By Zevan | September 14, 2009
Actionscript:
-
import com.actionsnippet.qbox.*;
-
import Box2D.Common.Math.*;
-
-
[SWF(width = 800, height = 600, backgroundColor = 0x000000, frameRate=60)]
-
-
var sim:QuickBox2D = new QuickBox2D(this, {debug:false, frim:true});
-
-
sim.createStageWalls();
-
-
var boxA:QuickObject = sim.addBox({x:3, y:3, width:2, height:2, fillColor:0xFF0000});
-
var boxB:QuickObject = sim.addBox({x:3, y:6, width:2, height:2, fillColor:0xFF0000});
-
-
sim.start();
-
sim.mouseDrag();
-
-
// when boxA touches boxB a circle QuickObject is created
-
var contacts:QuickContacts = sim.addContactListener();
-
// listen for contact points being added
-
contacts.addEventListener(QuickContacts.ADD, onAdd);
-
function onAdd(evt:Event):void{
-
// see if this contact event is associated with boxA and boxB
-
if(contacts.isCurrentContact(boxA, boxB)){
-
// get the location of the collision in world space
-
var loc:b2Vec2 = contacts.currentPoint.position;
-
// you cannot create new QuickObjects inside this listener function
-
// so we just give a 5 ms delay
-
setTimeout(sim.addCircle, 5, {x:loc.x, y:loc.y});
-
}
-
}
Note: This snippet requires QuickBox2D 1.0 or greater
I've created a few simple examples to show how to use QuickBox2D contact listeners. This is the first one. When two boxes collide circles are added to the simulation at the point of collision.
Have a look at the swf...
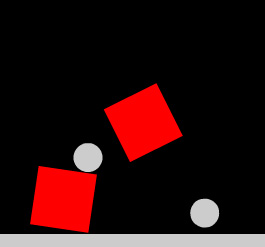
By Zevan | September 14, 2009
QuickBox2D 1.0 is ready. It contains a bunch of small bug fixes and a few new features that I'll be demoing in the next few posts. The main new features relate to collision detection and simple event sequencing.
Go download it from here...
I also spent some time updating the docs.... everything is documented... I may add additional documentation text over the next few days...
A detailed tutorial for QuickBox2D is also in the works...
[EDIT]
Also, if you find any bugs send me an e-mail (see about page for e-mail)... if there are any bugs left I'd like to fix the asap.... You can also feel free to e-mail any API suggestions...
By Zevan | September 13, 2009
Actionscript:
-
private function addCube(xp:Number, yp:Number, zp:Number, w:Number, h:Number, leng:Number):void{
-
var hw:Number = w * 0.5;
-
var hh:Number = h * 0.5;
-
var hl:Number = leng * 0.5;
-
var xA:Number = xp - hw;
-
var xB:Number = hw + xp;
-
var yA:Number = yp - hh;
-
var yB:Number = hh + yp;
-
var zA:Number = zp - hl;
-
var zB:Number = hl + zp;
-
_verts.push(xA, yA, zA,
-
xB, yA, zA,
-
xA, yB, zA,
-
xB, yB, zA,
-
xA, yA, zB,
-
xB, yA, zB,
-
xA, yB, zB,
-
xB, yB, zB);
-
-
var index:int = _boxIndex * 8;
-
var i0:int = index, i1:int = index + 1, i2:int = index + 2;
-
var i3:int = index + 3, i4:int = index + 4, i5:int = index + 5;
-
var i6:int = index + 6, i7:int = index + 7;
-
_indices.push(i0, i1, i2,
-
i1, i3, i2,
-
i6, i7, i4,
-
i7, i5, i4,
-
i1, i5, i3,
-
i7, i3, i5,
-
i4, i5, i0,
-
i1, i0, i5,
-
i2, i6, i0,
-
i0, i6, i4,
-
i2, i3, i6,
-
i3, i7, i6);
-
-
_faces.push(new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face());
-
_uvts.push(Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0);
-
_boxIndex++;
-
}
Lately I've been posting large code snippets... so today I'm highlighting part of a larger snippet - The above code is the heart of a small experiment I created this morning. It sets up a cube for use with drawTraingles().
The rest of the code can be read here:
Cubes3D.as
Have a look at the swf here...
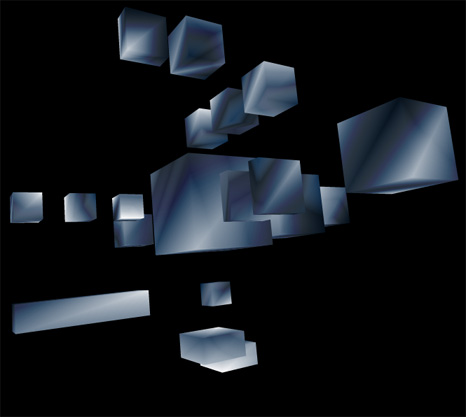
I also put it on wonderfl...
By Zevan | September 12, 2009
Actionscript:
-
var rad:Number = 1;
-
-
var surfaceArea:Number = sphereSurfaceArea(rad);
-
var volume:Number = sphereVolume(rad)
-
trace("surface area: ", surfaceArea);
-
trace("volume:", volume);
-
trace("volume / surface area:", volume / surfaceArea);
-
-
function sphereSurfaceArea(r:Number):Number{
-
return 4 * Math.PI * r * r;
-
}
-
-
function sphereVolume(r:Number):Number{
-
return r * r * r * (4.0/3.0) * Math.PI;
-
}
-
-
/*outputs:
-
surface area: 12.566370614359172
-
volume: 4.1887902047863905
-
volume / surface area: 0.3333333333333333
-
*/
Last night I was feeling curious about sphere volume and sphere surface area... I used WolframAlpha to find the neccessary equations and then wrote the above code snippet...
Check out how WolframAlpha does it:
surface area of a sphere
surface area of a sphere with radius 10
sphere volume
sphere volume with radius 10