By Zevan | December 31, 2009
Actionscript:
-
var one:Array = [1,2,3];
-
var two:Array = [10, 20, 30];
-
-
var zipOneTwo:Array = zip(one, two);
-
-
// trace each tupple
-
for each (var tuple:Array in zipOneTwo){
-
trace(tuple);
-
}
-
-
/* outputs:
-
1,10
-
2,20
-
3,30
-
*/
-
-
function zip(a:Array, b:Array):Array{
-
var longest:Array = (a.length>= b.length) ? a : b;
-
var zipped:Array = [];
-
for (var i:int = 0; i<longest.length; i++){
-
zipped.push([a[i], b[i]]);
-
}
-
return zipped;
-
}
This snippet shows a function called zip that takes two arrays and returns a two dimensional array of tuples. Just imagine that each array is one side of a zipper and you'll sort of get the idea...
I do wish flash would trace this:
[[1, 10], [2, 20], [3, 30]]
We shouldn't have to write a utility function to see the real array structure...
I've been messing with haskell for a few days now... just for fun I thought I'd write a few functions inspired by it... this is the first one...
Posted in arrays | Tagged actionscript, as3, flash |
Actionscript:
-
var a:Array = [true, true, true, false, false, true, true, true, false];
-
-
var counter:int = 0;
-
var prev:Boolean;
-
var summary:Array = [];
-
for (var i:int = 1; i<a.length; i++){
-
prev = a[i - 1]
-
counter++;
-
if (prev != a[i]){
-
if (prev){
-
summary.push("true: "+ counter);
-
}else{
-
summary.push("false: "+ counter);
-
}
-
counter = 0;
-
}
-
}
-
summary.push(a[i-1].toString()+": "+ (counter+1));
-
-
trace(summary);
-
-
/** outputs:
-
true: 3,false: 2,true: 3,false: 1
-
*/
This is a handy way to summarize the contents of an array of boolean values.
Also posted in misc | Tagged actionscript, as3, flash |
Actionscript:
-
[SWF(width=401,height=401,background=0xEFEFEF)]
-
-
var w:Number = stage.stageWidth-1;
-
var h:Number = stage.stageHeight-1;
-
var tileSize:Number = 20;
-
var halfTileSize:Number = 20;
-
var hTiles:Number = w / tileSize;
-
var vTiles:Number = h / tileSize;
-
var world:Shape = Shape(addChild(new Shape()));
-
var map:Array=[];
-
populateMap();
-
var gridColor:uint = 0xCCCCCC;
-
grid(tileSize, gridColor);
-
-
vTiles -= 1;
-
var movers:Array = [];
-
for (var i:int = 0; i<100; i++){
-
movers.push(makeMover(i % hTiles, int( i / hTiles),0x000000))
-
movers.push(makeMover(i % hTiles, vTiles - int( i / hTiles),0xFF0000))
-
}
-
var moverNum:int = movers.length;
-
hTiles -= 1;
-
-
addEventListener(Event.ENTER_FRAME, onLoop);
-
function onLoop(evt:Event):void {
-
world.graphics.clear();
-
for (var i:int = 0; i<moverNum; i++){
-
movers[i]();
-
}
-
}
-
function populateMap():void{
-
for (var i:int = 0; i<vTiles; i++){
-
map[i] = [];
-
for (var j:int = 0; j<hTiles; j++){
-
map[i][j] = 0;
-
}
-
}
-
}
-
function grid(size:Number=30, lineColor:uint=0xFFFF00, lineAlpha:Number=1):void {
-
with(graphics){
-
lineStyle(0, lineColor, lineAlpha);
-
drawRect(0,0,w,h);
-
for (var i:Number = size; i<w; i+=size) {
-
moveTo(i, 0);
-
lineTo(i, w);
-
}
-
for (i = size; i<h; i+=size) {
-
moveTo(0, i);
-
lineTo(h, i);
-
}
-
}
-
}
-
function makeMover(x:Number, y:Number, col:uint):Function{
-
var xp:Number = x;
-
var yp:Number = y;
-
var prevX:Number = x;
-
var prevY:Number = y;
-
map[yp][xp] = 1;
-
var dx:Number = xp;
-
var dy:Number = yp;
-
var counter:int = 0;
-
return function():void{
-
if (counter> 20){
-
if (int(Math.random()*30) == 1){
-
xp += int(Math.random()*2) - 1 | 1;
-
xp = xp <0 ? 0 : xp;
-
xp = xp> hTiles ? hTiles : xp;
-
if (map[yp][xp] == 1){
-
xp = prevX;
-
}else{
-
map[prevY][prevX] = 0;
-
map[yp][xp] = 1;
-
counter = 0;
-
}
-
prevX = xp;
-
}else
-
if (int(Math.random()*30) == 1){
-
yp += int(Math.random()*2) - 1 | 1;
-
yp = yp <0 ? 0 : yp;
-
yp = yp> vTiles ? vTiles : yp;
-
if (map[yp][xp] == 1){
-
yp = prevY;
-
}else{
-
map[prevY][prevX] = 0;
-
map[yp][xp] = 1;
-
counter = 0;
-
}
-
prevY = yp;
-
}
-
}
-
counter++;
-
dx += (xp - dx) * 0.5;
-
dy += (yp - dy) * 0.5;
-
with(world.graphics){
-
lineStyle(0, gridColor,1, true)
-
beginFill(col);
-
drawRect(dx * tileSize, dy * tileSize, tileSize, tileSize);
-
}
-
}
-
}
This (somewhat long) snippet moves boxes around on a grid - the boxes avoid one another by reading values in a 2D array. This technique can also be used for collision detection in tile-based games.
Have a look at the swf here...
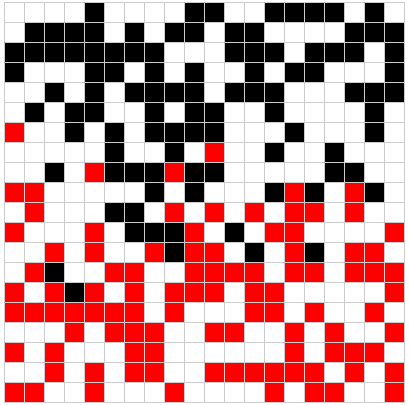
Also posted in motion, random | Tagged actionscript, as3, flash |
Actionscript:
-
var canvas:BitmapData=new BitmapData(400,400,false,0x000000);
-
addChild(new Bitmap(canvas));
-
var pix:Vector.<uint>=canvas.getVector(canvas.rect);
-
-
canvas.lock();
-
for (var i:int = 0; i<300; i++) {
-
var xp:int=50+i;
-
var yp:int=50+i/2;
-
// target x and y coords in 1D array
-
pix[xp+yp*400]=0xFFFFFF;
-
}
-
canvas.setVector(canvas.rect, pix);
-
canvas.unlock();
This snippet shows how to target x and y coordinates in a 1D Array / Vector. This can be useful sometimes when working with setVector().
This is sort of like re-inventing setPixel().... and for that reason is kind of pointless - that said, it's interesting to know. I first learned about this technique from using processing.