Usually if you want to draw lines in HTML you use canvas or SVG. Awhile back I wondered how I might do it without those. This is really just a proof of concept speed coded answer to that question:
This works by using a div with a border, rotating it and scaling it as needed so it fits between two arbitrary points.
This could be abstracted a bit more, but it works pretty well. I usually choose `setInterval` over `requestAnimationFrame` when prototyping - because I like to easily be able to change the speed of
framebased things like this. If I were to try and make this code more dynamic, I would probably switch out to `requestAnimationFrame`.
If you try and connect two lines together - you’ll notice some inaccuracy - a good argument for using SVG or canvas over something like this. That said, if you are connecting two elements using a single line, this inaccuracy would become irrelevant.
Around 2015 I had the idea for a PRNG that would clamp itself and have moments of “smoothness”. When I got around to trying to create such a thing, the result was something I jokingly called the “Hermit Crab Curve”. I also called it the “Shard Curve”.
The equation for the curve defines a radius in polar coordinates:
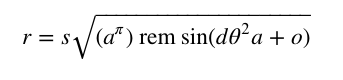
Where a and d are paramters that control the detail of the curve. o is a rotation and offset value for the angle and s is a scalar. Note the use of rem. The resulting curve is much less interesting if a standard modulo is used in place of a remainder:
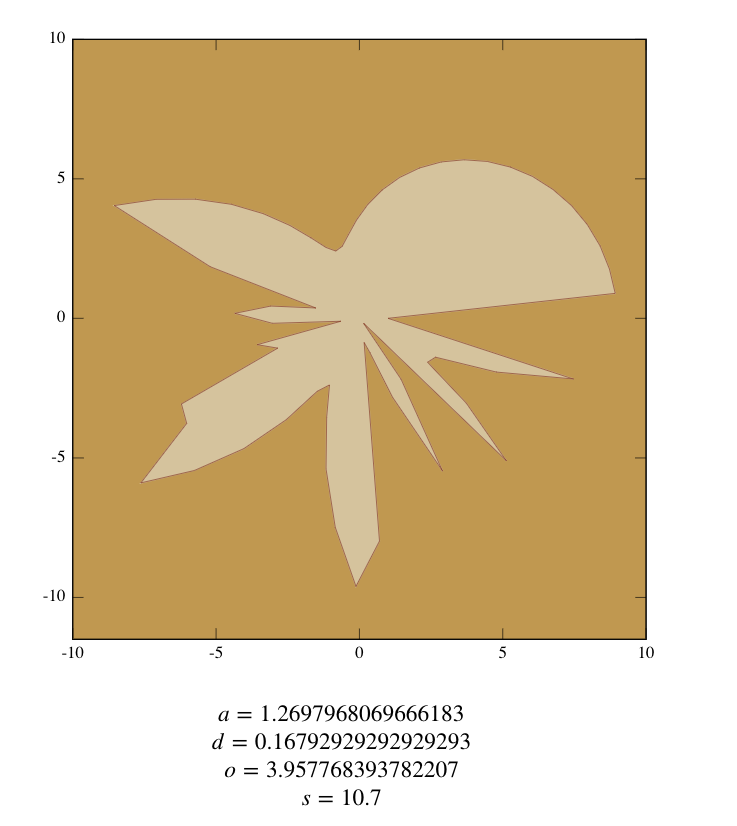
The above variable values were found using this interactive version of the equation:
To illustrate how you might use this as a PRNG I created this fork of the above pen:
That, in combination with the information from my other article from yesterday… Should be enough to see what I mean.
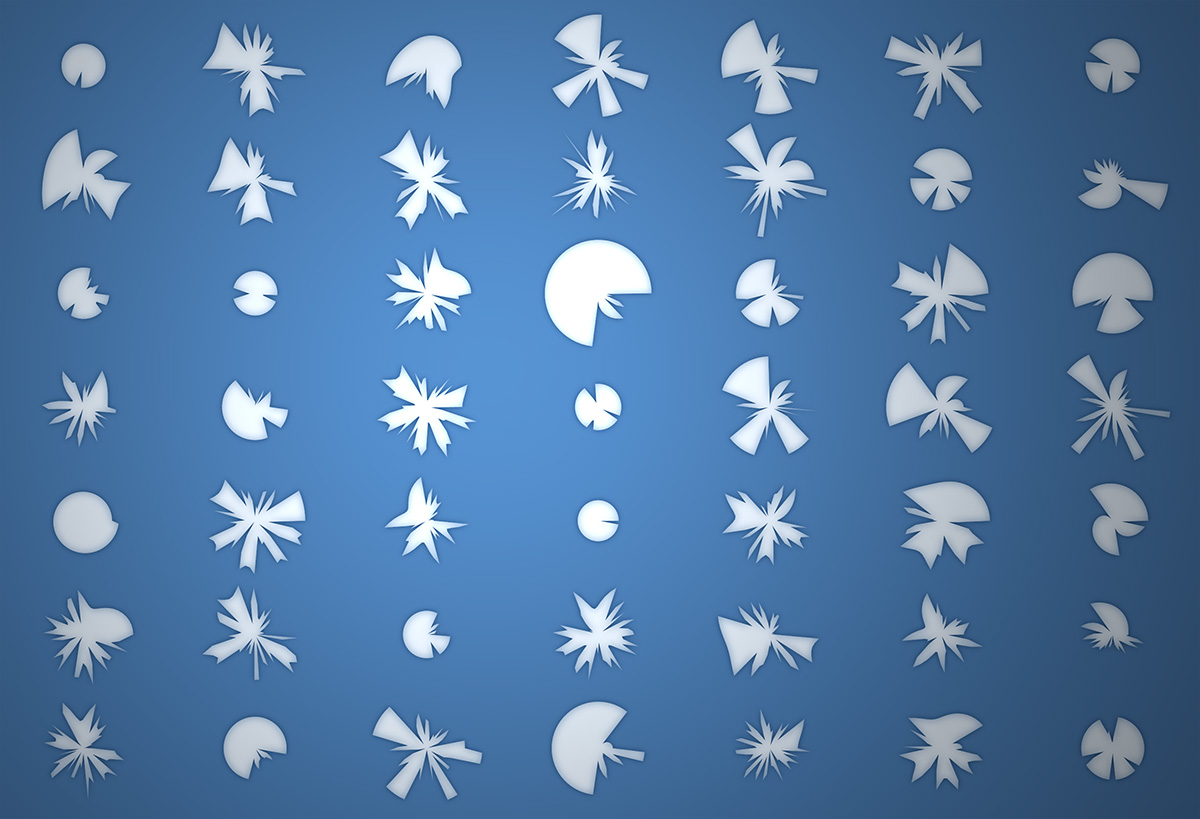
You can read the original description of the Hermit Crab Curve that I created using ArcType here:
http://zevanrosser.com/arctype-dev/hermit-crab-curve.html
If you end up using this for something interesting let me know. I’d love to see it 
Circle Fitting
Actionscript:
-
var circs:Array = []
-
var circNum:int = 600;
-
addEventListener(Event.ENTER_FRAME, onAdd);
-
function onAdd(evt:Event):void {
-
if (circs.length <circNum){
-
makeGrowable();
-
}
-
}
-
-
function makeGrowable(){
-
-
var s:MovieClip = MovieClip(addChild(new MovieClip()));
-
s.x = Math.random() * stage.stageWidth;
-
s.y = Math.random() * stage.stageHeight;
-
with(s.graphics){
-
lineStyle(0,0);
-
drawCircle(0,0,10);
-
}
-
s.scaleX = s.scaleY = 0;
-
circs.push(s);
-
s.addEventListener(Event.ENTER_FRAME, onScaleUp);
-
}
-
-
function onScaleUp(evt:Event):void {
-
var c:MovieClip = MovieClip(evt.currentTarget);
-
c.scaleX = c.scaleY += 0.05;
-
for (var i:int = 0; i<circs.length; i++){
-
var circ:MovieClip = circs[i];
-
if (circ != c){
-
var amt:Number = circ.width/2 + c.width/2;
-
var dx:Number = circ.x - c.x;
-
var dy:Number = circ.y - c.y;
-
var dist:Number = Math.sqrt(dx * dx + dy * dy);
-
if (amt> dist){
-
c.removeEventListener(Event.ENTER_FRAME, onScaleUp);
-
if (c.scaleX <0.1){
-
if (contains(c)){
-
removeChild(c);
-
}
-
}
-
}
-
}
-
-
}
-
}
Circle fitting is one of those things I've never bothered to do... today I figured I'd give it a try and this is what I came up with. I posted it on wonderfl:
Also posted in Graphics, misc | Tagged actionscript, as3, flash, s |
3D Ring
Actionscript:
-
[SWF(width = 500, height=500)]
-
var ring:MovieClip = createRing();
-
ring.x = stage.stageWidth / 2;
-
ring.y = stage.stageHeight / 2;
-
addChild(ring);
-
-
function createRing(sectionNum:int = 30):MovieClip{
-
var container:MovieClip = new MovieClip();
-
container.circles = [];
-
container.theta = 0;
-
container.thetaDest = 0;
-
var step:Number = (Math.PI * 2) / sectionNum;
-
for (var i:int = 0; i<sectionNum; i++){
-
var c:MovieClip = new MovieClip();
-
with (c.graphics){
-
lineStyle(0,0x000000);
-
beginFill(0xCCCCCC);
-
drawCircle(0,0,20);
-
}
-
c.thetaOffset = step * i;
-
container.addChild(c);
-
container.circles.push(c);
-
}
-
container.addEventListener(Event.ENTER_FRAME, onRun);
-
return container;
-
}
-
function onRun(evt:Event):void {
-
var container:MovieClip = MovieClip(evt.currentTarget);
-
var num:int = container.circles.length;
-
for (var i:int = 0; i<num; i++){
-
var c:MovieClip = container.circles[i];
-
var angle:Number = container.theta + c.thetaOffset;
-
c.x = 200 * Math.cos(angle);
-
c.y = 100 * Math.sin(angle);
-
c.scaleX = (100 + c.y) / 120 + 0.2;
-
c.scaleY = c.scaleX;
-
}
-
container.circles.sortOn("y", Array.NUMERIC);
-
for (i = 0; i<num; i++){
-
container.addChild(container.circles[i]);
-
}
-
if (container.mouseX <-100){
-
container.thetaDest -= 0.05;
-
}
-
if (container.mouseX> 100){
-
container.thetaDest += 0.05;
-
}
-
container.theta += (container.thetaDest - container.theta) / 12;
-
-
}
This snippet shows how to create a 3D ring navigation using sine and cosine. Have a look: