By Zevan | April 27, 2009
Actionscript:
-
package {
-
-
[SWF(width=1000,height=1000)]
-
import flash.display.*;
-
import flash.events.*;
-
public class RandomWalkTexture extends Sprite {
-
-
private var _canvas:BitmapData;
-
private var _populationNum:int=100;
-
private var _movers:Vector.<Mover>;
-
public function RandomWalkTexture() {
-
var sw:Number=stage.stageWidth;
-
var sh:Number=stage.stageHeight;
-
scaleX=scaleY=.25;
-
_canvas=new BitmapData(sw*4,sh*4,false,0x000000);
-
addChild(new Bitmap(_canvas));
-
-
_movers = new Vector.<Mover>();
-
for (var i:int = 0; i<_populationNum; i++) {
-
_movers[i]=new Mover(_canvas,sw*1.5+Math.random()*sw,sh*1.5+Math.random()*sh);
-
}
-
addEventListener(Event.ENTER_FRAME, onRun);
-
}
-
private function onRun(evt:Event):void {
-
for (var i:int = 0; i<200; i++) {
-
for (var j:int = 0; j<_populationNum; j++) {
-
_movers[j].run();
-
}
-
}
-
}
-
}
-
}
-
-
import flash.display.BitmapData;
-
class Mover {
-
public var x:Number;
-
public var y:Number;
-
public var velX:Number;
-
public var velY:Number;
-
public var speed:Number;
-
private var _canvas:BitmapData;
-
-
public function Mover(canvas:BitmapData, xp:Number, yp:Number) {
-
_canvas=canvas;
-
x=xp;
-
y=yp;
-
velX=0;
-
velY=0;
-
speed=Math.random()*5-2.5;
-
}
-
public function run():void {
-
x+=velX;
-
y+=velY;
-
_canvas.setPixel(x, y, 0xFFFFFF);
-
var dir:Number=int(Math.random()*4);
-
if (dir==0) {
-
velX=0;
-
velY=- speed;
-
} else if (dir == 1) {
-
velX=0;
-
velY=speed;
-
} else if (dir == 2) {
-
velX=- speed;
-
velY=0;
-
} else if (dir == 3) {
-
velX=speed;
-
velY=0;
-
}
-
}
-
}
This snippet is meant to be run as a document class. Nothing special here... this is just something I found laying around - the actual bitmap being drawn is rather big, so I recommending right clicking (control clicking on mac) on the swf and to zoom in and out.
Here are a few images:
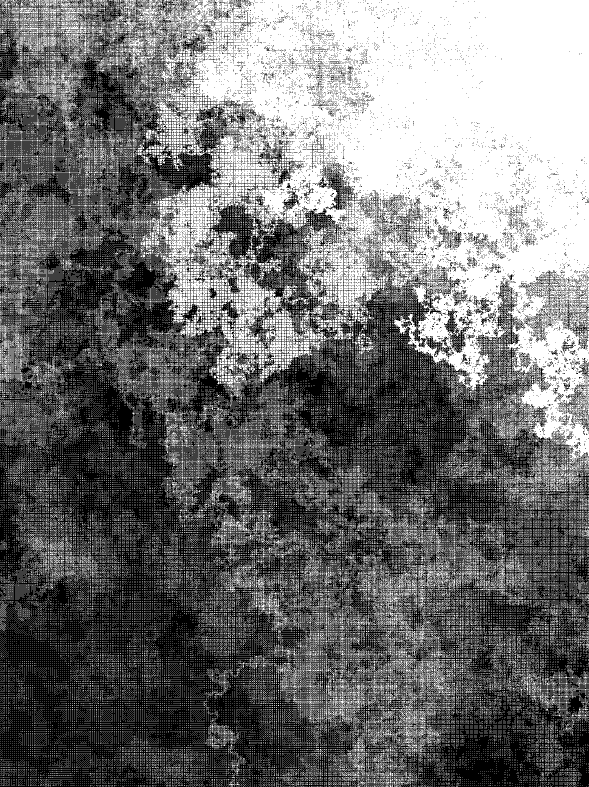
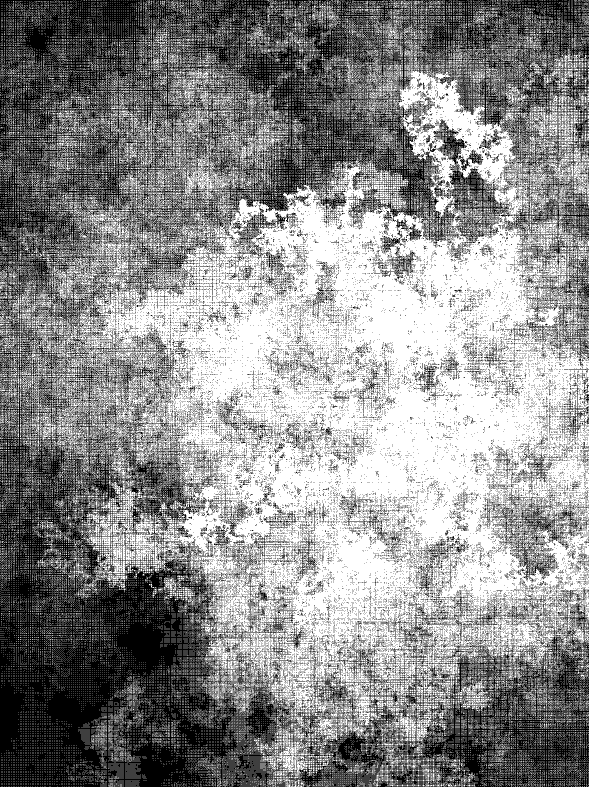
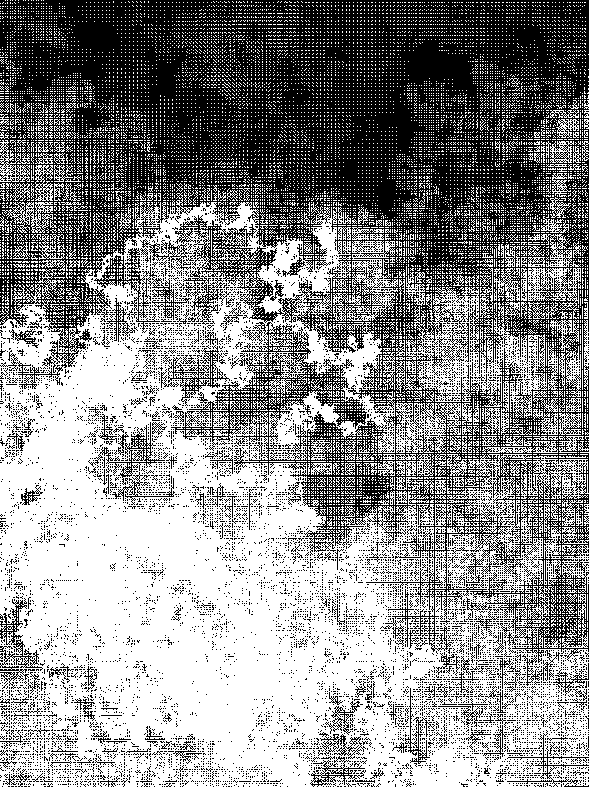
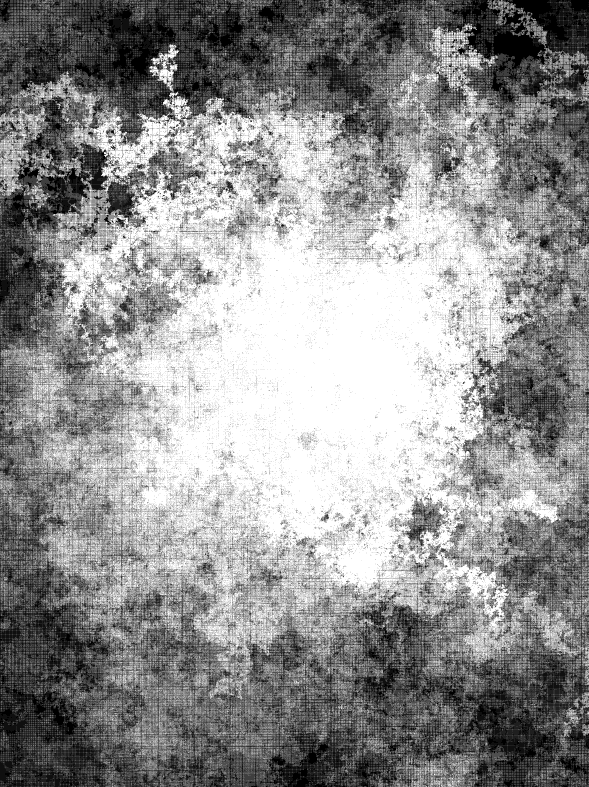
Posted in misc | Tagged actionscript, flash |
By Zevan | April 18, 2009
Actionscript:
-
// build some functions:
-
var redGradient:Function = sl(16, add(100, mult(5)));
-
-
// grid positioning
-
var xPos:Function = add(50, mult(30, cInt(div(4))));
-
var yPos:Function = add(50, mult(30, mod(4)));
-
-
// create some shapes:
-
var shapes:Array = createShapes(this, 22, [["beginFill", 0xCCCCCC], ["drawCircle", 0, 0, 10], ["endFill"], ["lineStyle",1, redGradient], ["drawRect", -5, -5, 10, 10]], {x:yPos, y:xPos, rotation:mult(10)});
-
-
function createShapes(par:DisplayObjectContainer, num:Number,
-
funcs:Array, props:Object):Array {
-
var shapes:Array = [];
-
for (var i:int = 0; i<num; i++){
-
shapes[i] = par.addChild(new Shape());
-
for (var j:int = 0; j<funcs.length; j++) {
-
var a:Array = funcs[j].concat();
-
for (var k:int = 0; k<a.length; k++){
-
if (a[k] is Function){
-
a[k] = a[k](i);
-
}
-
}
-
for (var key:String in props){
-
var v:* = props[key];
-
if (v is Function){
-
shapes[i][key] = v(i);
-
}else{
-
shapes[i][key] = v;
-
}
-
}
-
shapes[i].graphics[a[0]].apply(shapes[i].graphics, a.slice(1));
-
}
-
}
-
return shapes;
-
}
-
-
// function building blocks
-
const F:Function = function(a:*):*{return a};
-
-
function cInt(f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return int(f(n));
-
}
-
}
-
-
function mod(m:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return f(n) % m;
-
}
-
}
-
-
function div(d:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return f(n) / d;
-
}
-
}
-
-
function mult(scalar:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return f(n) * scalar;
-
}
-
}
-
-
function add(off:Number, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return f(n) + off;
-
}
-
}
-
// shift left
-
function sl(amount:int, f:Function=null):Function{
-
if (f == null) f = F;
-
return function(n:Number):Number {
-
return f(n) <<amount
-
}
-
}
This is an unusual snippet I wrote a couple weeks back.... not sure where I was going with this really... has some interesting ideas in it.
Posted in misc | Tagged actionscript, flash |
By Zevan | April 14, 2009
Actionscript:
-
var size:Array = [1, 1.5, .5, 1, .4, 1, 1, 1, .2, 1.1]
-
var boxes:Array = new Array();
-
var spacing:Number = 4;
-
var container:Sprite = Sprite(addChild(new Sprite()));
-
container.x = container.y = 100;
-
-
for (var i:int = 0; i<size.length; i++){
-
var box:Sprite = makeBox();
-
var prev:int = i - 1;
-
box.scaleX= box.scaleY = size[i];
-
if (i == 0){
-
box.y = 10;
-
}else{
-
// here's the trick
-
// if you animate the height property you need to do this again and again:
-
box.y = boxes[prev].y + boxes[prev].height/2+ box.height/2 + spacing
-
}
-
boxes.push(box);
-
}
-
-
function makeBox():Sprite{
-
var box:Sprite = Sprite(container.addChild(new Sprite()));
-
with (box.graphics) beginFill(0xFF0000), drawRect(-50,-10, 100, 20);
-
return box;
-
}
Sometimes you need to position a bunch of Sprites or MovieClips that are different sizes - and you want to keep the spacing between them the same. This snippet shows a simple example of this.
For more info you could also do this tutorial that I wrote on learningactionscript3.com
Also posted in UI | Tagged actionscript, flash |
By Zevan | April 13, 2009
Recently launched a new project over at shapevent.com. If your enjoy sketchbooks, drawings and interactive ugliness... have a look here:
Shapevent Log
Many of the techniques covered in the code on this website will be used in shapevent log entries... no rss feed yet, but I'll have one up for it in the next few days....