By Zevan | November 11, 2024
Still speed-coding shorts for youtube these last few months… - move your mouse over the codepen below - click and hold to zoom out
https://www.youtube.com/@zevanrosser/shorts
Also posted in 3D, Graphics, Math, color, glsl, graphics algorithms, html5, javascript, misc, motion, webgl |
By Zevan | January 3, 2010
Actionscript:
-
[SWF(width = 500, height = 500, frameRate = 30)]
-
-
var canvas:BitmapData = new BitmapData(stage.stageWidth,stage.stageHeight,false, 0xFFFFFF);
-
-
var indexCanvas:BitmapData = new BitmapData(stage.stage.stageWidth, stage.stageHeight, false,
-
0xFFFFFF);
-
addChild(new Bitmap(canvas));
-
-
var s:Shape = new Shape();
-
-
var lineData:Array = [];
-
var dataIndex:int = 0;
-
-
trace(0xFFFFFF - 1)
-
var totalLines:int = 20000;
-
var iterations:int = 9;
-
var linesPerIter:int = totalLines / iterations;
-
-
var xp:int = stage.stageWidth / 2;
-
var yp:int = stage.stageHeight / 2;
-
-
var stepAmt:Number = 60;
-
var halfStepAmt:Number = stepAmt / 2;
-
-
addEventListener(Event.ENTER_FRAME, onDraw);
-
function onDraw(evt:Event):void {
-
if (lineData.length <totalLines){
-
generateData(linesPerIter);
-
}else{
-
stage.quality = "high";
-
addChild(s);
-
s.x = 0;
-
s.y = 0;
-
-
removeEventListener(Event.ENTER_FRAME, onDraw);
-
addEventListener(Event.ENTER_FRAME, onRun);
-
}
-
}
-
-
function onRun(evt:Event):void {
-
var currentIndex:int = indexCanvas.getPixel(mouseX, mouseY);
-
var currentLine:Array = lineData[currentIndex];
-
-
s.graphics.clear();
-
if (currentIndex != 0xFFFFFF){
-
s.graphics.lineStyle(3, 0xFF0000);
-
s.graphics.moveTo(currentLine[0], currentLine[1]);
-
s.graphics.lineTo(currentLine[2], currentLine[3]);
-
}
-
}
-
-
function generateData(num:int):void{
-
var rxA:int, rxB:int, ryA:int, ryB:int;
-
var g:Graphics = s.graphics;
-
for (var i:int = 0; i<num; i++){
-
rxA = xp;
-
ryA = yp;
-
-
xp += Math.round(Math.random() * stepAmt) - halfStepAmt;
-
yp += Math.round(Math.random() * stepAmt) - halfStepAmt;
-
-
if (xp> stage.stageWidth){
-
xp = stage.stageWidth - halfStepAmt;
-
}else
-
if (xp <0){
-
xp = halfStepAmt;
-
}
-
if (yp> stage.stageHeight){
-
yp = stage.stageHeight - halfStepAmt;
-
}else
-
if (yp <0){
-
yp = halfStepAmt;
-
}
-
-
rxB = xp;
-
ryB = yp;
-
-
lineData[dataIndex] = [rxA, ryA, rxB, ryB];
-
s.x = rxA;
-
s.y = ryA;
-
var endX:Number = rxB - rxA;
-
var endY:Number = ryB - ryA;
-
var m:Matrix = s.transform.matrix;
-
g.clear();
-
g.lineStyle(1, 0x000000, 0.3);
-
-
g.lineTo(endX, endY);
-
stage.quality = "high";
-
canvas.draw(s, m);
-
-
g.clear();
-
g.lineStyle(3, dataIndex);
-
-
g.lineTo(endX, endY);
-
stage.quality = "low";
-
indexCanvas.draw(s, m);
-
-
dataIndex++
-
}
-
}
I'm working on a data visualization that contains a long path made up of approximately one million points. There is some information associated with every two sets of coordinates that needs to be displayed when the user rolls their mouse over any part of the line.
I took a little time to think about the best way to do this and came up with a few techniques. The first one I tried seems to work nicely - this snippet is the proof of concept for that first technique. I tested this snippet with 1,000,000 xy coordinates and it works nicely. It takes a little while to draw though, so for the purposes of this demo I've just included 20,000 coordinates.
Have a look at the swf over at wonderfl.net
The way this works is by drawing lines to two different BitmapData instances. I draw anti-aliased slightly transparent lines to a BitmapData instance called "canvas" (this is added to the display list) - I then draw aliased lines to a BitmapData called "indexCanvas" (this is never added to the display list) - each aliased line uses an incremental value for its color - this incremental value is also the index for a two dimensional array containing the coordinate information for the aliased line. I use getPixel() on the "indexCanvas" and use the return value as the index for the 2D array. The data from the 2D array is used to draw a red line with the graphics class. This technique enables you to have many many rollovers and all you ever have to do is call getPixel() and use the returned color value to look up info about what you're mouse is touching.
There are a few cool ways this could be repurposed and this is really only one solution to the problem of having many many things that you need to be able to rollover... there are others that don't use BitmapData at all... I may write those up in the next couple of days.
Also posted in BitmapData, Data Structures, UI, arrays, display list, graphics algorithms, misc, pixel manipulation, return values | Tagged actionscript, as3, flash |
By Zevan | September 13, 2009
Actionscript:
-
private function addCube(xp:Number, yp:Number, zp:Number, w:Number, h:Number, leng:Number):void{
-
var hw:Number = w * 0.5;
-
var hh:Number = h * 0.5;
-
var hl:Number = leng * 0.5;
-
var xA:Number = xp - hw;
-
var xB:Number = hw + xp;
-
var yA:Number = yp - hh;
-
var yB:Number = hh + yp;
-
var zA:Number = zp - hl;
-
var zB:Number = hl + zp;
-
_verts.push(xA, yA, zA,
-
xB, yA, zA,
-
xA, yB, zA,
-
xB, yB, zA,
-
xA, yA, zB,
-
xB, yA, zB,
-
xA, yB, zB,
-
xB, yB, zB);
-
-
var index:int = _boxIndex * 8;
-
var i0:int = index, i1:int = index + 1, i2:int = index + 2;
-
var i3:int = index + 3, i4:int = index + 4, i5:int = index + 5;
-
var i6:int = index + 6, i7:int = index + 7;
-
_indices.push(i0, i1, i2,
-
i1, i3, i2,
-
i6, i7, i4,
-
i7, i5, i4,
-
i1, i5, i3,
-
i7, i3, i5,
-
i4, i5, i0,
-
i1, i0, i5,
-
i2, i6, i0,
-
i0, i6, i4,
-
i2, i3, i6,
-
i3, i7, i6);
-
-
_faces.push(new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face(),
-
new Face(), new Face(), new Face());
-
_uvts.push(Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0,
-
Math.random(), Math.random(), 0);
-
_boxIndex++;
-
}
Lately I've been posting large code snippets... so today I'm highlighting part of a larger snippet - The above code is the heart of a small experiment I created this morning. It sets up a cube for use with drawTraingles().
The rest of the code can be read here:
Cubes3D.as
Have a look at the swf here...
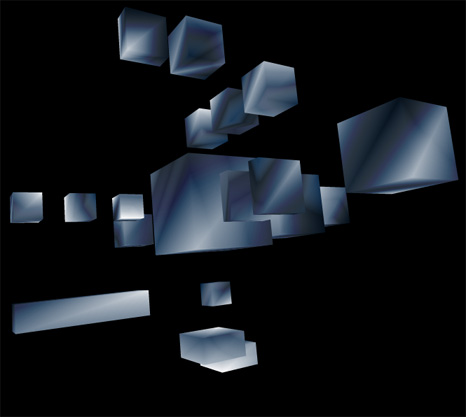
I also put it on wonderfl...
By Zevan | September 8, 2009
Actionscript:
-
[SWF(width=600, height=600, frameRate=30)]
-
var sw:Number = stage.stageWidth;
-
var sh:Number = stage.stageHeight;
-
-
var s:Shape = Shape(addChild(new Shape()));
-
-
var scale:Number = 1;
-
var scaleDest:Number = 1;
-
var down:Boolean = false;
-
var dx:Number = 0, dy:Number = 0, time:Number = 0;
-
-
buttonMode = true;
-
-
addInstructions();
-
vectorTexture();
-
-
stage.addEventListener(MouseEvent.MOUSE_DOWN, onDown);
-
stage.addEventListener(MouseEvent.MOUSE_UP, onUp);
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
-
function addInstructions():void{
-
var instruct:Sprite = Sprite(addChild(new Sprite()));
-
with (instruct.graphics) beginFill(0x666666), drawRect(0,0,270, 30);
-
instruct.x = instruct.y = 20;
-
var txt:TextField = TextField(instruct.addChild(new TextField()));
-
txt.defaultTextFormat = new TextFormat("Verdana", 11);
-
txt.x = txt.y = 5;
-
txt.selectable = false;
-
with (txt) textColor = 0xFFFFFF, autoSize = "left", text = "Click and hold to zoom, move mouse to pan";
-
}
-
-
function vectorTexture():void{
-
var cols:Vector.<uint> = Vector.<uint>([0xFFFFFF, 0x000000]);
-
var rnd:Vector.<Number> = new Vector.<Number>(6, true);
-
-
for(var i:int = 0 ; i<50; i++){
-
with(s.graphics){
-
lineStyle(Math.random() * 50 + 2, cols[int(Math.random()*cols.length)]);
-
drawCircle(Math.random() * sw, Math.random() * sh, 10 + Math.random() * Math.random() * 400);
-
}
-
}
-
s.graphics.lineStyle(20, 0xCCCCCC);
-
s.graphics.drawRect(0, 0,sw, sh);
-
}
-
-
function onDown(evt:MouseEvent):void{ down = true; }
-
function onUp(evt:MouseEvent):void{ down = false; }
-
function onLoop(evt:Event):void {
-
if (down){
-
scaleDest *= 1.05;
-
time = 0;
-
}else{
-
time++;
-
// zoom out after 30 iterations
-
if (time == 30){
-
scaleDest = 1;
-
}
-
}
-
scale += (scaleDest - scale) / 4;
-
if (scale> 10) scale = scaleDest = 10;
-
-
dx += (mouseX - dx) / 4;
-
dy += (mouseY - dy) / 4;
-
if (dx <0) dx = 0;
-
if (dy <0) dy = 0;
-
if (dx> sw) dx = sw;
-
if (dy> sh) dy = sh;
-
-
// matrix zoom/pan
-
var m:Matrix = s.transform.matrix;
-
m.identity();
-
m.translate(-dx,-dy);
-
m.scale(scale, scale);
-
m.translate(dx,dy);
-
s.transform.matrix = m;
-
}
I haven't been by the computer much these last two weeks - been traveling. Going back to nyc tomorrow so I'll go back to posting once a day.
This snippet uses a transformation matrix to zoom in and pan a Sprite instance. For demo purposes I filled the sprite with a few circles - but you'd likely be using this with a vector image of a map, a floor plan or some other graphic that warrants zooming and panning.
Back around flash 7 (I think) before the Matrix class was introduced we used to have to use MovieClip nesting to achieve this effect.
Have a look at the swf...
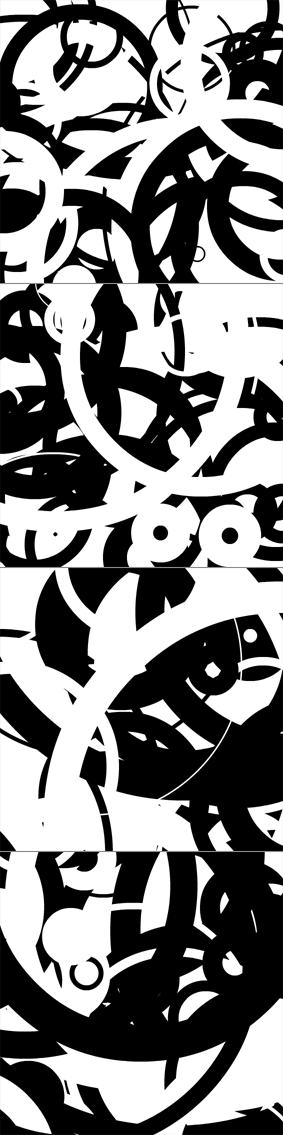