Actionscript:
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.autoSize = TextFieldAutoSize.LEFT;
-
txt.x = txt.y = 20;
-
txt.text = "click anywhere to load an image file...";
-
-
var fileRef:FileReference= new FileReference();
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
function onDown(evt:MouseEvent):void{
-
fileRef.browse([new FileFilter("Images", "*.jpg;*.gif;*.png")]);
-
fileRef.addEventListener(Event.SELECT, onSelected);
-
stage.removeEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
}
-
function onSelected(evt:Event):void{
-
fileRef.addEventListener(Event.COMPLETE, onLoaded);
-
fileRef.load();
-
fileRef.removeEventListener(Event.SELECT, onSelected);
-
}
-
function onLoaded(evt:Event):void{
-
var loader:Loader = new Loader();
-
loader.loadBytes(evt.target.data);
-
addChild(loader);
-
fileRef.removeEventListener(Event.COMPLETE, onLoaded);
-
}
This snippet shows how to use the FileReference.load() method to load an image into flash player RAM and display it on the stage.
Actionscript:
-
var canvas:Shape = Shape(addChild(new Shape()));
-
var gestures:Array=[];
-
var gestureNum:int = 0;
-
var capGesture:Array;
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
stage.addEventListener(MouseEvent.MOUSE_UP, onUp);
-
function onDown(evt:MouseEvent):void{
-
capGesture=[];
-
addEventListener(Event.ENTER_FRAME, onCapture);
-
-
canvas.graphics.lineStyle(3, 0xFF0000);
-
canvas.x = mouseX;
-
canvas.y = mouseY;
-
canvas.graphics.moveTo(0, 0);
-
}
-
function onUp(evt:MouseEvent):void{
-
gestures.push(capGesture.concat());
-
gestureNum++;
-
canvas.graphics.clear();
-
removeEventListener(Event.ENTER_FRAME, onCapture);
-
}
-
function onCapture(evt:Event):void{
-
capGesture.push(new Point(canvas.mouseX, canvas.mouseY));
-
canvas.graphics.lineTo(canvas.mouseX, canvas.mouseY);
-
}
-
-
var currGesture:Array;
-
var drawing:Boolean = false;
-
var lineThickness:Number = 0;
-
var lineColor:Number = 0x000000;
-
var index:int = 0;
-
var pnt:Point;
-
var trans:Matrix = new Matrix();
-
var i:int
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
if (gestureNum> 0){
-
if (!drawing){
-
currGesture = gestures[int(Math.random() * gestureNum)].concat();
-
trans.identity();
-
trans.rotate(Math.random()*6.28);
-
var scale:Number = Math.random() * 2 + .1;
-
trans.scale(scale, scale);
-
trans.tx = Math.random() * stage.stageWidth
-
trans.ty = Math.random() * stage.stageHeight
-
for (i = 0; i<currGesture.length; i++){
-
currGesture[i] = trans.transformPoint(currGesture[i]);
-
}
-
lineThickness = Math.random() * Math.random() * 50;
-
if (int(Math.random()*10) ==1){
-
var col:uint = uint(Math.random()*255);
-
lineColor = col <<16 | col <<8 | col;
-
}
-
index = 0;
-
drawing = true;
-
graphics.lineStyle(lineThickness, lineColor);
-
}else{
-
for (i = 0; i<10; i++){
-
if (drawing == true){
-
pnt = currGesture[index];
-
if (index == 0){
-
graphics.moveTo(pnt.x, pnt.y);
-
}else{
-
graphics.lineTo(pnt.x, pnt.y);
-
}
-
index++;
-
if (index == currGesture.length){
-
drawing = false;
-
}
-
}
-
}
-
}
-
}
-
}
This snippet is an idea I have been meaning to try for sometime. It's a mini-drawing program. You can draw single gestures (shapes, letters etc...) and the program then randomly scales, rotates, tints and translates these gestures repeatedly on the canvas. You can continue to draw as it does this, the more gestures you draw, the more the program will have to randomly choose from.
Have a look at the swf here...
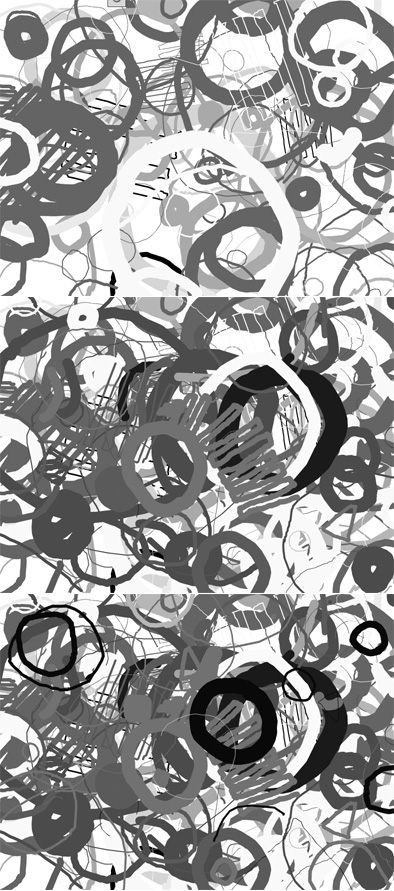
Actionscript:
-
[SWF(width=800, height=600)]
-
var xn1:Number;
-
var xn:Number = Math.random() * Math.random() * .2;
-
var inc:int = 0;
-
var xp:Number = 10;
-
var yp:Number = 10;
-
var count:int = 1;
-
scaleX = scaleY = 2;
-
graphics.lineStyle(0,0x00000);
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
xn1 = 2 * xn % 1;
-
xn = xn1;
-
if (inc == 0){
-
graphics.moveTo(xp + inc, yp + 30 - xn1 * 30);
-
}else{
-
graphics.lineTo(xp + inc, yp + 30 - xn1 * 30);
-
}
-
inc++
-
if (inc == 50){
-
inc = 0;
-
xp = 10 + count % 6 * 60;
-
yp = 10 + int(count / 6) * 60;
-
xn = Math.random() * Math.random() * .2;
-
trace(xn);
-
count++;
-
}
-
}
This snippet plots 2x mod 1 maps with random starting values for xn. More info over at wikipedia and mathworld.
Also posted in Math, motion | Tagged actionscript, as3, flash |
XML:
-
<code>
-
<make reference="w" class="BasicView" args="stage.stageWidth, stage.stageHeight, false"/>
-
<call method="addChild" args="w"/>
-
-
<make reference="wireMat" class="WireframeMaterial" args="0x000000" />
-
-
<make reference="sphere" class="Sphere" args="wireMat, 100" />
-
-
<call method="w.scene.addChild" args="sphere" />
-
-
<make reference="animation" class="Object">
-
<set z="-500" rotationY="360" rotationX="360" ease="Back.easeOut"/>
-
</make>
-
-
<call method="TweenLite.to" args="sphere, 3, animation" />
-
-
<call method="setInterval" args="w.singleRender, 32" />
-
-
</code>
This snippet shows XML that the mini-library AsXML can read and run - in this case AsXML is set up to run with Papervision
A few days ago I had the idea to write some code that would run ActionScript based on XML. I spent some time getting rid of a few bugs and setting up some demos with TweenLite, Papervision and QuickBox2D. I wrapped everything up into a mini-library called AsXML.
Check out the demos here.
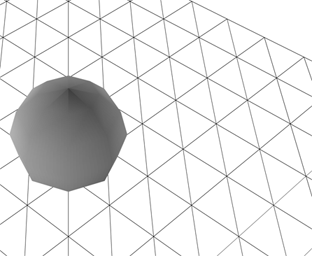
Download AsXML and demo files here.
AsXML Features:
1) call methods of the main timeline
2) read and write properties on the main timeline
3) instantiate classes on the main timeline
4) call methods on these classes
5) read and write properties on these classes
6) store references to return values from functions
Also posted in Box2D, Graphics, Math, QuickBox2D, XML, dynamic, external data, instantiation, motion, return values, string manipulation, strings | Tagged actionscript, as3, flash |