By Zevan | April 28, 2009
Actionscript:
-
var canvas:BitmapData=new BitmapData(400,400,false,0x000000);
-
addChild(new Bitmap(canvas));
-
-
var a:Number=-1.21;
-
var r:Rectangle=new Rectangle(0,0,3,5);
-
var halfWidth:Number=canvas.width/2;
-
var halfHeight:Number=canvas.height/2;
-
-
render();
-
-
function render():void{
-
for (var x:Number = -2; x<=2; x+=.01) {
-
for (var y:Number = -2; y<=2; y+=.02) {
-
-
// equation from : http://en.wikipedia.org/wiki/Bicuspid_curve
-
//(x^2 - a^2) * (x - a)^2 + (y^2 - a^2) * (y^2 - a^2) = 0
-
-
// unpoptimized:
-
// var e:Number = (x*x - a*a) * (x-a)*(x-a) + (y*y-a*a) * (y*y-a*a);
-
// optimized:
-
var x_a:Number=x-a;
-
// factoring: x^2 - a^2 = (x + a) * (x - a)
-
var y2_a2:Number = (y + a) * (y - a);
-
var e:Number = (x + a) * x_a * x_a * x_a + y2_a2 * y2_a2;
-
-
r.x=halfWidth+y*50;
-
r.y=halfHeight-x*100;
-
var col:Number = e * 50;
-
if (col <10){
-
col = Math.abs(col) + 70;
-
canvas.fillRect(r, col <<16 | col <<8 | col );
-
}
-
}
-
}
-
}
This is a variation on a post from a little while back.... it plots a modified Bicuspid that resembles a tooth:
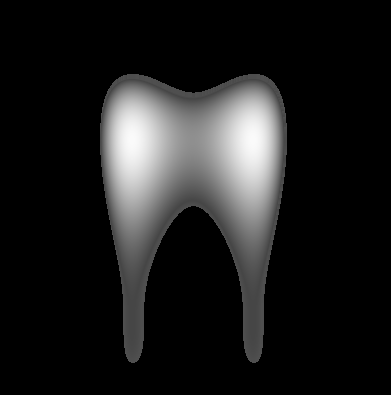
By Zevan | April 26, 2009
Actionscript:
-
var canvas:BitmapData=new BitmapData(200,200,false,0x000000);
-
addChild(new Bitmap(canvas));
-
scaleX=scaleY=2;
-
var pixNum:int=canvas.width*canvas.height;
-
-
var xp:Vector.<int> = new Vector.<int>();
-
var yp:Vector.<int> = new Vector.<int>();
-
var radius:Vector.<Number> = new Vector.<Number>();
-
var theta:Vector.<Number> = new Vector.<Number>();
-
for (var i:int = 0; i<pixNum; i++) {
-
xp.push(i % 200);
-
yp.push(int(i / 200));
-
var dx:Number=100-xp[i];
-
var dy:Number=100-yp[i];
-
theta.push(Math.atan2(dy, dx));
-
radius.push(Math.sqrt(dx * dx + dy * dy)/20);
-
}
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
canvas.lock();
-
var n:Number = mouseX / 100;
-
for (var i:int = 0; i<pixNum; i++) {
-
var swirl:Number = 1+Math.sin(6*Math.cos(radius[i]) -n*theta[i]);
-
canvas.setPixel(xp[i], yp[i], Math.abs(255 - swirl * 255));
-
}
-
canvas.unlock();
-
}
This snippet creates a swirl gradient. While this snippet is not highly optimized, it does implement a basic optimization technique ... I cache some repetitive calculations in Vectors and then use them on the main loop.
I got the function for a swirl gradient from mathworld...
By Zevan | April 21, 2009
Actionscript:
-
var matrix:Matrix3D = new Matrix3D();
-
var verts:Vector.<Number> = new Vector.<Number>();
-
var pVerts:Vector.<Number> = new Vector.<Number>();
-
var uvts:Vector.<Number> = new Vector.<Number>();
-
const TWO_PI:Number=Math.PI * 2;
-
var step:Number=.05;
-
-
var brush:BitmapData = new BitmapData(3, 2, true, 0x41FFFFFF);
-
var n:Number=8;
-
var xp:Number=0,yp:Number=0,a:Number=12,t:Number=0;
-
for (var i:Number = 0; i<TWO_PI; i+=step) {
-
for (var j:Number = 0; j<TWO_PI; j+=step) {
-
// unoptimized for readability
-
var cosi:Number = a/n * ((n - 1) * Math.cos(i) + Math.cos(Math.abs((n - 1) * i)));
-
var sini:Number = a/n * ((n - 1) * Math.sin(i) - Math.sin(Math.abs((n - 1) * i)));
-
var cosj:Number = a/n * ((n - 1) * Math.cos(j) + Math.cos(Math.abs((n - 1) * j)));
-
var sinj:Number = a/n * ((n - 1) * Math.sin(j) - Math.sin(Math.abs((n - 1) * j)));
-
verts.push(cosi * cosj);
-
verts.push(sini * cosj);
-
verts.push(a * sinj);
-
pVerts.push(0),pVerts.push(0);
-
uvts.push(0),uvts.push(0),uvts.push(0);
-
}
-
}
-
var canvas:BitmapData=new BitmapData(400,400,false,0x000000);
-
addChild(new Bitmap(canvas));
-
var dx:Number=0;
-
var dy:Number=0;
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
dx += (mouseX - dx)/4;
-
dy += (mouseY - dy)/4;
-
matrix.identity();
-
matrix.appendRotation(dy,Vector3D.X_AXIS);
-
matrix.appendRotation(dx,Vector3D.Y_AXIS);
-
matrix.appendTranslation(200, 200, 0);
-
Utils3D.projectVectors(matrix, verts, pVerts, uvts);
-
canvas.lock();
-
canvas.fillRect(canvas.rect, 0x000000);
-
var p = new Point();
-
for (var i:int = 0; i<pVerts.length; i+=2) {
-
p.x = pVerts[i];
-
p.y = pVerts[i + 1];
-
canvas.copyPixels(brush, brush.rect, p, null, null, true);
-
}
-
canvas.unlock();
-
}
Taking the Hypocycloid stuff from yesterday into 3D...
Also posted in 3D, BitmapData | Tagged actionscript, flash |
By Zevan | April 19, 2009
Actionscript:
-
x = stage.stageWidth / 2;
-
y = stage.stageHeight / 2;
-
// change n to alter number of spikes (cuspes)
-
var n:Number = 8;
-
var xp:Number = 0, yp:Number = 0, a:Number = 10, t:Number = 0;
-
-
graphics.lineStyle(0, 0x000000);
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
for (var i:int = 0; i<10; i++){
-
// unoptimized for simplicity and similarity to original equations from here:
-
// http://mathworld.wolfram.com/Hypocycloid.html
-
xp = a/n * ((n - 1) * Math.cos(t) + Math.cos(Math.abs((n - 1) * t)));
-
yp = a/n * ((n - 1) * Math.sin(t) - Math.sin(Math.abs((n - 1) * t))) ;
-
-
a *= 1.002;
-
if (t == 0){
-
graphics.moveTo(xp, yp);
-
}else{
-
graphics.lineTo(xp, yp);
-
}
-
t += .1;
-
}
-
}
This code draws a Hypocycloid with a radius that increments - this results in a spiral formation.
I got the math from mathworld... here.
Posted in Math | Tagged actionscript, flash |