By Zevan | February 24, 2010
We’ve all done it… we’ve wasted way too much time writing an overly complex class just to create a simple square/roundrect button. Who has the nicest one? Post a link to yours (googlecode, wonderfl, etc…).
I’ve always avoided wasting too much time writing one of these but just spent 2 hours writing an overly complex one… looking forward to the way that people have done it….
For instance, did you make your class dynamic? Did you use scale9? did you use CSS? etc… Just curious how complex have gotten with it.
Also posted in UI | Tagged actionscript, as3, flash, UI |
By Zevan | November 4, 2009
Are you working on something right now that makes use of interfaces? Copy and paste an interface into the comments of this post (no need for code tags or anything)....
Actionscript:
-
package app{
-
-
import flash.events.IEventDispatcher;
-
-
public interface IBaseModel extends IEventDispatcher{
-
-
function set decimal(val:int):void;
-
-
function get decimal():int ;
-
-
function get stringValue():String;
-
-
function get errorMessage():String;
-
-
function set base(val:int):void;
-
-
function get base():int;
-
-
function startCounting(interval:Number, changeBy:Number):void;
-
-
function stopCounting():void;
-
}
-
}
Also posted in misc | Tagged actionscript, as3, flash |
Actionscript:
-
package{
-
-
import flash.display.*;
-
import flash.events.*;
-
import flash.geom.*;
-
-
public class QuickCheckBox extends Sprite{
-
-
private var _checked:Boolean;
-
private var _bitmap:Bitmap;
-
private var _canvas:BitmapData;
-
-
private static var checked:BitmapData;
-
private static var unchecked:BitmapData;
-
private static var bg:Shape;
-
private static var ex:Shape;
-
private static var pnt:Point;
-
-
// static init for BitmapData and drawing
-
{
-
trace("only render your graphics once");
-
pnt = new Point(0,0);
-
checked = new BitmapData(10,10, true, 0x00000000);
-
unchecked = new BitmapData(10, 10, true, 0x00000000);
-
bg = new Shape();
-
with(bg.graphics) lineStyle(0,0x000000), beginFill(0xEFEFEF), drawRect(0,0,9,9);
-
unchecked.draw(bg);
-
ex = new Shape();
-
with(ex.graphics) {
-
lineStyle(2,0x333333), moveTo(3, 3),
-
lineTo(7, 7), moveTo(7, 3), lineTo(3, 7);
-
}
-
checked.draw(bg);
-
checked.draw(ex);
-
}
-
-
public function QuickCheckBox(value:Boolean = false):void{
-
_checked = value;
-
_canvas = new BitmapData(10,10, true, 0x00000000);
-
_bitmap = new Bitmap(_canvas);
-
addChild(_bitmap);
-
buttonMode = true;
-
render();
-
addEventListener(MouseEvent.CLICK, onClick);
-
}
-
-
public function render():void{
-
if (_checked){
-
_canvas.copyPixels(QuickCheckBox.checked, _canvas.rect, pnt);
-
}else{
-
_canvas.copyPixels(unchecked, _canvas.rect, pnt);
-
}
-
}
-
-
private function onClick(evt:Event):void{
-
_checked = !_checked;
-
render();
-
this.dispatchEvent(new Event(Event.CHANGE, true));
-
}
-
-
public function get checked():Boolean{
-
return _checked;
-
}
-
-
public function set checked(val:Boolean):void{
-
_checked = val;
-
render();
-
}
-
}
-
}
This checkbox class uses a static initializer. Static initializers can come in handy to initialize some static variables for all class instances to make use of. In this case I'm using the static initializer to create checked and unchecked BitmapData objects - all instances of QuickCheckBox use these two static BitmapData objects rather than create their own unique ones.
Here is some client code if you want to test this class out:
Actionscript:
-
for (var i:int = 0; i<100; i++){
-
var checkBox:QuickCheckBox = new QuickCheckBox(true);
-
checkBox.x =100 + i % 10 * 12;
-
checkBox.y = 100 + int(i / 10) * 12;
-
// uncheck a few checkboxes
-
if (checkBox.x == checkBox.y){
-
checkBox.checked = false;
-
}
-
addChild( checkBox);
-
// checkBox dispatches a change event
-
checkBox.addEventListener(Event.CHANGE, onChange);
-
}
-
function onChange(evt:Event):void{
-
trace(evt.currentTarget.checked);
-
}
-
/*outputs
-
only render your graphics once
-
*/
This draws 100 checkboxes, - you'll also notice in the output window that the trace statement from QuickCheckBox only runs once.
Here are 100 instances of QuickCheckBox:
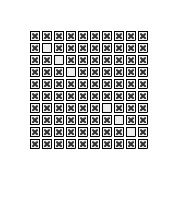
Also posted in BitmapData, UI | Tagged actionscript, flash |
Actionscript:
-
package {
-
-
import flash.display.Sprite;
-
import flash.utils.describeType;
-
-
public class Main extends Sprite {
-
-
public function Main(){
-
var test:Test = new Test();
-
var desc:XML= describeType(test);
-
// public vars
-
for each (var v:XML in desc.variable){
-
trace(v.@name, test[v.@name]);
-
}
-
// getters
-
for each (v in desc.accessor){
-
trace(v.@name, test[v.@name]);
-
}
-
}
-
-
}
-
}
-
-
class Test{
-
public var a:Number = 123;
-
public var b:Number = 100;
-
private var _getterVal:Boolean = false;
-
public function get getter():Boolean{
-
return _getterVal;
-
}
-
}
-
/*
-
outputs:
-
b 100
-
a 123
-
getter false
-
*/
I'm working on a few libraries, QuickBox2D and a library for auto-generated UI stuff... this technique just came in handy. It shows how to use describeType() to loop through public vars and getters of a given class.
The title of this post should really be Loop Through All PUBLIC properties of a class.... but it was long enough as it is....
Note: this should be run as document class
Also posted in Object, XML, dynamic | Tagged actionscript, flash |