By Zevan | March 27, 2010
Actionscript:
-
var offX:Number = 100;
-
var offY:Number = 300;
-
var scalarX:Number = 6;
-
var scalarY:Number = 200;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
var r:Number = mouseY / 100;
-
var xn:Number = (mouseX - 100) / 650;
-
var xn1:Number = 0;
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (var i:int = 0; i<100; i++){
-
xn1 = r * xn * (1 - xn);
-
xn = xn1;
-
if (i == 0){
-
graphics.moveTo(offX+i*scalarX,offY+xn1*scalarY);
-
}else{
-
graphics.lineTo(offX+i*scalarX, offY+xn1*scalarY);
-
}
-
}
-
}
Whenever I can't decide what kind of snippet to make, I simply go to wikipedia or mathworld for a bit and I always end up with something. I've messed with Logistic Maps before (when learning about strange attractors). This is a simple rendering where the x and y axis change the biotic potential (r) and the starting value for x.
Here is the link I used for reference.
Have a look at the swf (just move your mouse around).
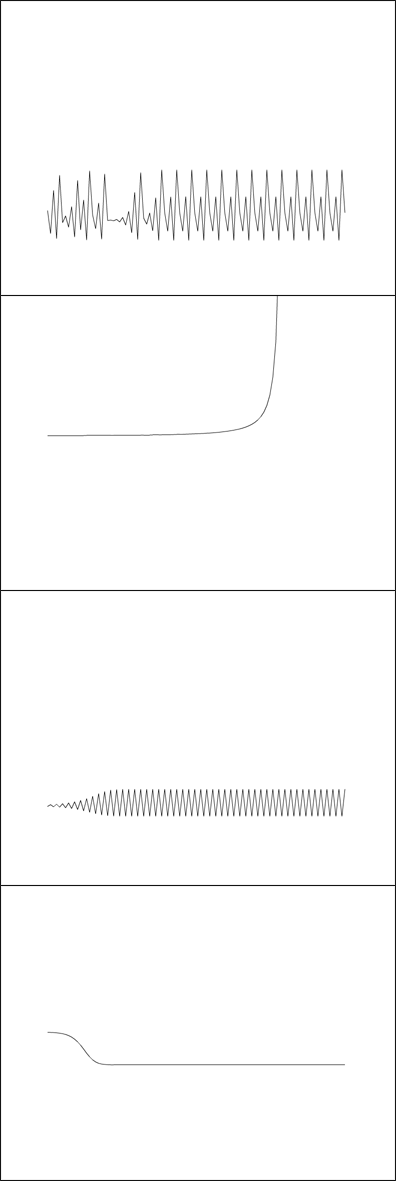
Also posted in Graphics, Math | Tagged actionscript, as3, flash |
By Zevan | March 20, 2010
We're still working on this long term project for medialab prado in madrid. Here is a camera test from today. It uses frame differencing and places names of colors on the areas of motion.
Click here to view the swf file... make sure to move around a bit in front of your web-cam.
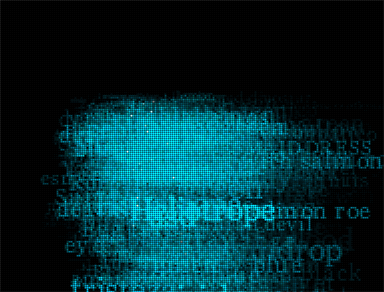
By Zevan | March 19, 2010
Actionscript:
-
[SWF(width = 500, height = 500)]
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = stage.stageWidth / 2;
-
var centerY:Number = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
// data
-
var points:Array = [];
-
var i:int = 0;
-
var pointNum : int = Math.max(2,int(mouseX / 12))
-
-
var radius:Number = 200;
-
var step:Number = TWO_PI / pointNum;
-
var theta:Number = step / 2;
-
for (i = 0; i<pointNum; i++){
-
var xp:Number = centerX + radius * Math.cos(theta);
-
var yp:Number = centerY + radius * Math.sin(theta);
-
points[i] = new Point(xp, yp);
-
theta += step;
-
}
-
// render
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for ( i = 0; i<pointNum; i++){
-
var a:Point = points[i];
-
for (var j:int = 0; j<pointNum; j++){
-
var b:Point = points[j];
-
if (a != b){
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
}
-
}
I've been using this geometric shape for lots of different things recently. Including during consulting gigs as a helpful visualization. Just move your mouse left and right... I particularly like the simpler forms you get by moving your mouse to the left (triangles squares and simple polygons):
While not entirely related this wikipedia article is interesting.
[EDIT : Thanks to martin for reminding me that I can do away with the if statement here in the above code ]
Actionscript:
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (i = 0; i<pointNum; i++) {
-
var a:Point=points[i];
-
for (var j:int = i+1; j<pointNum; j++) {
-
var b:Point=points[j];
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
graphics.drawCircle(a.x, a.y, 10);
I implemented that change over at wonderfl and it works nicely
Also posted in Graphics, Math | Tagged actionscript, as3, flash |
By Zevan | March 15, 2010
Actionscript:
-
[SWF(width = 500, height=500, backgroundColor=0x000000)]
-
-
var clockNum:int = 100;
-
var clocks:Vector.<Function> = new Vector.<Function>(clockNum, true);
-
-
var clockContainer:Sprite = Sprite(addChild(new Sprite()));
-
clockContainer.x = stage.stageWidth / 2;
-
clockContainer.y = stage.stageHeight / 2;
-
buildClocks();
-
runClocks();
-
-
function buildClocks():void{
-
for (var i:int = 0; i<clockNum; i++){
-
var theta:Number = Math.random() * Math.PI * 2;
-
var radius:Number = Math.random() * 200;
-
var xp:Number = radius * Math.cos(theta);
-
var yp:Number = radius * Math.sin(theta);
-
clocks[i] = makeClock(xp,yp,Math.random() * Math.PI * 2);
-
}
-
}
-
function runClocks():void{
-
addEventListener(Event.ENTER_FRAME, onRunClocks);
-
}
-
function onRunClocks(evt:Event):void{
-
for (var i:int = 0; i<clockNum; i++){
-
clocks[i]();
-
}
-
clockContainer.rotationX = clockContainer.mouseY / 30;
-
clockContainer.rotationY = -clockContainer.mouseX / 30;
-
}
-
function makeClock(x:Number, y:Number, time:Number=0):Function{
-
var radius:Number = Math.random() * 20 + 5;
-
var border:Number = radius * 0.2;
-
var smallRadius:Number = radius - radius * 0.3;
-
-
var clock:Sprite = Sprite(clockContainer.addChild(new Sprite()));
-
clock.x = x;
-
clock.y = y;
-
clock.z = 100 - Math.random() * 200;
-
clock.rotationX = Math.random() * 40 - 20;
-
clock.rotationY = Math.random() * 40 - 20;
-
clock.rotationZ = Math.random() * 360;
-
return function():void{
-
with (clock.graphics){
-
clear();
-
lineStyle(1,0xFFFFFF);
-
drawCircle(0,0,radius + border);
-
var xp:Number = smallRadius * Math.cos(time/2);
-
var yp:Number = smallRadius * Math.sin(time/2);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
xp = radius * Math.cos(time);
-
yp = radius * Math.sin(time);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
}
-
time+=0.1;
-
}
-
}
You can go check the swf out at wonderfl.net...
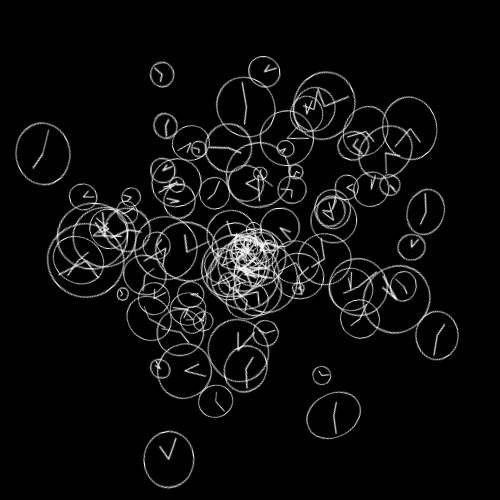