPixel(xp, yp, c[0] <<16 | c[1] <<8 | c[2]);
-
}
-
-
var dx:Number, dy:Number, dist:Number, ang:Number;
-
-
for (i = 0; i<size; i++){
-
xp = i % 360;
-
yp = i / 360;
-
dx = xp - 180;
-
dy = yp - 180;
-
dist = 1 - Math.sqrt((dx * dx) + (dy * dy)) / 360;
-
ang = Math.atan2(dy, dx) / Math.PI * 180;
-
if (ang <0){
-
ang += 360;
-
}
-
c = hsv(ang, 1, dist);
-
canvas.setPixel(360 + xp, yp, c[0] <<16 | c[1] <<8 | c[2]);
-
}
-
canvas.unlock();
This is one of those things I've been meaning to play with for awhile. The above demos a function called hsv() which takes 3 arguments: angle (0-360), saturation(0-1) and value(0-1). The function returns an array of rgb values each with a range of (0-255).
There's some room for optimization here, but for clarity I left as is. Even just playing with HSV (also know as HSB) for a few minutes, I see some interesting potential for dynamically generating color palettes for generative style experiments.
I looked around for the most elegant looking code snippet to port in order to write this... I eventually stumbled upon this great resource.
If you test the above on your timeline it will generate this image:
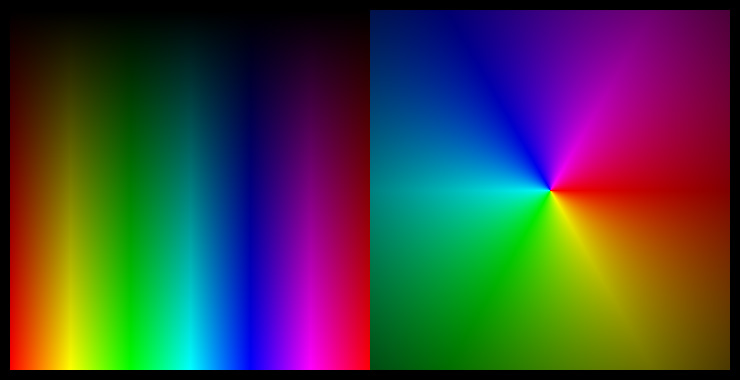
I usually only post one snippet a day... not sure why I decided to post two today.
By Zevan | February 16, 2009
Actionscript:
-
trace("min", Math.min(100, 99, 32, 75, 44, 90));
-
trace("max", Math.max(100, 99, 32, 75, 44, 90));
-
/*outputs:
-
32
-
100
-
*/
It's easy not to notice that Math.min() and Math.max() can take any number of arguments. I've seen people nest min/max calls instead of using the above option.... like this:
Actionscript:
-
trace(Math.min(1, Math.min(2, 3)));
Posted in misc | Tagged actionscript, flash |
By Zevan | February 15, 2009
Actionscript:
-
stage.frameRate=30;
-
var nav:Sprite = Sprite(addChild(new Sprite()));
-
nav.x=nav.y=150;
-
-
var cover:Sprite;
-
var coverDest:Number=0;
-
var spacing:int=4;
-
var btnNum:int=6;
-
-
buildNav();
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function onLoop(evt:Event):void {
-
cover.x += (coverDest - cover.x) /4;
-
}
-
-
function buildNav():void {
-
for (var i:int = 0; i<btnNum; i++) {
-
var b:Sprite=makeBox(50,50);
-
b.x = i * (b.width + spacing);
-
b.buttonMode=true;
-
b.addEventListener(MouseEvent.CLICK, onClick);
-
}
-
cover=makeBox(54, 60);
-
cover.blendMode=BlendMode.INVERT;
-
}
-
-
function onClick(evt:MouseEvent):void {
-
coverDest=evt.currentTarget.x;
-
}
-
-
function makeBox(w:Number, h:Number) {
-
var b:Sprite = new Sprite();
-
with (b.graphics) {
-
beginFill(0x000000),drawRect(-w/2,-h/2,w,h);
-
}
-
nav.addChild(b);
-
return b;
-
}
This is something I do in my intermediate flash classes... the only difference is that we do the graphics in the flash IDE during class time instead of drawing them with ActionScript.
Posted in motion | Tagged actionscript, flash |