By Zevan | April 13, 2009
Actionscript:
-
function sinh(x:Number):Number{
-
return (Math.pow(Math.E, x) - Math.pow(Math.E, -x)) * 0.5;
-
}
-
-
function cosh(x:Number):Number{
-
return (Math.pow(Math.E, x) + Math.pow(Math.E, -x)) * 0.5;
-
}
Needed sinh and cosh today. Easy enough to create with existing math functions. If you needed more speed you could inline these and replace Math.E with 2.71828183.
Got the math over at wikipedia (as usual).
Also posted in Math | Tagged actionscript, flash |
Actionscript:
-
// Superformula (equations from):
-
// http://www.geniaal.be/downloads/AMJBOT.pdf
-
// http://en.wikipedia.org/wiki/Superformula
-
const TWO_PI:Number = Math.PI * 2;
-
function superShape(a:Number, b:Number, m:Number, n1:Number, n2:Number, n3:Number, pnt:Point, scale:Number):void{
-
var r:Number = 0
-
var p:Number = 0;
-
var xp:Number = 0, yp:Number = 0;
-
while(p <= TWO_PI){
-
var ang:Number = m * p / 4;
-
with(Math){
-
r = pow(pow(abs(cos(ang) / a), n2) + pow(abs(sin(ang) / b), n3),-1/n1);
-
xp = r * cos(p);
-
yp = r * sin(p);
-
}
-
p += .01;
-
canvas.setPixel(pnt.x + xp *scale, pnt.y + yp * scale, 0xFFFFFF);
-
}
-
}
-
// test it out:
-
var canvas:BitmapData = new BitmapData(700,600,false, 0x000000);
-
addChild(new Bitmap(canvas, "auto", true));
-
-
superShape(1, 1, 5, 23, 23, 23, new Point(100,80), 30);
-
superShape(1, 1, 5, 13, 13, 3, new Point(200,80), 30);
-
superShape(1, 1, 8, 3, 13, 3, new Point(300,80), 30);
-
superShape(10,8, 16, 30, 13, 3, new Point(450,80), 30);
-
superShape(1,1, 1, .5, .5, .5, new Point(100,190), 100);
-
-
for (var i:int = 0; i <150; i++){
-
superShape(1,1, 2, 1+i/800, 4, 8-i * .1, new Point(550,350), 50);
-
}
-
for (i = 0; i <20; i++){
-
superShape(1.1,1.2, 6, 2 + i , 4, 9 - i, new Point(200,350), 50);
-
}
The above snippet demos a function that will draw Supershapes using the Superformula...
From wikipedia:
The Superformula appeared in a work by Johan Gielis. It was obtained by generalizing the superellipse, named and popularized by Piet Hein...
Here is the result of the above code:
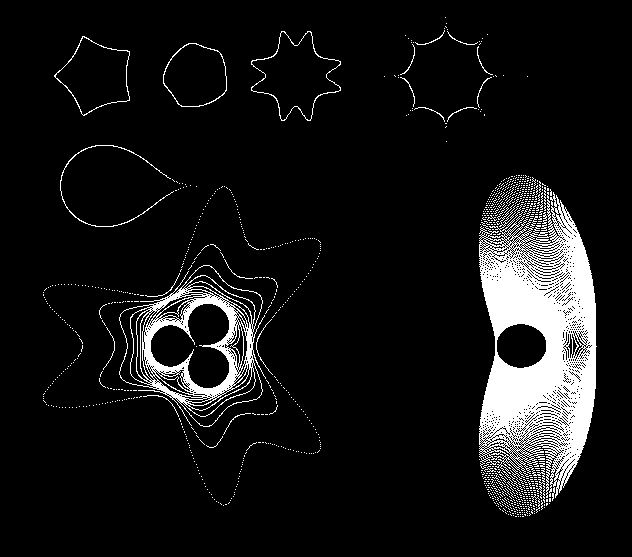
You can read more about the Superformula here in the original paper by Gielis.
wikipedia entry...
3d Supershapes by Paul Bourke
Actionscript:
-
var target:Number = 1024;
-
var slices:Array = [target];
-
var leng:int = 11;
-
-
for (var i:int = 0; i<leng-1; i++){
-
var index:int = int(Math.random()*slices.length);
-
var val:Number = slices[index];
-
var rand:Number = Math.random() * val/2;
-
slices[index] = val - rand;
-
slices.push(rand);
-
}
-
-
trace(slices);
-
-
// test that they all add up
-
var sum:Number = 0;
-
for (i = 0; i<slices.length; i++){
-
sum += slices[i];
-
}
-
trace("test that they all add up: ", sum);
The above snippet creates an array of a specified length whose elements all add up to the variable target. Here is some example output:
165.31133050055192,322.23456030456015,
257.47582363389245,26.9984893942173,1.96283924962002,
5.466277873168191,21.362282634705164,62.68168197512457,
76.63028224500404,36.27274381401516,12.558309228795265,35.04537914634583
test that they all add up: 1024
Also posted in arrays | Tagged actionscript, flash |
Actionscript:
-
var target:Number = 360;
-
var steps:Array = new Array();
-
for (var step:Number = 0; step <target; step += int(Math.random() * 36 + 36)){
-
steps.push(Math.min(target,step));
-
}
-
steps.push(target);
-
trace(steps);
-
/* outputs something similar to:
-
0,46,99,144,189,259,330,360
-
*/
This is something I've had to do a few times recently.... it randomly steps a number toward a given target...
Also posted in arrays | Tagged actionscript, flash |