By Zevan | April 24, 2010
Actionscript:
-
var circles:Array = [];
-
for (var i:int = 0; i<30; i++){
-
var c:Sprite = makeCircle();
-
c.x = stage.stageWidth / 2;
-
c.y = stage.stageHeight / 2;
-
c.scaleX = 1 + i/2;
-
c.scaleY = 0.5 + i/4;
-
addChild(c);
-
circles.push(c);
-
}
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
circles[0].y += (mouseY - circles[0].y) / 4;
-
for (var i:int = 1; i<circles.length; i++){
-
var pre:Sprite = circles[i - 1];
-
circles[i].y += (pre.y - circles[i].y) / 4;
-
}
-
}
-
function makeCircle():Sprite{
-
var s:Sprite = new Sprite();
-
with(s.graphics){
-
lineStyle(0,0x000000);
-
drawCircle(0,0,10);
-
}
-
return s;
-
}
This morning I woke up with a vision of this simple mouse toy in my head. I decided I might as well code it up... I may do more simple things like this in the next few days, it's relaxing.
Also posted in misc, motion | Tagged actionsnippet, as3, flash |
Actionscript:
-
x = y = 10
-
graphics.lineStyle(1,0);
-
drawBox(6);
-
-
function drawBox(iter:Number=10, count:Number=1, y:Number=0, w:Number=500):void{
-
if (count <iter){
-
var width:Number = w / count
-
for (var i:int = 0; i<count; i++){
-
graphics.drawRect(i * width, width * count/w, width, width);
-
}
-
count++;
-
drawBox(iter, count, y, width);
-
}
-
}
This small snippet just draws this image:
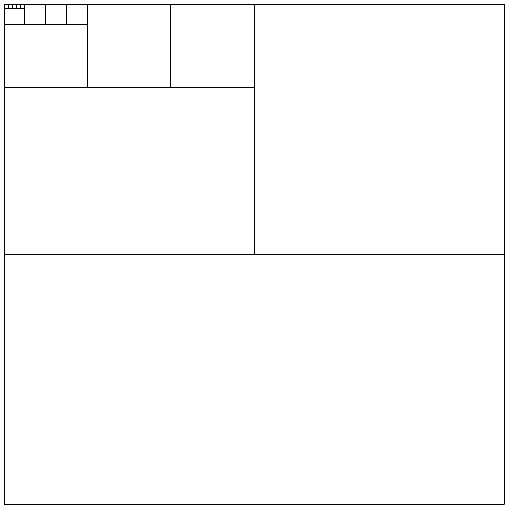
If you have an idea for a short recursive snippet. Feel free to post it in the comments.
Also posted in functions | Tagged actionscript, as3, flash |
By Zevan | March 27, 2010
Actionscript:
-
var offX:Number = 100;
-
var offY:Number = 300;
-
var scalarX:Number = 6;
-
var scalarY:Number = 200;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
var r:Number = mouseY / 100;
-
var xn:Number = (mouseX - 100) / 650;
-
var xn1:Number = 0;
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (var i:int = 0; i<100; i++){
-
xn1 = r * xn * (1 - xn);
-
xn = xn1;
-
if (i == 0){
-
graphics.moveTo(offX+i*scalarX,offY+xn1*scalarY);
-
}else{
-
graphics.lineTo(offX+i*scalarX, offY+xn1*scalarY);
-
}
-
}
-
}
Whenever I can't decide what kind of snippet to make, I simply go to wikipedia or mathworld for a bit and I always end up with something. I've messed with Logistic Maps before (when learning about strange attractors). This is a simple rendering where the x and y axis change the biotic potential (r) and the starting value for x.
Here is the link I used for reference.
Have a look at the swf (just move your mouse around).
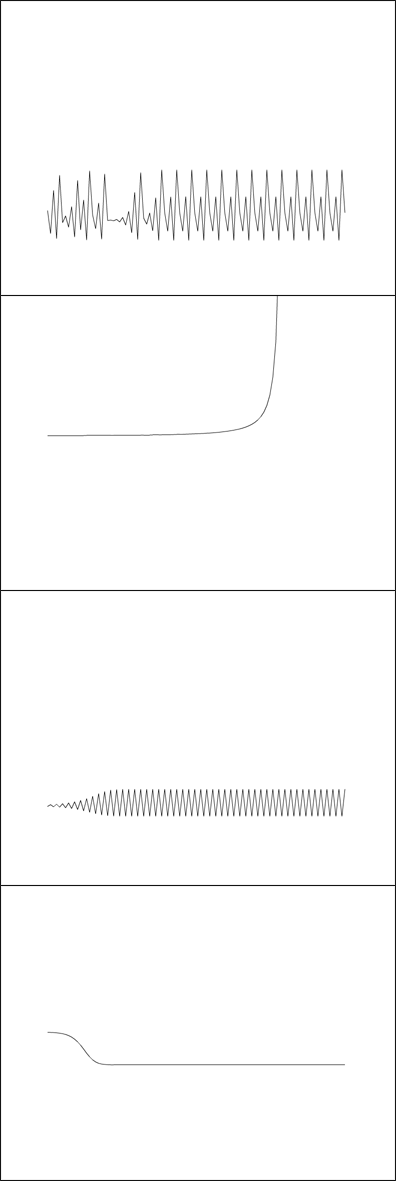
Also posted in Math, misc | Tagged actionscript, as3, flash |
By Zevan | March 19, 2010
Actionscript:
-
[SWF(width = 500, height = 500)]
-
const TWO_PI:Number = Math.PI * 2;
-
var centerX:Number = stage.stageWidth / 2;
-
var centerY:Number = stage.stageHeight / 2;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void{
-
// data
-
var points:Array = [];
-
var i:int = 0;
-
var pointNum : int = Math.max(2,int(mouseX / 12))
-
-
var radius:Number = 200;
-
var step:Number = TWO_PI / pointNum;
-
var theta:Number = step / 2;
-
for (i = 0; i<pointNum; i++){
-
var xp:Number = centerX + radius * Math.cos(theta);
-
var yp:Number = centerY + radius * Math.sin(theta);
-
points[i] = new Point(xp, yp);
-
theta += step;
-
}
-
// render
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for ( i = 0; i<pointNum; i++){
-
var a:Point = points[i];
-
for (var j:int = 0; j<pointNum; j++){
-
var b:Point = points[j];
-
if (a != b){
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
}
-
}
I've been using this geometric shape for lots of different things recently. Including during consulting gigs as a helpful visualization. Just move your mouse left and right... I particularly like the simpler forms you get by moving your mouse to the left (triangles squares and simple polygons):
While not entirely related this wikipedia article is interesting.
[EDIT : Thanks to martin for reminding me that I can do away with the if statement here in the above code ]
Actionscript:
-
graphics.clear();
-
graphics.lineStyle(0,0);
-
for (i = 0; i<pointNum; i++) {
-
var a:Point=points[i];
-
for (var j:int = i+1; j<pointNum; j++) {
-
var b:Point=points[j];
-
graphics.drawCircle(a.x, a.y, 10);
-
graphics.moveTo(a.x, a.y);
-
graphics.lineTo(b.x, b.y);
-
}
-
}
-
graphics.drawCircle(a.x, a.y, 10);
I implemented that change over at wonderfl and it works nicely
Also posted in Math, misc | Tagged actionscript, as3, flash |