Actionscript:
-
makeFlyer();
-
-
function makeFlyer():void{
-
var thing:MovieClip = new MovieClip();
-
thing.x = 200;
-
thing.y = 200;
-
-
addChild(thing);
-
-
var prop:Shape = new Shape();
-
with (prop.graphics){
-
lineStyle(0,0x000000);
-
beginFill(0x000000);
-
moveTo(-100,0);
-
curveTo(-100, -30, 0, 0);
-
curveTo(100, 30, 100, 0);
-
curveTo(100, -30, 0, 0);
-
curveTo(-100, 30, -100, 0);
-
}
-
prop.scaleX = prop.scaleY = 0.5;
-
var container:MovieClip = new MovieClip();
-
//container.x = -50;
-
container.addChild(prop);
-
container.scaleY = 0.6;
-
thing.addChild(container);
-
-
var body:Shape = new Shape();
-
with (body.graphics){
-
lineStyle(0, 0x000000);
-
beginFill(0x000000);
-
lineTo(0,80);
-
drawCircle(0,80,10);
-
}
-
thing.addChild(body);
-
thing.velX = 0;
-
thing.velY = 0;
-
thing.posX = thing.x;
-
thing.posY = thing.y;
-
thing.theta = 0;
-
thing.prop = prop;
-
thing.addEventListener(Event.ENTER_FRAME, onRun);
-
}
-
function onRun(evt:Event):void{
-
var t:MovieClip = MovieClip(evt.currentTarget);
-
t.prop.rotation += 10
-
t.velY = 3 * Math.cos(t.theta);
-
t.velX = 3 * Math.sin(t.theta / 2);
-
t.theta += 0.05
-
t.posX += t.velX;
-
t.posY += t.velY;
-
-
t.x = t.posX;
-
t.y = t.posY;
-
}
This snippet creates a small flying object that moves with sine and cosine.
Have a look at the swf...
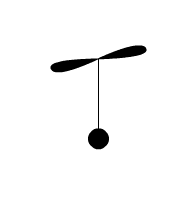
Posted in motion | Tagged actionscript, as3, flash |
By Zevan | April 24, 2010
Actionscript:
-
var circles:Array = [];
-
for (var i:int = 0; i<30; i++){
-
var c:Sprite = makeCircle();
-
c.x = stage.stageWidth / 2;
-
c.y = stage.stageHeight / 2;
-
c.scaleX = 1 + i/2;
-
c.scaleY = 0.5 + i/4;
-
addChild(c);
-
circles.push(c);
-
}
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
circles[0].y += (mouseY - circles[0].y) / 4;
-
for (var i:int = 1; i<circles.length; i++){
-
var pre:Sprite = circles[i - 1];
-
circles[i].y += (pre.y - circles[i].y) / 4;
-
}
-
}
-
function makeCircle():Sprite{
-
var s:Sprite = new Sprite();
-
with(s.graphics){
-
lineStyle(0,0x000000);
-
drawCircle(0,0,10);
-
}
-
return s;
-
}
This morning I woke up with a vision of this simple mouse toy in my head. I decided I might as well code it up... I may do more simple things like this in the next few days, it's relaxing.
Also posted in Graphics, misc | Tagged actionsnippet, as3, flash |
By Zevan | March 15, 2010
Actionscript:
-
[SWF(width = 500, height=500, backgroundColor=0x000000)]
-
-
var clockNum:int = 100;
-
var clocks:Vector.<Function> = new Vector.<Function>(clockNum, true);
-
-
var clockContainer:Sprite = Sprite(addChild(new Sprite()));
-
clockContainer.x = stage.stageWidth / 2;
-
clockContainer.y = stage.stageHeight / 2;
-
buildClocks();
-
runClocks();
-
-
function buildClocks():void{
-
for (var i:int = 0; i<clockNum; i++){
-
var theta:Number = Math.random() * Math.PI * 2;
-
var radius:Number = Math.random() * 200;
-
var xp:Number = radius * Math.cos(theta);
-
var yp:Number = radius * Math.sin(theta);
-
clocks[i] = makeClock(xp,yp,Math.random() * Math.PI * 2);
-
}
-
}
-
function runClocks():void{
-
addEventListener(Event.ENTER_FRAME, onRunClocks);
-
}
-
function onRunClocks(evt:Event):void{
-
for (var i:int = 0; i<clockNum; i++){
-
clocks[i]();
-
}
-
clockContainer.rotationX = clockContainer.mouseY / 30;
-
clockContainer.rotationY = -clockContainer.mouseX / 30;
-
}
-
function makeClock(x:Number, y:Number, time:Number=0):Function{
-
var radius:Number = Math.random() * 20 + 5;
-
var border:Number = radius * 0.2;
-
var smallRadius:Number = radius - radius * 0.3;
-
-
var clock:Sprite = Sprite(clockContainer.addChild(new Sprite()));
-
clock.x = x;
-
clock.y = y;
-
clock.z = 100 - Math.random() * 200;
-
clock.rotationX = Math.random() * 40 - 20;
-
clock.rotationY = Math.random() * 40 - 20;
-
clock.rotationZ = Math.random() * 360;
-
return function():void{
-
with (clock.graphics){
-
clear();
-
lineStyle(1,0xFFFFFF);
-
drawCircle(0,0,radius + border);
-
var xp:Number = smallRadius * Math.cos(time/2);
-
var yp:Number = smallRadius * Math.sin(time/2);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
xp = radius * Math.cos(time);
-
yp = radius * Math.sin(time);
-
moveTo(0,0);
-
lineTo(xp, yp);
-
}
-
time+=0.1;
-
}
-
}
You can go check the swf out at wonderfl.net...
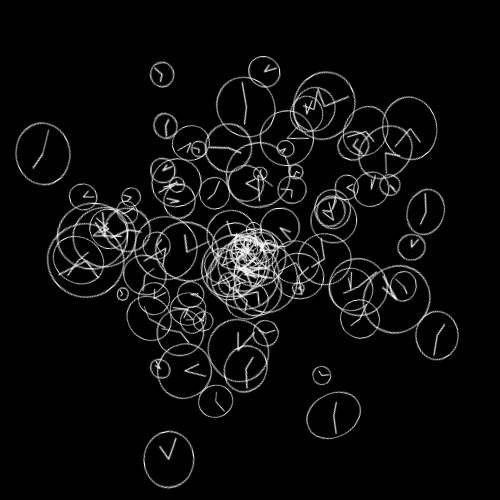
Also posted in 3D, Graphics, misc | Tagged actionscript, as3, flash |
By Zevan | December 30, 2009
Actionscript:
-
var boxA:Shape = Shape(addChild(new Shape()));
-
with (boxA.graphics) beginFill(0), drawRect(-10,-10,20,20);
-
-
var boxB:Shape = Shape(addChild(new Shape()));
-
with (boxB.graphics) beginFill(0), drawRect(-10,-10,20,20);
-
-
boxA.x = 100;
-
boxA.y = 100;
-
-
boxB.x = 200;
-
boxB.y = 100;
-
-
var rot:Number = 32750;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
rot += 1
-
// will stop rotating
-
boxA.rotation = rot
-
// will keep rotating
-
boxB.rotation = rot % 360;
-
}
I recently became aware of a strange aspect of the rotation property on DisplayObjects. For some reason, once it's value goes a little beyond ~32750 the DisplayObject will simply stop rotating. If you read the rotation property it is still changing, but there is no visual update - a quick check on the DisplayObject.transform.matrix property will show that the value has stopped.
The easy fix is to use mod before applying the value to the rotation property. Surprised I've never come across this one before. Maybe someone can shed some light on this.
// for people searching google for solutions to this problem I'll add the following key words:
MovieClip stops rotating, DisplayObject stops rotating, rotation property broken, not rotating