By Zevan | March 12, 2009
Actionscript:
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(400, 400, false, 0x000000)))).bitmapData;
-
-
function line(x1:Number, y1:Number, x2:Number, y2:Number, res:int=10):void{
-
var dx:Number = x2 - x1;
-
var dy:Number = y2 - y1;
-
var dist:Number = Math.sqrt((dx * dx) + (dy * dy));
-
var step:Number = 1 / (dist / res);
-
for (var i:Number = 0; i<=1; i+= step){
-
// lerp : a + (b - a) * f
-
canvas.setPixel(x1 + dx * i, y1 + dy * i, 0xFFFFFF);
-
}
-
}
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
canvas.fillRect(canvas.rect, 0x000000);
-
line(100 , 100, mouseX, mouseY,1);
-
line(100 + 50, 100, mouseX+ 50, mouseY,5);
-
}
Yesterday I posted an implementation of the Bresenham Line Algorithm. Today I'm posting a comparatively slow way to draw a line with setPixel(). This snippet uses lerp and the Pythagorean theorem. It works nicely for small numbers of lines, its easy to draw dotted lines with it and its easy to explain. In a real app where you needed to use setPixel() to draw a line you should use one of the fast algorithms like Wu or Bresenham.
I didn't originally write this snippet to use set pixel... a few weeks ago I wrote something very similar to calculate a set of x y coords between two given points. In the program I used it in speed wasn't an issue (as I only needed to run the function one time). I've needed this kind of function many times before in games and small apps...
This was the original:
Actionscript:
-
function calculatePoints(x1:Number, y1:Number, x2:Number, y2:Number, res:int=10):Array{
-
var points:Array = new Array();
-
var dx:Number = x2 - x1;
-
var dy:Number = y2 - y1;
-
var dist:Number = Math.sqrt((dx * dx) + (dy * dy));
-
var step:Number = 1 / (dist / res);
-
for (var i:Number = 0; i<=1; i+= step){
-
points.push(new Point(x1 + dx * i, y1 + dy * i));
-
}
-
return points;
-
}
-
-
trace(calculatePoints(0,0,100,0,10));
-
/* outputs:
-
(x=0, y=0),(x=10, y=0),(x=20, y=0),(x=30.000000000000004, y=0),(x=40, y=0),(x=50, y=0),(x=60, y=0),(x=70, y=0),(x=80, y=0),(x=89.99999999999999, y=0),(x=99.99999999999999, y=0)
-
*/
...and another version to allow you to specify the number of points to calculate rather than the pixel interval at which they should be calculated:
Actionscript:
-
function calculatePoints(x1:Number, y1:Number, x2:Number, y2:Number, pointNum:int=10):Array{
-
var points:Array = new Array();
-
var step:Number = 1 / (pointNum + 1);
-
for (var i:Number = 0; i<=1; i+= step){
-
points.push(new Point(x1 + (x2 - x1) * i, y1 + (y2 - y1) * i));
-
}
-
return points;
-
}
-
-
trace(calculatePoints(0,30,30,0,1));
-
/* outputs:
-
(x=0, y=30),(x=15, y=15),(x=30, y=0)
-
*/
This last version isn't perfect, sometimes the pointNum will be off by 1, I may fix that in a future post.
By Zevan | March 10, 2009
Actionscript:
-
var harmonic:Number = 0;
-
var k:Number = 1;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
harmonic += 1 / k;
-
trace("+");
-
trace("1 / " + k + " = " + harmonic);
-
k+=1;
-
}
Read more about the harmonic series at wikipedia.
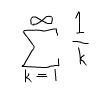
1 / 1 = 1
+
1 / 2 = 1.5
+
1 / 3 = 1.8333333333333333
+
1 / 4 = 2.083333333333333
+
...
Posted in Math | Tagged actionscript, flash, Math |
Actionscript:
-
[SWF(width = 600, height = 600)]
-
var a:Number = 0.02;
-
var b:Number = .9998;
-
-
var xn1:Number = 5;
-
var yn1:Number = 0;
-
var xn:Number, yn:Number;
-
-
var scale:Number = 10;
-
var iterations:Number = 20000;
-
-
function f(x:Number):Number{
-
var x2:Number = x * x;
-
return a * x + (2 * (1 - a) * x2) / (1 + x2);
-
}
-
-
var canvas:BitmapData = Bitmap(addChild(new Bitmap(new BitmapData(600,600,false,0xEFEFEF)))).bitmapData;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
-
canvas.fillRect(canvas.rect, 0xEFEFEF);
-
a = mouseY / 1000;
-
xn1 = mouseX / 30;
-
yn1 = 0;
-
for (var i:int = 0; i<iterations; i++){
-
xn = xn1;
-
yn = yn1;
-
-
xn1 = b * yn + f(xn);
-
yn1 = -xn + f(xn1);
-
canvas.setPixel( 280 + xn1 * scale, 300 + yn1 * scale, 0x000000);
-
}
-
}
Notice the setup for this is very similar to yesterdays flames attractor post.
Back in october of last year I stumbled upon the excellent subblue website by Tom Beddard. I was REALLY impressed by a blog post about Gumowski / Mira patterns. If you haven't seen it you should go take a look.
I'd never heard of Gumowski / Mira patterns before and made a mental note to go and try to read about them and maybe find an equation to port to actionscript or processing. Anyway, a few days ago I decided to go ahead and look up the math and... this is the result.
I got equation over at mathworld...
For simplicity I intentionally made the actionscript code look as much like the mathworld equation as possible. Using f for my function name and using xn1, yn1 etc... There are a few speed optimizations that could be made but I wanted this snippet to be very readable.
Here are a few examples of what this code will generate:
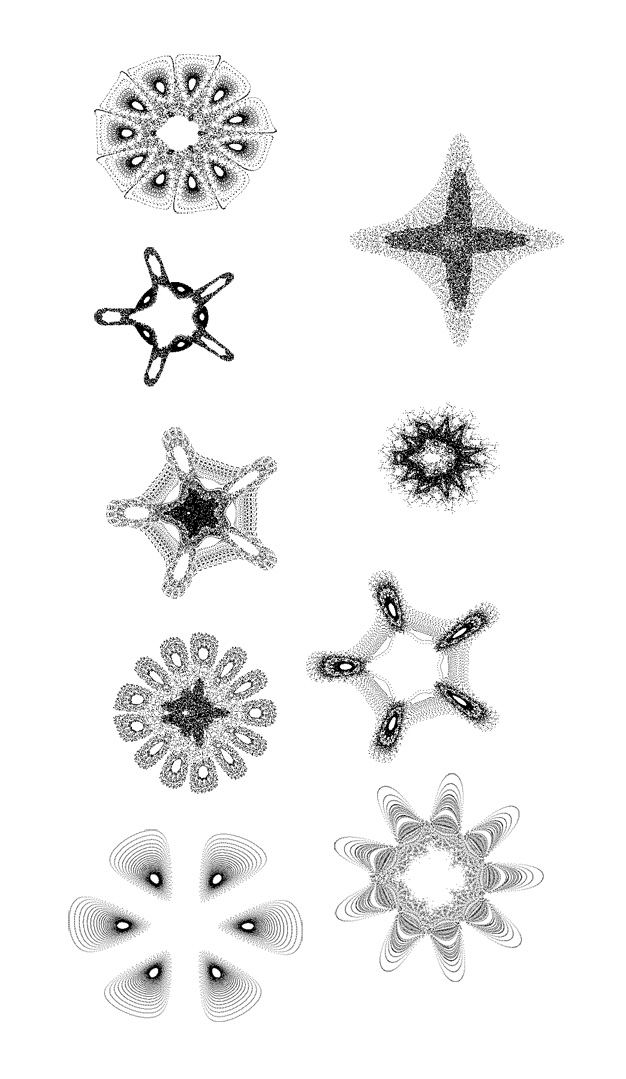
Also posted in setPixel | Tagged actionscript, flash |
By Zevan | February 25, 2009
Actionscript:
-
var txt:TextField = TextField(addChild(new TextField()));
-
txt.text = "";
-
txt.width = 190;
-
txt.height = 400;
-
txt.multiline = true;
-
-
var count:int = 1;
-
function render():void{
-
var line = int(count).toString(2);
-
while(line.length <31){
-
line = "0" + line;
-
}
-
txt.appendText(line + "\n");
-
txt.scrollV= txt.maxScrollV;
-
}
-
-
addEventListener(Event.ENTER_FRAME, onCountUp);
-
function onCountUp(evt:Event):void {
-
count *= 2;
-
render();
-
if (count ==0x40000000){
-
removeEventListener(Event.ENTER_FRAME, onCountUp);
-
addEventListener(Event.ENTER_FRAME, onCountDown);
-
}
-
}
-
function onCountDown(evt:Event):void {
-
count /= 2;
-
render();
-
if (count ==1){
-
addEventListener(Event.ENTER_FRAME, onCountUp);
-
removeEventListener(Event.ENTER_FRAME, onCountDown);
-
}
-
}
The above animates a zig zag pattern in a TextField.